Discuss the usage of Sleep function in Golang
Go language (Golang) is an open source programming language developed by Google. Go has natural support for concurrent programming and provides some built-in functions and tools to facilitate developers to perform concurrent programming. Among them, the Sleep function is provided in the time package. This article will explore the usage of the Sleep function in Golang.
1. Sleep function overview
The Sleep function is used to put the current coroutine (goroutine) into sleep state and suspend execution for a period of time. The function prototype is as follows:
func Sleep(d Duration)
Among them, Duration is a type representing a time period. The minimum time unit supported is nanoseconds (ns), and the maximum time is approximately 290 years. In the program, you can use the constants provided in the time package to represent different time periods, such as:
const ( Nanosecond Duration = 1 Microsecond = 1000 * Nanosecond Millisecond = 1000 * Microsecond Second = 1000 * Millisecond Minute = 60 * Second Hour = 60 * Minute )
2. Sleep function usage examples
Below we demonstrate the Sleep function through several examples usage.
- Basic usage
package main import ( "fmt" "time" ) func main() { fmt.Println("start") time.Sleep(1 * time.Second) fmt.Println("end") }
After executing the program, the output is as follows:
start (end 1秒后输出) end
After the program starts, "end" is output after sleeping for 1 second.
- Precise time control
When multiple goroutines are executed concurrently, in order to ensure the correctness of the program, it is necessary to ensure the execution of each goroutine The time is as precise as possible. The following example demonstrates how to use the Sleep function to precisely control time.
package main import ( "fmt" "time" ) func main() { for i := 0; i < 3; i++ { go func() { fmt.Println("start") time.Sleep(1 * time.Second) fmt.Println("end") }() } time.Sleep(2 * time.Second) }
After executing the program, the output is as follows:
start start start (end 1秒后输出) end end end
In the above code, we used a for loop to create 3 coroutines for concurrent execution, and the execution time of each coroutine is 1 second. bell. The sleep time is 2 seconds, ensuring that each coroutine can be executed and output as expected.
- Prevent repeated operations in a short time
In the actual programming process, sometimes it is necessary to prevent repeated operations in a short time. For example, after clicking a button, you need to wait for a while before clicking again. The following example demonstrates how to use the Sleep function to implement this functionality.
package main import ( "fmt" "time" ) func main() { click() time.Sleep(2 * time.Second) click() } func click() { now := time.Now() fmt.Println(now.Format("2006-01-02 15:04:05")) time.Sleep(1 * time.Second) }
After executing the program, the output is as follows:
(start time) 2022-06-15 20:35:43 (end time) 2022-06-15 20:35:44 (start time) 2022-06-15 20:35:46 (end time) 2022-06-15 20:35:47
In the above code, we define a click function, which is used to record the current time and sleep for 1 second. In the main function, we first call the click function once, and then wait 2 seconds to call it again. Since we waited 2 seconds before the second call, the second call will be executed 1 second after the first call.
3. Conclusion
This article introduces the usage and examples of Sleep function in Golang.
Using the Sleep function can effectively control the execution time of concurrent programs and ensure the correctness and stability of the program.
Special attention should be paid to the fact that when using the Sleep function, try to avoid using a long sleep time. Too long sleep time will block coroutine execution and affect program performance.
The above is the detailed content of Discuss the usage of Sleep function in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


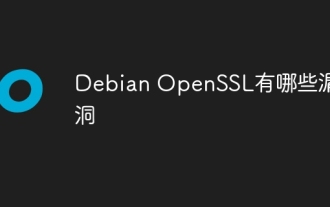
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
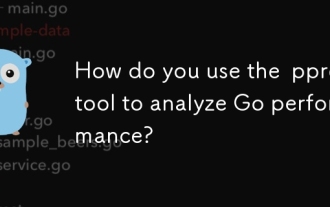
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
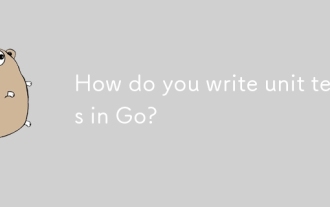
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
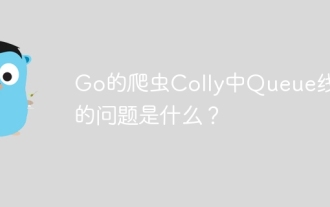
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
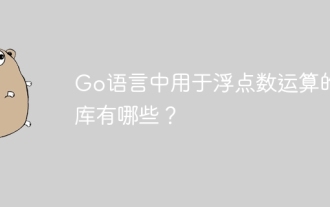
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
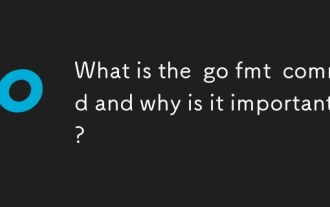
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
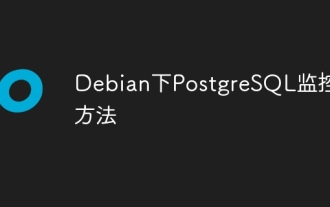
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
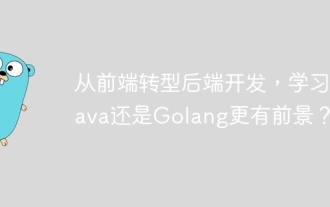
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
