How to delete server video in php
During the website development process, we may need to delete some video files on the server for various reasons. Today we will discuss how to use PHP language to delete video files on the server.
First of all, we need to clarify a few concepts:
- Server file path
The server file path refers to the storage path of the video file on the server . In Linux systems, absolute paths are generally used, such as /var/www/html/video/1.mp4
; in Windows systems, relative paths can be used, such as .\video\1. mp4
.
- File deletion function unlink()
PHP provides us with a function unlink() for deleting files, its syntax is as follows:
bool unlink ( string $filename [, resource $context ] )
Among them, $filename represents the file path and file name string to be deleted; $context is an optional parameter, which can be a context stream of resource type returned by stream_context_create().
Next, let’s take a look at how PHP uses the unlink() function to delete a video file:
<?php $file = "/var/www/html/video/1.mp4"; //服务器上视频文件路径 if(unlink($file)) { //删除文件 echo "成功删除视频文件: ".$file; } else { echo "无法删除视频文件: ".$file; } ?>
The above PHP code first defines the file path to be deleted. Then, we use the unlink() function to delete the specified file. If the deletion is successful, "Video file successfully deleted: file path" is output on the page; if deletion fails, "Video file cannot be deleted: file path" is output.
It is worth noting that when the unlink() function accesses a file that does not exist, it will return false, so before deleting the file, we need to determine whether the file exists. We can use the file_exists() function that comes with PHP to determine whether a file exists, for example:
if (file_exists($file)) { // 如果文件存在,就执行删除操作 unlink($file); } else { // 如果文件不存在,则提示文件不存在。 echo "文件不存在,无法删除"; }
Finally, if we need to delete multiple video files, we can use a loop to achieve it. The following is a simple example to delete all video files in a specified directory:
<?php $dir = "/var/www/html/video/"; // 视频文件所在路径 if (is_dir($dir)){ if ($dh = opendir($dir)){ while (($file = readdir($dh)) !== false){ $file_path = $dir.$file; if (is_file($file_path) && stripos($file, '.mp4') !== false) { unlink($file_path); } } closedir($dh); } } ?>
In the above example, we first define the directory where the video files to be deleted are located. Then, we use PHP's built-in is_dir() function and opendir() function to verify whether the specified directory exists. If it exists, we use a while loop to traverse all files in the directory.
In each loop, we use the is_file() function to determine whether the current file is a video file (assuming here that the files we want to delete are all video files with an extension of .mp4), if so, we Call the unlink() function to delete the file.
Finally, we close the directory handle through the closedir() function.
In short, it is very convenient to use PHP to delete video files on the server. We only need to know the path of the file to be deleted, and the deletion operation can be easily achieved. At the same time, to ensure that the files we deleted were not accidentally deleted, we can use the file_exists() function to make judgments.
The above is the detailed content of How to delete server video in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
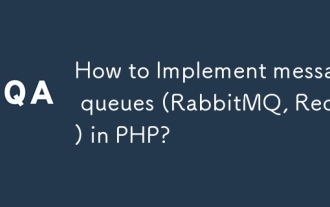
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
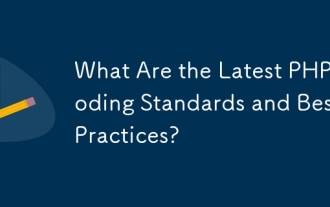
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
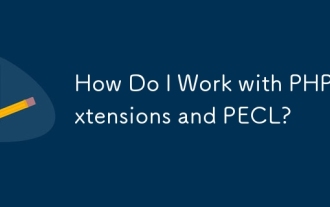
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
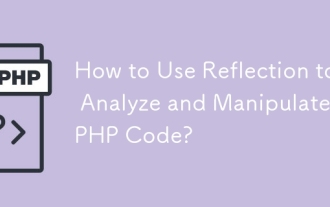
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
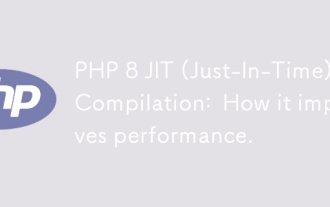
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
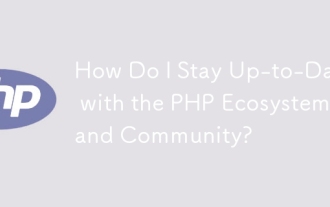
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
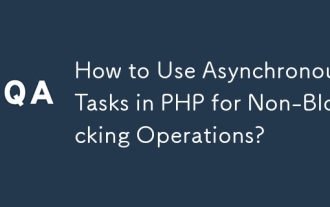
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
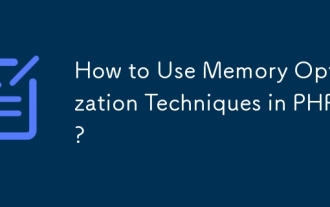
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
