


How to implement addition, subtraction, multiplication and division calculations in php
PHP is one of the common high-level programming languages used for web development. PHP has many advantages compared to other programming languages, one of which is that it is easy to learn. In this article, we will use PHP to implement a calculator for the four arithmetic operations of addition, subtraction, multiplication, and division.
1. Environment preparation
Before writing code, we need to ensure that the PHP running environment has been installed on the computer. If you haven't installed it yet, you can go to the official website to download the latest version of the PHP installer, and then follow the prompts to install it.
2. Development Ideas
Before implementing the calculator, we need to plan the development idea of the program:
- First, we need to create a A form containing two numeric input boxes and a select box to determine the operator type.
- Next, we need to obtain the value submitted by the form through PHP and perform corresponding calculation operations.
- Finally, we need to output the calculation results to the page and present them on the page.
3. HTML form
First, we need to create a form in HTML, the code is as follows:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>计算器</title> </head> <body> <h1>计算器</h1> <form method="post" action="calculator.php"> <label for="num1">第一个数字:</label> <input type="number" id="num1" name="num1" required> <br> <label for="num2">第二个数字:</label> <input type="number" id="num2" name="num2" required> <br> <label for="operator">选择运算符:</label> <select id="operator" name="operator"> <option value="+">加</option> <option value="-">减</option> <option value="*">乘</option> <option value="/">除</option> </select> <br> <input type="submit" value="计算"> </form> </body> </html>
In the above code, we create a form through HTML form. The form is submitted to the calculator.php page through the POST method, which contains two numeric input boxes and a selection box, and the user selects the calculation operation that needs to be performed.
4. PHP Calculator Implementation
Next, we need to go to the PHP file calculator.php to complete the specific implementation of the calculator.
Before starting, first get the value submitted by the form:
$num1 = $_POST['num1']; $num2 = $_POST['num2']; $operator = $_POST['operator'];
Then, we need to perform the corresponding calculation operation:
switch($operator) { case '+': $result = $num1 + $num2; break; case '-': $result = $num1 - $num2; break; case '*': $result = $num1 * $num2; break; case '/': $result = $num1 / $num2; break; }
In the above code, we use the switch statement To perform corresponding calculation operations based on the value of the selection box, calculate the results of addition, subtraction, multiplication, and division respectively, and the results are saved in the variable $result.
Finally, we need to output the calculation results to the page:
echo "<h1>计算结果</h1>"; echo "<p>{$num1} {$operator} {$num2} = {$result}</p>";
The above code uses the echo keyword to output the calculation results to the page, and the user can clearly see the calculation results.
5. Complete code
The following is the complete calculator.php code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>计算器</title> </head> <body> <h1>计算器</h1> <form method="post" action="calculator.php"> <label for="num1">第一个数字:</label> <input type="number" id="num1" name="num1" required> <br> <label for="num2">第二个数字:</label> <input type="number" id="num2" name="num2" required> <br> <label for="operator">选择运算符:</label> <select id="operator" name="operator"> <option value="+">加</option> <option value="-">减</option> <option value="*">乘</option> <option value="/">除</option> </select> <br> <input type="submit" value="计算"> </form> <?php if ($_POST) { $num1 = $_POST['num1']; $num2 = $_POST['num2']; $operator = $_POST['operator']; switch($operator) { case '+': $result = $num1 + $num2; break; case '-': $result = $num1 - $num2; break; case '*': $result = $num1 * $num2; break; case '/': $result = $num1 / $num2; break; } echo "<h1>计算结果</h1>"; echo "<p>{$num1} {$operator} {$num2} = {$result}</p>"; } ?> </body> </html>
6. Summary
Through this article, we learned how A calculator that uses PHP language to implement the four arithmetic operations of addition, subtraction, multiplication and division. During the implementation, we first created an HTML form where the user could enter numbers and select operators. Next, we use PHP to obtain the value submitted by the form and perform corresponding calculation operations. Finally, we output the calculation results to the page so that users can clearly see it.
The above is the detailed content of How to implement addition, subtraction, multiplication and division calculations in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


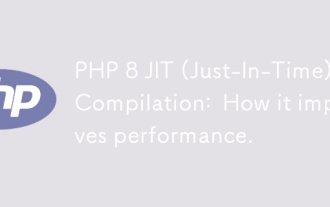
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
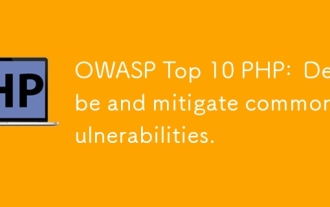
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
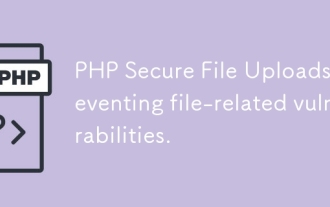
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
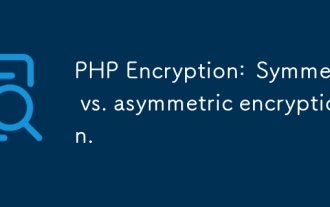
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
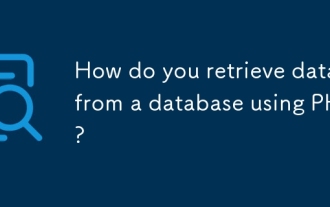
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
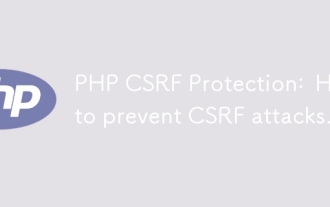
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.
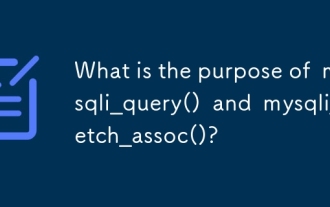
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
