Let's talk about how to use PHP to replace and generate images
In website development, we often encounter situations where images need to be processed, such as making thumbnails, generating watermarks, etc. The realization of these functions is inseparable from the operations of replacing and generating pictures. This article will discuss how to replace and generate images using PHP.
1. Image replacement
In website development, it is often necessary to replace images. For example, a user uploads a wrong picture, and the administrator needs to replace it or replace an outdated picture with a new one, etc. At this time, we can use PHP's image processing library to implement image replacement.
1. Preparation
First, we need to install the PHP image processing library. PHP supports a variety of image processing libraries, the most common of which are the GD library and the Imagick library. This article takes the GD library as an example.
Under Linux system, you can use the following command to install the GD library:
1 |
|
If you are using Windows system, you can add the comment characters before the following two lines in the php.ini file Remove:
1 2 |
|
This will enable the GD library.
2. Replace the image code
The PHP code to replace the image is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
|
In the code, $src and $dst are the paths of the source and target files. The getimagesize() function is used to obtain image information and obtain the source image handle according to the image type. Use the imagecreatetruecolor() function to create the target image handle, use the imagecopy() function to copy the source image to the target image, and finally call the corresponding image saving function according to the source image type to save the generated target image. Finally, destroy the image handle to avoid memory leaks.
2. Picture generation
In addition to replacing pictures, we often need to generate various pictures. For example, make thumbnails, generate verification codes, etc. At this time, we can use PHP's image processing library to generate images.
1. Preparation
Before generating an image, we need to determine the size, color and other attributes of the required image, and then create an image handle accordingly. In this article, we take creating a black and white plaid background image as an example.
The code to create the image handle is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
In the code, $width and $height are the width and height of the image. Use the imagecreatetruecolor() function to create a canvas, and then use the imagecolorallocate() function to define the black and white colors. Use a loop to draw a rectangle, determine the color of the current rectangle based on the values of $i and $j, and use the imagefilledrectangle() function to draw the rectangle. Finally, use the header() function to tell the browser that the output is an image, and use the imagepng() function to output the generated image.
2. Other image generation
In addition to creating grid background images, PHP's image processing library can also create thumbnails, generate verification codes, etc. For example, the following code can be used to generate a 4-digit verification code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
In the code, $code is a randomly generated verification code. Use the imagecreate() function to create a 60×24 graphics drawing area. And use the imagecolorallocate() function to set the background color of the area to white. Use the imagestring() function to write the randomly generated verification code in this area, and finally use the header() function to tell the browser that the output is an image, and use the imagepng() function to output the generated PNG format image.
Summary
This article introduces the method of using PHP's image processing library to replace and generate images. Image processing is a problem that often needs to be dealt with in website development. Using PHP's image processing library can easily replace and generate images, bringing convenience and efficiency to website development.
The above is the detailed content of Let's talk about how to use PHP to replace and generate images. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
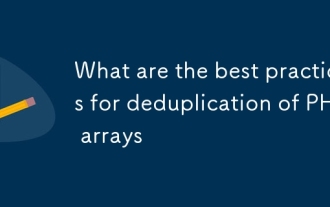
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
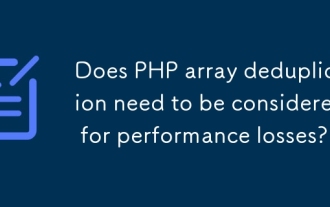
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
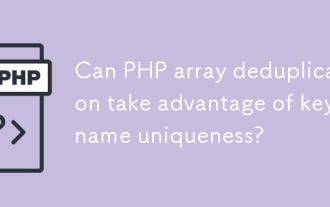
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
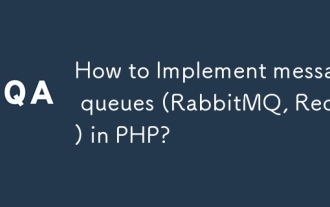
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
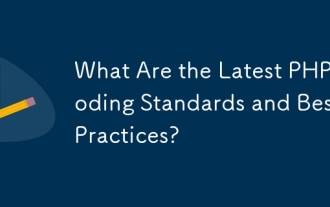
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
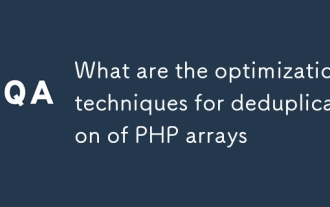
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
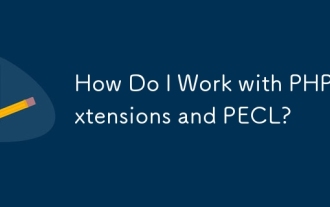
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
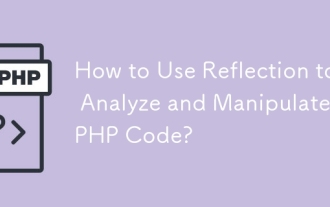
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
