How to use PHP to submit form data and jump to the page
When writing web applications using PHP, we often need to submit form data and jump to another page. This article will introduce how to use PHP to submit form data and jump to the page.
First, we need to create an HTML form to collect user data. Here is a simple example:
<form action="process.php" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name"><br> <label for="email">Email:</label> <input type="email" id="email" name="email"><br> <input type="submit" value="Submit"> </form>
In the form, we specify an action called "process.php" and use the "post" method to submit the form data. When the user clicks the submit button, the form will send data to process.php.
Next, we need to create the process.php file to process the submitted form data. In this file, we can use the $_POST array to retrieve the submitted data. Here is a simple example:
<?php $name = $_POST['name']; $email = $_POST['email']; // do something with $name and $email header("Location: thank-you.php"); // redirect to thank-you page ?>
In the above example, we use the $_POST array to get the data named "name" and "email". We can then do whatever we want with this data. Finally, we use the header() function to redirect the user to another page called "thank-you.php". Note that this code should be called before any other output in the process.php file. Otherwise, the header() function will not work properly.
In the thank-you.php file we can display a thank you message or any other message to the user. The following is a simple example:
<!DOCTYPE html> <html> <head> <title>Thank You</title> </head> <body> <h1>Thank You</h1> <p>Thank you for submitting your information, <?php echo $_POST['name']; ?>!</p> </body> </html>
In the above example, we use the "name" data in the $_POST array to display the thank you message to the user. Please note that we use the syntax to insert user-entered data into the HTML.
In short, it is very simple to submit form data and jump to another page using PHP. We just need to use the $_POST array to retrieve the data and then use the header() function to redirect the user to another page. I wish you a pleasant experience!
The above is the detailed content of How to use PHP to submit form data and jump to the page. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
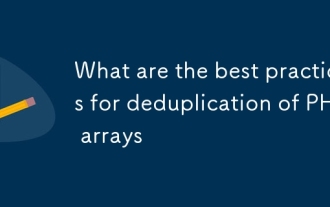
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
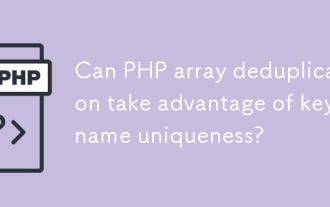
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
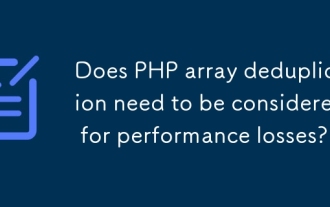
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
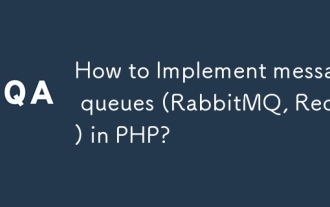
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
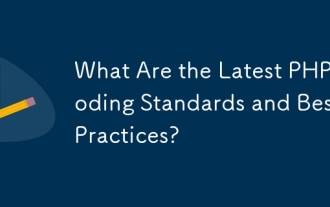
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
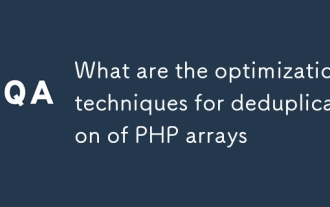
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
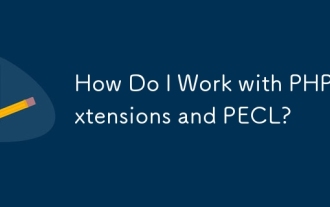
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
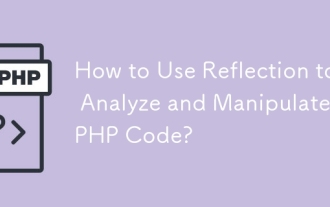
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
