How is PHP escaping implemented?
Escape refers to converting special characters into a form that can be recognized by the machine in the program. In PHP, such escaping also exists. PHP escaping is accomplished by adding a backslash "\" before the character. For example, to escape double quotes ("), you can write:
echo "She said \"Hello\"";
This will output on the screen: She said "Hello".
In PHP, there are many needs Escaped characters. Here are some common characters that need to be escaped and their escape characters:
Characters that need to be escaped | Escape Characters |
---|---|
single quotes | \' |
double quotes | \ " |
Backslash | \ |
Line break | \n |
Carriage return character | \r |
Horizontal tab character | \t |
Failure to escape will result in syntax errors or program errors.
When using the database, escaping is also required. If not escaped, users may insert malicious code into the database, causing the system to be attacked. PHP provides us with two functions for escaping: mysqli_real_escape_string() and addslashes().
The mysqli_real_escape_string() function is the MySQL escape function provided by PHP. It has good compatibility and supports multiple character sets. The addslashes() function is a built-in function of PHP. The escape characters are fixed and only support strings with the character set ISO-8859-1.
The following is an example of using the mysqli_real_escape_string() function:
$mysqli = new mysqli("localhost", "username", "password", "database"); if ($mysqli->connect_errno) { echo "Failed to connect to MySQL: " . $mysqli->connect_error; exit(); } $name = mysqli_real_escape_string($mysqli, $_POST['name']); $email = mysqli_real_escape_string($mysqli, $_POST['email']); $message = mysqli_real_escape_string($mysqli, $_POST['message']); $query = "INSERT INTO messages (name, email, message) VALUES ('$name', '$email', '$message')"; $result = $mysqli->query($query); if ($result === TRUE) { echo "Message sent successfully"; } else { echo "Error: " . $mysqli->error; } $mysqli->close();
In the above example, we use the mysqli_real_escape_string() function to escape the name, email and message entered by the user to avoid SQL injection attacks.
In addition to MySQL, other databases also need to be escaped. Different databases have different escape methods, and you need to choose the appropriate escape function according to the specific situation.
To summarize, escaping is an important part of writing safe PHP programs and must be used with caution. Escapes are required when outputting characters or inserting data into the database. It is recommended to use the mysqli_real_escape_string() function for escaping to avoid missing escape characters.
The above is the detailed content of How is PHP escaping implemented?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
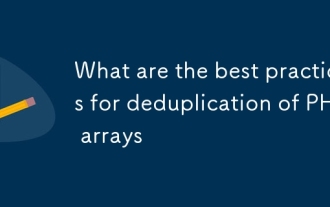
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
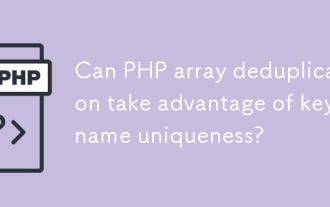
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
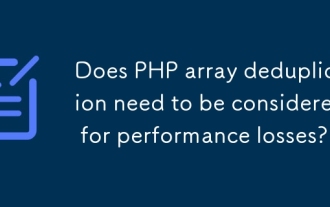
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
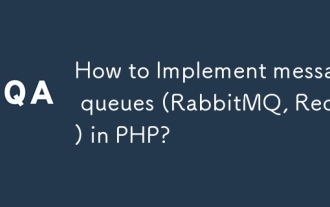
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
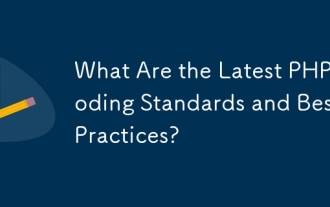
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
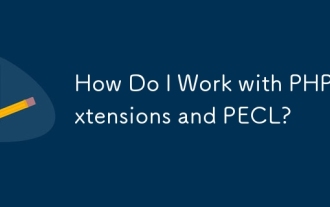
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
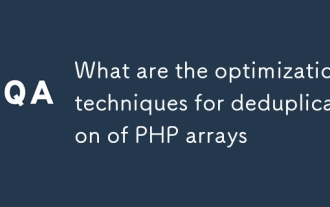
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
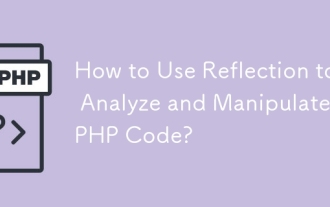
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
