Detailed introduction to the usage of golang cond
Golang is an efficient, concise, and concurrent programming language. In Golang, cond is a tool used to control the concurrent execution of programs. This article will introduce the usage of cond in detail.
1. Basic introduction to cond
The full name of cond is condition variable, which is an important concurrency control mechanism in Golang. The main function of cond is to allow multiple goroutines to wait for the state of a shared variable to change at the same time, and to notify the waiting goroutine to perform subsequent operations when the state of the shared variable changes. Unlike mutex, cond cannot be used to protect concurrent access to shared variables, it needs to rely on mutex to complete. Its basic usage is very simple and is divided into three steps:
- When goroutine needs to wait for changes in the status of shared variables, call the cond.Wait() function to enter the waiting state;
- Other goroutines When the state of a shared variable changes, call the cond.Signal() or cond.Broadcast() function to wake up the waiting goroutine;
- After waking up the waiting goroutine, you need to reacquire the mutex lock of the shared variable. Continue execution.
2. Usage of cond
In practical applications, cond is usually used in combination with mutex to achieve concurrent safe access to shared variables. The following is a simple sample program that demonstrates how to use cond to control the concurrent execution of multiple goroutines:
package main import ( "fmt" "sync" ) var ( count int mutex sync.Mutex cond *sync.Cond = sync.NewCond(&mutex) ) func worker() { for { mutex.Lock() for count < 10 { cond.Wait() } count-- fmt.Printf("worker: %d\n", count) mutex.Unlock() cond.Signal() } } func main() { for i := 0; i < 10; i++ { go worker() } for { mutex.Lock() if count >= 10 { mutex.Unlock() break } count++ fmt.Printf("main: %d\n", count) mutex.Unlock() cond.Signal() } }
In this sample code, count represents a shared variable with an initial value of 0, indicating the number of tasks that can be executed. . When the value of the shared variable count is less than 10, all worker goroutines will wait until the value of the shared variable count is increased to more than 10 before being awakened.
In the main goroutine, increase the value of the shared variable count by calling the cond.Signal() function in a loop, and notify the waiting worker goroutines to continue execution. When the value of the shared variable count is increased to 10, the main goroutine stops calling the cond.Signal() function, and all worker goroutines exit execution.
It is worth noting that when goroutine waits for cond, it will automatically release the mutex lock so that other goroutines can access shared variables. Once awakened, the goroutine needs to reacquire the mutex lock to continue execution.
3. Precautions for cond
Although using cond is an efficient synchronization mechanism compared to the traditional condition variable mechanism, there are also matters that need to be paid attention to when using it:
- The cond.Wait() function should always be used in a for loop to avoid false wake-ups. Specifically, the judgment of waiting conditions should be added when waking up;
- When using Before cond, mutex should be initialized;
- After using cond.Signal() or cond.Broadcast(), the awakened goroutine needs to reacquire the mutex lock;
- cond also cannot guarantee thread safety , so when multiple goroutines access shared variables, mutex needs to be used for concurrency security control.
4. Conclusion
Golang’s concurrency control mechanism is very powerful. Through the two basic concurrency control mechanisms of mutex and cond, powerful concurrent programs can be realized. When using cond, you need to pay attention to its basic usage methods and precautions, and understand the operating principle of the cond mechanism in order to better play its role.
The above is the detailed content of Detailed introduction to the usage of golang cond. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


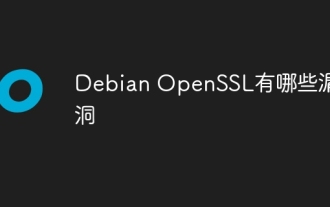
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
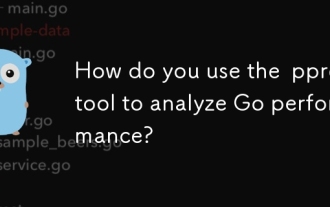
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
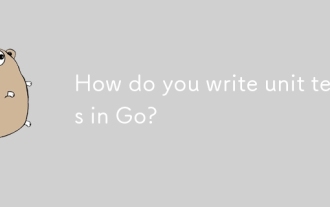
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
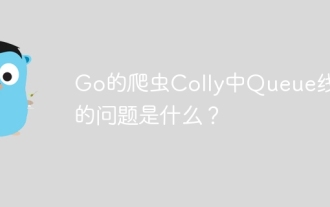
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
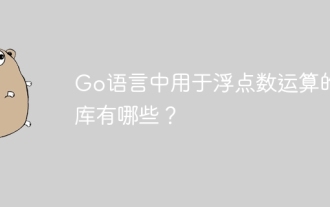
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
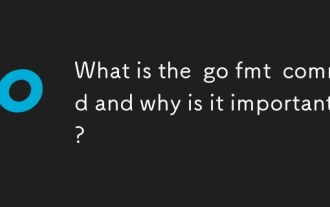
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
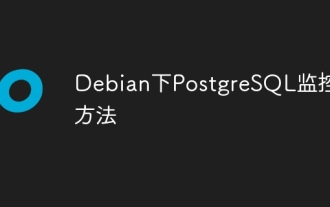
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
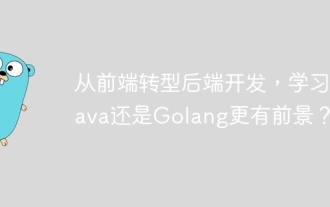
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
