


How to query domain name information from whois server using PHP
[Foreword]
In the world of the Internet, a domain name is the portal through which a website enters. It is the link address we enter when visiting a website. It is also an important item that we need to purchase and configure when creating a website.
For website administrators and network security engineers, it is also necessary to understand the relevant information of the domain name, such as: domain name owner, registration information, DNS, etc.
Today we will introduce how to use PHP to query domain name information from the whois server.
[Preparation]
Before conducting the query, we need to first understand the whois service.
Whois refers to a query protocol that provides domain name information query services on the Internet. We can query the information of a domain name through whois, including the registrant, registration time, expiration time, DNS server information, etc.
At the same time, we also need to prepare some basic knowledge: HTTP protocol and PHP network requests.
The HTTP protocol is a communication rule used to transmit hypertext between a web browser and a web server, while PHP's network requests are completed through curl or fsockopen.
Next, we use PHP code to implement the function of querying domain name information.
[PHP code for querying domain name information]
First, we need to create a form to allow users to enter the domain name they need to query in the input box, as shown below:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>查询域名信息</title> </head> <body> <form method="post" action=""> <fieldset> <legend>请输入要查询的域名:</legend> <input type="text" name="domain" value="" /> <input type="submit" name="submit" value="查询" /> </fieldset> </form> </body> </html>
Next, we need to write PHP code to perform the query function.
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { // 获取用户输入的域名 $domain = $_POST['domain']; // 查询域名信息 $whois = get_whois($domain); // 输出查询结果 echo "<pre class="brush:php;toolbar:false">"; echo $whois; echo ""; } // 获取域名信息 function get_whois($domain) { // 指定whois服务器地址 $whois_server = "whois.domain.com"; // 连接whois服务器 $fp = fsockopen($whois_server, 43); // 查询域名信息 fputs($fp, $domain . "\r\n"); // 获取查询结果 $whois = ""; while (!feof($fp)) { $whois .= fgets($fp, 128); } fclose($fp); // 返回查询结果 return $whois; } ?>
First, we determine whether the user has submitted a query request by checking the HTTP request method.
Next, we obtain the domain name entered by the user through $_POST and pass it to the get_whois function to obtain the whois information of the domain name.
In the get_whois function, we first specify the whois server address to query.
Next, connect to the whois server through the fsockopen function, use the fputs function to send a query request to the server, and obtain the query results through a while loop.
Finally, we return the query results and output the query results on the page.
[Summary]
Querying domain name information with PHP is very simple. You only need to use the fsockopen function to connect to the whois server and send a query request to the server.
Whether you are a website manager or a network security engineer, understanding domain name information is very helpful. Through the whois query protocol, we can obtain the registration information, owner, expiration time, DNS server information, etc. of the domain name.
The above is the detailed content of How to query domain name information from whois server using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


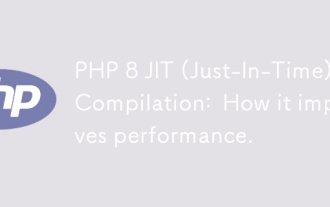
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
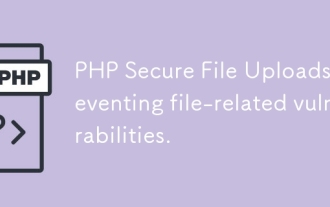
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
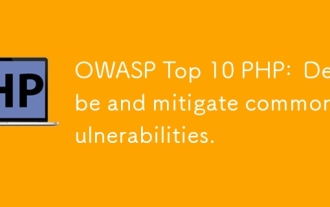
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
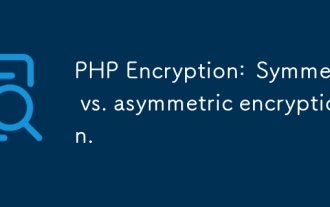
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
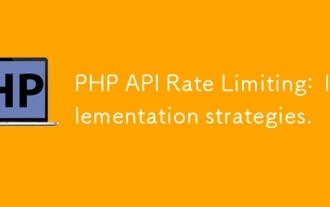
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
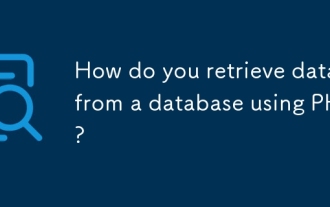
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
