


Summarize the commonly used add, delete, modify and query functions in PHP
PHP is a widely used open source server-side scripting language. Its powerful database support makes it a key tool for web application development. In PHP, many functions are provided for addition, deletion, modification and query. These functions can help us operate the database more conveniently. This article will introduce some commonly used add, delete, modify and query functions in PHP.
1. Add data
1.mysql_query()
mysql_query() is one of the most commonly used functions in PHP, used to add data to the database. The SQL statement it executes must be an INSERT statement, which is used to insert a record into a table.
The following is a sample code for adding data to the database using the mysql_query() function:
//连接到MySQL数据库 $conn = mysql_connect($host, $user, $password); mysql_select_db($database, $conn); //定义要添加的数据 $name = "Peter"; $age = 25; //执行INSERT语句 $sql = "INSERT INTO persons (name, age) VALUES ('$name', '$age')"; $result = mysql_query($sql); if (!$result) { echo "添加数据失败:" . mysql_error(); }
2.PDO::exec()
PDO::exec() function also Data can be added to the database. The difference between it and the mysql_query() function is that the PDO::exec() function returns the number of affected rows, while the mysql_query() function returns resource type data. When executing an INSERT statement, the PDO::exec() function returns the number of inserted records.
The following is a sample code for adding data to the database using the PDO::exec() function:
//连接到MySQL数据库 $conn = new PDO("mysql:host=$host;dbname=$database", $user, $password); //定义要添加的数据 $name = "Peter"; $age = 25; //执行INSERT语句 $sql = "INSERT INTO persons (name, age) VALUES ('$name', '$age')"; $affectedRows = $conn->exec($sql); if ($affectedRows == 0) { echo "添加数据失败"; }
2. Delete data
1.mysql_query()
When using the mysql_query() function to delete data, the SQL statement executed must be a DELETE statement. The following is a sample code for using the mysql_query() function to delete data:
//连接到MySQL数据库 $conn = mysql_connect($host, $user, $password); mysql_select_db($database, $conn); //执行DELETE语句 $sql = "DELETE FROM persons WHERE name = 'Peter'"; $result = mysql_query($sql); if (!$result) { echo "删除数据失败:" . mysql_error(); }
2.PDO::exec()
The main difference between the PDO::exec() function and the mysql_query() function is It returns the number of rows affected. When executing the DELETE statement, the PDO::exec() function returns the number of deleted records.
The following is a sample code for using the PDO::exec() function to delete data:
//连接到MySQL数据库 $conn = new PDO("mysql:host=$host;dbname=$database", $user, $password); //执行DELETE语句 $sql = "DELETE FROM persons WHERE name = 'Peter'"; $affectedRows = $conn->exec($sql); if ($affectedRows == 0) { echo "删除数据失败"; }
3. Modify the data
1.mysql_query()
When using the mysql_query() function to modify data, the SQL statement executed must be an UPDATE statement. The following is a sample code for using the mysql_query() function to modify data:
//连接到MySQL数据库 $conn = mysql_connect($host, $user, $password); mysql_select_db($database, $conn); //执行UPDATE语句 $sql = "UPDATE persons SET age = 26 WHERE name = 'Peter'"; $result = mysql_query($sql); if (!$result) { echo "修改数据失败:" . mysql_error(); }
2.PDO::exec()
PDO::exec() function can also be used to modify data, executed The SQL statement must also be an UPDATE statement. The following is an example code for using the PDO::exec() function to modify data:
//连接到MySQL数据库 $conn = new PDO("mysql:host=$host;dbname=$database", $user, $password); //执行UPDATE语句 $sql = "UPDATE persons SET age = 26 WHERE name = 'Peter'"; $affectedRows = $conn->exec($sql); if ($affectedRows == 0) { echo "修改数据失败"; }
4. Query data
1.mysql_query()
Use the mysql_query() function to query When extracting data, the SQL statement executed must be a SELECT statement. The following is a sample code for querying data using the mysql_query() function:
//连接到MySQL数据库 $conn = mysql_connect($host, $user, $password); mysql_select_db($database, $conn); //执行SELECT语句 $sql = "SELECT * FROM persons WHERE age = 26"; $result = mysql_query($sql); $numRows = mysql_num_rows($result); if ($numRows > 0) { while ($row = mysql_fetch_assoc($result)) { //处理查询结果 } } else { echo "没有找到数据"; }
2.PDO::query()
The PDO::query() function is used to execute a SELECT statement and return a PDOStatement object. The following is a sample code for querying data using the PDO::query() function:
//连接到MySQL数据库 $conn = new PDO("mysql:host=$host;dbname=$database", $user, $password); //执行SELECT语句 $sql = "SELECT * FROM persons WHERE age = 26"; $result = $conn->query($sql); $numRows = $result->rowCount(); if ($numRows > 0) { while ($row = $result->fetch(PDO::FETCH_ASSOC)) { //处理查询结果 } } else { echo "没有找到数据"; }
Summary: PHP provides many functions for adding, deleting, modifying, and querying. This article introduces some commonly used functions, including mysql_query(), PDO::exec(), PDO::query(), etc. Readers can choose the function that suits them according to their needs in actual development.
The above is the detailed content of Summarize the commonly used add, delete, modify and query functions in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


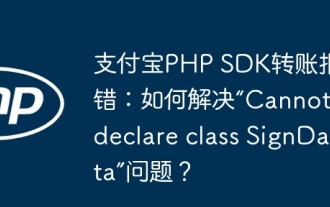
Alipay PHP...
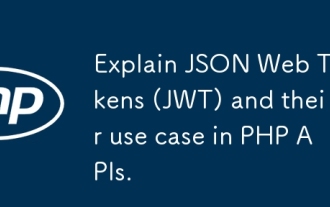
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
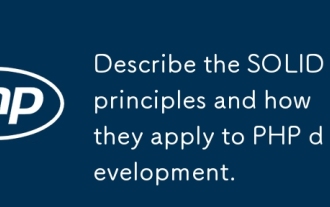
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
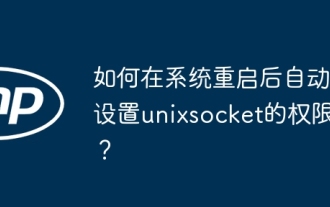
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
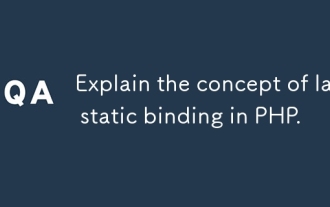
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
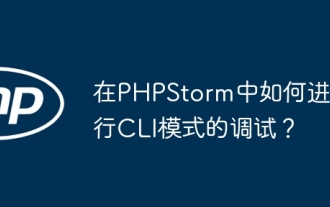
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
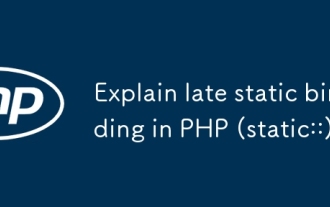
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
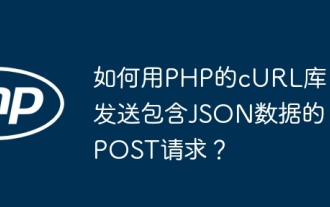
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
