How to use golang to implement plug-ins
In modern programming languages, plug-ins are an important concept. Through the plug-in mechanism, the program can achieve more flexible and efficient expansion and function expansion. If the program supports the plug-in mechanism, users can use plug-ins to add specific functions as needed without worrying about breaking the core code. The plug-in mechanism is also supported in the golang language. The following will introduce how to use golang to implement plug-ins.
- Define the interface
Since plug-ins are usually external modules that are independent of the main program, an interface must be defined to ensure that the plug-in can cooperate well with the main program. When defining the interface, you need to clarify the functions and interface methods required by the plug-in. For example, in this example, we define a simple interface PluginInterface:
type PluginInterface interface { Run() Info() string }
This interface defines two methods: Run() and Info(). These two methods will be what plugin developers must implement to ensure that the plugin and main program can communicate with each other.
- Implementing the plugin
After this, the developer can start implementing the plugin. Plugins can be any structure that conforms to the requirements of the interface. For example, the following is a simple implementation:
type PluginExample struct { Info string } func (p *PluginExample) Run() { fmt.Println(“Plugin Example is running…”) } func (p *PluginExample) Info() string { return p.Info }
In this implementation, we define a structure named PluginExample, which contains an Info field of type string. The Run() and Info() methods are both methods in the PluginInterface interface and must be implemented by the plug-in.
- Loading the plug-in
Once the plug-in implementation is completed, you can start loading the plug-in. In golang language, plug-ins are implemented by using the plugin package. Here is a simple example:
package main import ( "fmt" "plugin" ) func main() { p, err := plugin.Open("./plugin_example.so") if err != nil { panic(err) } sym, err := p.Lookup("PluginExample") if err != nil { panic(err) } plugin, ok := sym.(PluginInterface) if !ok { panic("invalid plugin") } plugin.Run() fmt.Println(plugin.Info()) }
In this example, we use plugin.Open() to open the compiled plugin file plugin_example.so. Next, find the plug-in symbol PluginExample through the p.Lookup() method. Once the symbol is found, we can cast it to the PluginInterface interface and use that interface to call the plugin's methods.
- Compile plug-in
In the golang language, in order to create a plug-in, we need to use the go build command to compile instead of the go run command. We also need to specify a specific compilation flag -buildmode=plugin to compile and create a plug-in. For example, the following command will compile a plugin file named plugin_example:
go build -buildmode=plugin -o plugin_example.so plugin_example.go
In this command, we use the -buildmode=plugin flag to tell the Go compiler to create a plugin, and then name the plugin plugin_example.so.
Plug-in is an important concept in modern programming, which can achieve better program flexibility and scalability. In the golang language, by using the plugin package, we can easily implement plug-in functions and provide additional thinking space for our programs.
The above is the detailed content of How to use golang to implement plug-ins. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


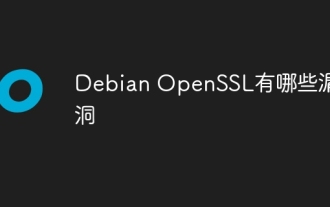
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
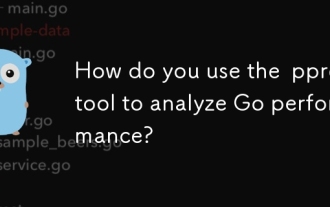
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
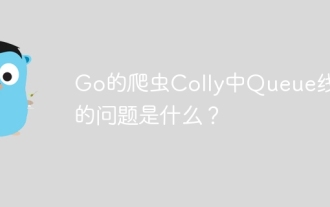
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
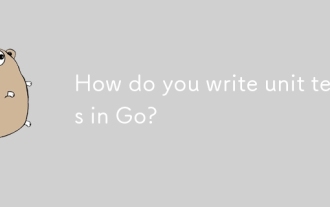
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
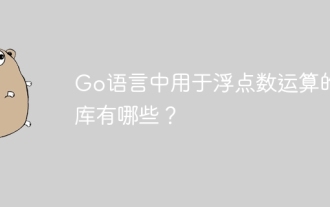
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
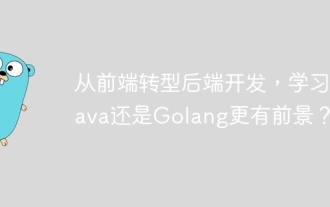
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
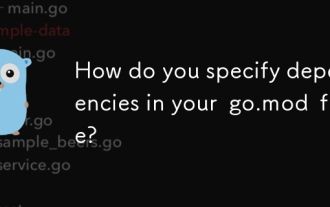
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
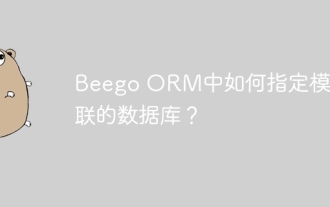
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
