Let's talk about how to delete data records and files in PHP
PHP is a widely used server-side scripting language that plays an important role in web development. When doing web development, it is often necessary to delete certain data records and files. PHP provides a series of functions and methods to implement these operations. This article will introduce how to delete data records and files in PHP.
1. Delete data records
When doing web development, it is often necessary to delete some data records in the database. PHP provides two keywords, DELETE FROM
and WHERE
and operators =
and <>
, for deleting data from the database delete data records.
- Use the DELETE FROM keyword to implement deletion operations
Using theDELETE FROM
keyword to delete data records can directly complete all related work in the SQL statement. Therefore, using this keyword can not only simplify the code, but also improve the efficiency of code execution. The following is a sample code for deleting data records using theDELETE FROM
keyword:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // 建立数据库连接 $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查数据库连接 if (!$conn) { die("连接失败: " . mysqli_connect_error()); } // SQL 删除语句 $sql = "DELETE FROM 表名 WHERE 列名=具体记录值"; // 执行 SQL 删除语句 if (mysqli_query($conn, $sql)) { echo "删除成功!"; } else { echo "删除失败:" . mysqli_error($conn); } // 关闭数据库连接 mysqli_close($conn); ?>
- Use the WHERE keyword to implement the delete operation
UseWHERE
Keyword deletion of data records requires building more complex SQL statements.DELETE
syntax example usingWHERE
keyword:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // 建立数据库连接 $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查数据库连接 if (!$conn) { die("连接失败: " . mysqli_connect_error()); } // SQL 删除语句 $sql = "DELETE FROM 表名 WHERE 列名=具体记录值"; // 执行 SQL 删除语句 if (mysqli_query($conn, $sql)) { echo "删除成功!"; } else { echo "删除失败:" . mysqli_error($conn); } // 关闭数据库连接 mysqli_close($conn); ?>
2. Delete files
When doing web development, you may encounter Need to delete certain files. PHP provides a series of functions and classes to implement these operations.
- Use the unlink() function to delete files
PHP can delete files very conveniently through theunlink()
function. The following is a sample code for using theunlink()
function to delete files:
$file = "path/to/file"; if (unlink($file)) { echo "文件删除成功!"; } else { echo "文件删除失败!"; }
- Use the unlink() function to delete a directory and all files and subdirectories under it
Code example of using theunlink()
function to recursively delete a directory and all files and subdirectories under it:
function deleteAll($dir) { $handler = opendir($dir); while (($file = readdir($handler)) !== FALSE) { if ($file != '.' && $file != '..') { $path = $dir . DIRECTORY_SEPARATOR . $file; if (is_dir($path)) { deleteAll($path); } else { if (unlink($path)) { echo '删除文件:' . $path . '<br />'; } else { echo '删除文件失败:' . $path . '<br />'; } } } } closedir($handler); if (rmdir($dir)) { echo '删除目录:' . $dir . '<br />'; } else { echo '删除目录失败:' . $dir . '<br />'; } }
Special attention needs to be paid when using this method because it is recursive operation, so if you want to perform this method, please make a backup of the relevant files first. At the same time, because this method will delete a directory and all files and subdirectories under it, it needs to be used with caution. If you want to delete a specified directory and specific files or file types under it, you need to write code according to specific needs.
To sum up, this article introduces how to delete data records and files in PHP. Through the introduction of this article, I believe readers can easily master these operations and successfully apply related technologies in their own Web applications.
The above is the detailed content of Let's talk about how to delete data records and files in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
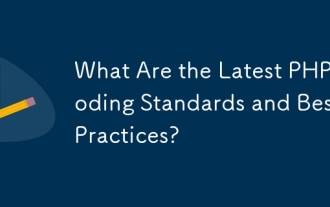
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
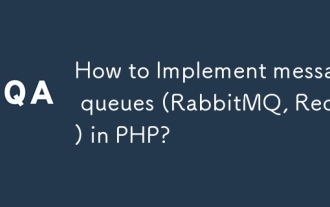
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
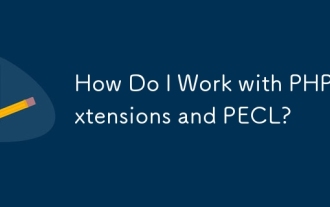
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
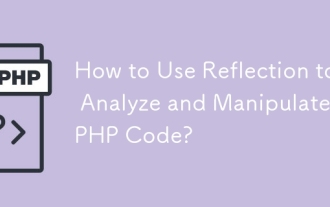
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
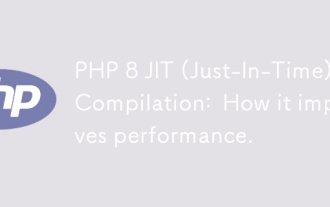
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
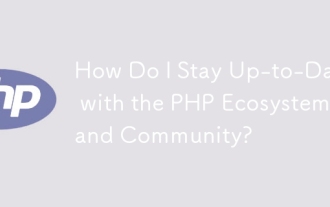
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
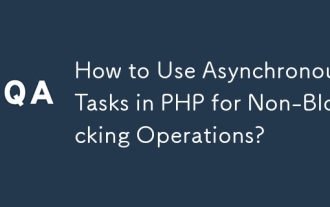
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
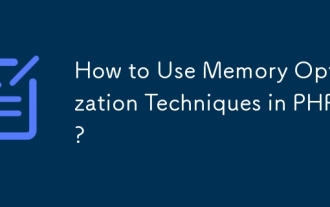
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
