How to close the connection in golang http
As Golang becomes more and more popular, its HTTP library becomes more and more popular. However, sometimes we need to close the connection during an HTTP request. What should we do at this time?
First, let us take a look at the life cycle of the HTTP connection. When a client sends an HTTP request to the server, it creates a TCP connection. After the server receives this request, it will return an HTTP response. At the end of the HTTP response, it sends a "Connection: close" header. This tells the client that the connection has been closed and that it should close the connection after reading the response. This process is called "short connection".
If the client does not read the entire response, it will remain connected and continue reading the response. This is called a "long connection". In this case, the client needs to explicitly close the connection after reading the response.
So how to close the connection in Golang?
First, we can use http.Client to close the connection. This can be achieved by setting a timeout in the request. For short connections, we can set the timeout to 0 seconds. This will cause the client to close the connection immediately after reading the response.
For example, the following code can be used to close the connection:
import ( "net/http" "time" ) func main() { client := http.Client{ Timeout: time.Second * 0, } req, err := http.NewRequest("GET", "http://example.com", nil) if err != nil { // handle error } resp, err := client.Do(req) if err != nil { // handle error } defer resp.Body.Close() // read response }
If you want a long connection, you need to set its value to a very large value when setting the timeout. For example, the following code can be used to maintain the connection state:
import ( "net/http" "time" ) func main() { client := http.Client{ Timeout: time.Second * 600, } req, err := http.NewRequest("GET", "http://example.com", nil) if err != nil { // handle error } resp, err := client.Do(req) if err != nil { // handle error } defer resp.Body.Close() // read response }
Another way to close the connection is to use context.Context. This approach allows context information to be shared across multiple HTTP requests and can be used to cancel or timeout requests.
The following is a sample code for closing a connection using context.Context:
import ( "context" "net/http" "time" ) func main() { ctx, cancel := context.WithTimeout(context.Background(), time.Second) defer cancel() req, err := http.NewRequestWithContext(ctx, "GET", "http://example.com", nil) if err != nil { // handle error } client := http.Client{} resp, err := client.Do(req) if err != nil { // handle error } defer resp.Body.Close() // read response }
In the above code, we use the WithTimeout method to create a context with a timeout of one second. Next, we use the NewRequestWithContext method to assign a context to the request. We then use http.Client to perform the request and close the response body at the end.
Summary
In this article, we introduced how to close HTTP connections in Golang. We discussed the life cycle of HTTP connections and how to use http.Client and context.Context to achieve connection closure. Whether it is a short connection or a long connection, we can achieve real HTTP connection closing in Golang.
The above is the detailed content of How to close the connection in golang http. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


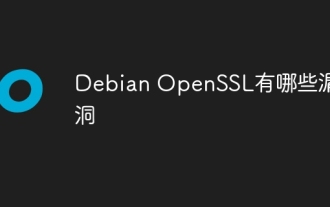
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
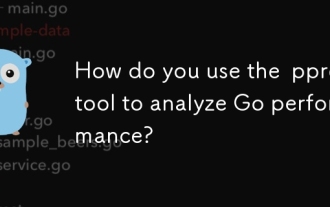
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
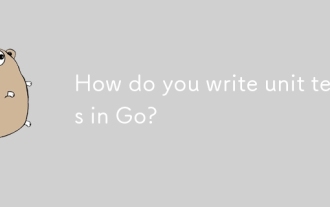
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
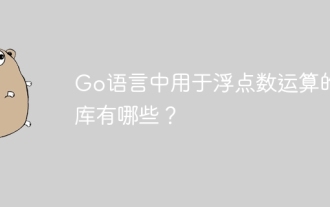
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
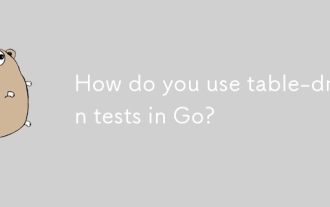
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
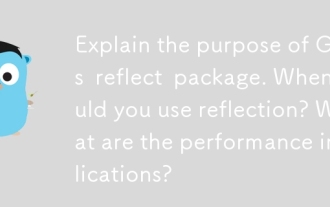
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
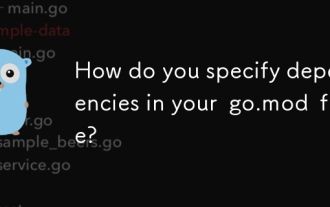
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
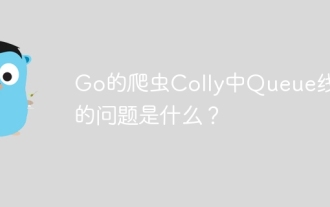
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
