


Summarize three methods of implementing Ajax cross-domain in PHP
With the popularity of front-end and back-end separation, it has become a common operation in web development for the front-end to call the back-end interface and obtain data through Ajax technology.
However, due to the browser's same-origin policy, web pages from different sources (different protocols, domain names or ports) cannot access each other's own DOM and cookies, which makes cross-domain access a very common requirement. There are many ways to solve this problem.
This article will introduce three ways to implement Ajax cross-domain in PHP.
1. JSONP
JSONP (JSON with Padding) is a very popular solution when the front end initiates cross-domain requests. It is actually a way to "cheat" the browser, taking advantage of the fact that the <script>
tag has no cross-domain restrictions to achieve cross-domain access. Its principle is to dynamically generate a JavaScript code snippet for responding to the request on the server side. This code snippet will call a JavaScript function with a specific name (callback function name) and pass the data processed by the server side as a parameter to this function. This achieves the effect of cross-domain access.
The usage of JSONP is as follows:
- Client code:
function handleJsonp(data) { console.log(data); } const script = document.createElement('script'); script.src = 'http://example.com/api?callback=handleJsonp'; document.head.appendChild(script);
- Server code:
<?php $data = array('foo' => 'bar'); $callback = $_GET['callback']; echo sprintf('%s(%s);', $callback, json_encode($data)); ?>
In this code, the return result from the server is a JavaScript code snippet containing a callback function call. After the client gets the data, it will automatically execute the callback function and use the data returned by the server as the parameter of the callback function.
The advantage of JSONP is good compatibility. The compatibility is only limited by the extent to which the browser supports the <script>
tag. However, the disadvantage is that there are security issues because the callback function is in the client. We cannot guarantee that this function executes the logic we expect. If the malicious callback function passes malicious code, there will be a risk of being attacked by XSS.
2. Proxy mode
The proxy mode is another popular cross-domain solution. Its basic idea is to set up a proxy on the server to access the specified URL, and then return the data obtained from the proxy server to the client. In this way, the client can directly access the proxy server from the same origin, and the proxy server then accesses the cross-domain target server and forwards the data returned by the target server.
The proxy mode is used as follows:
- Client code:
fetch('http://example.com/proxy_api') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
- Server code:
<?php $url = 'http://example.com/api'; $data = json_decode(file_get_contents($url), true); echo json_encode($data); ?>
In this code, the client's request is sent to the proxy server. The proxy server returns the data returned by the target server to the client, thus realizing the client's access to cross-domain services.
The advantage of proxy mode is good security, because the client will only access the proxy server from the same source, and the proxy server will then access the cross-domain target server, thus effectively avoiding the security caused by cross-domain access. Risky, but the disadvantage is that additional server-side code needs to be developed, which adds extra work and development costs.
3. CORS
CORS is currently the most popular cross-domain access solution. It sets the response header on the server side to tell the client whether to allow cross-domain access, thereby achieving cross-domain access security control.
CORS is used as follows:
- Client code:
fetch('http://example.com/api', { mode: 'cors' }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
- Server code:
<?php header('Access-Control-Allow-Origin: *'); $data = array('foo' => 'bar'); echo json_encode($data); ?>
In this code, the server sets the Access-Control-Allow-Origin:*
response header, indicating that all sources are allowed to access the interface. The client sets mode in the request: 'cors'
parameter to inform the browser that the request will be accessed across domains.
The advantage of CORS is that it supports natively and does not require additional development work. However, its disadvantage is that it does not support IE8/9 and needs to be supported from the server side. It does not support cross-domain access of subdomain names.
The above are three cross-domain methods for PHP to implement Ajax. In actual projects, you should choose the most suitable cross-domain solution according to the specific situation.
The above is the detailed content of Summarize three methods of implementing Ajax cross-domain in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
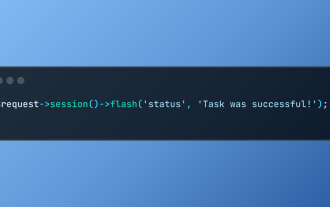
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
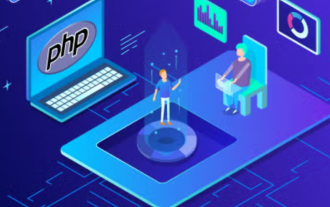
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
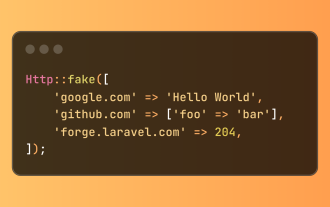
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
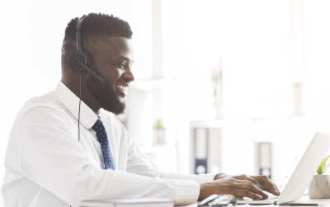
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
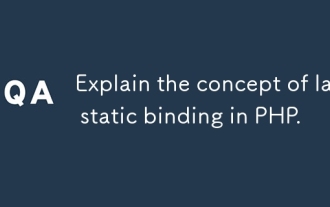
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
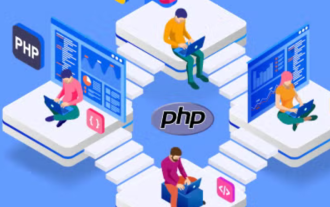
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
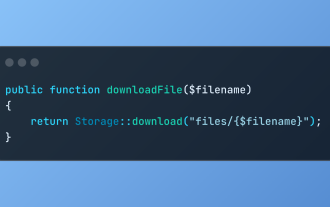
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
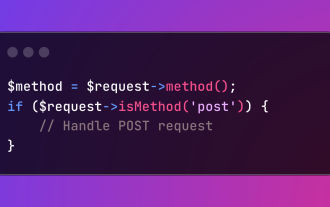
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building
