Let's take a look at the usage of golang gjson
In the field of modern software development, data processing and data parsing are tasks that developers often encounter. With the emergence and continuous development of new data formats, how to efficiently parse and process these data has become a challenge. In this regard, golang gjson is a very useful tool that can assist us in parsing JSON data easily.
Introduction to gjson
gjson is a go language library for parsing JSON data. It implements the function of quickly parsing JSON data through chained APIs. gjson is concise and easy to use because it only focuses on parts of the JSON data rather than the entire document. In addition, gjson also supports JSON path query. It can provide great convenience when parsing complex JSON data.
Simple usage example
Let us see how to use gjson in golang program. We first need to import the gjson library:
import "github.com/tidwall/gjson"
Then, we can quickly parse the JSON data in the following way:
package main import ( "fmt" "github.com/tidwall/gjson" ) func main(){ data := `{"name": "golang", "version": "1.15.8"}` value := gjson.Get(data, "version") fmt.Printf("version: %s\n", value.String()) }
In the above code, we retrieve it by using the gjson.Get() function The value named "version" in the JSON data. We can convert the type of the value to a string for output on the console.
Complex usage example
Let us look at a more complex example of parsing complex JSON data through gjson. Consider the JSON file as follows:
{ "name": "Jack", "age": 30, "hobbies": [ { "name": "reading", "level": 2 }, { "name": "music", "level": 3 }, { "name": "swimming", "level": 1 } ], "contact": { "email": "jack@example.com", "phone": { "home": "123456", "mobile": "78901234" } } }
We can use gjson to access various parts of the JSON data, such as getting the first array element of the hobbies part, the code is as follows:
package main import ( "fmt" "github.com/tidwall/gjson" ) func main() { data := `{"name": "Jack", "age": 30, "hobbies": [{"name": "reading", "level": 2}, {"name": "music", "level": 3}, {"name": "swimming", "level": 1}], "contact": {"email": "jack@example.com", "phone": {"home": "123456", "mobile": "78901234"}}}` hobbies := gjson.Get(data, "hobbies") firstHobby := gjson.Get(hobbies.String(), "#0") fmt.Printf("first hobby: %s\n", firstHobby.String()) }
In the above code, #0
represents the first element of the array, #1
represents the second element, and so on. We can also select all array elements by wildcard character *
. For example, you can get all interest levels using the following code:
package main import ( "fmt" "github.com/tidwall/gjson" ) func main() { data := `{"name": "Jack", "age": 30, "hobbies": [{"name": "reading", "level": 2}, {"name": "music", "level": 3}, {"name": "swimming", "level": 1}], "contact": {"email": "jack@example.com", "phone": {"home": "123456", "mobile": "78901234"}}}` levels := gjson.Get(data, "hobbies.*.level") fmt.Printf("hobby levels: %s\n", levels.String()) }
In the above example, we have used .
to indicate a deep traversal instead of just traversing the immediate child elements.
gjson can easily read nested JSON data or irregularly structured data, and its API is very intuitive and easy to read. If you want more information and examples, please visit [gjson official website](https://github.com/tidwall/gjson).
Summary
This article introduces the main usage of golang gjson, including simple use cases and complex use cases. Using gjson can improve our code writing efficiency when processing JSON data. gjson is one of the simplest and easiest-to-use JSON parsing libraries in the golang community. One of the great things about gjson is that its API is relatively simple and can be used with other libraries to build applications. Because gjson can provide efficient JSON parsing performance, it is easier to maintain excellent performance in big data parsing scenarios.
The above is the detailed content of Let's take a look at the usage of golang gjson. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
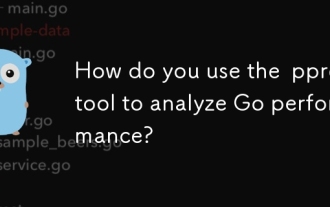
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
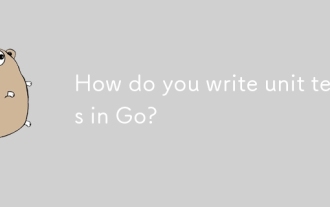
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
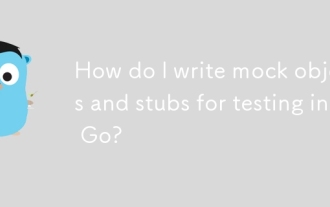
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
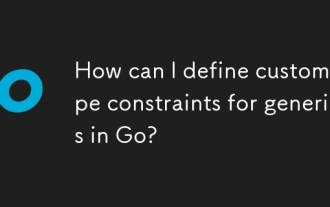
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
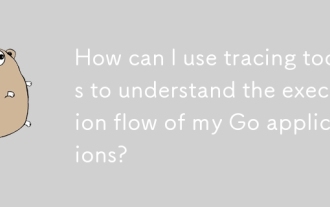
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
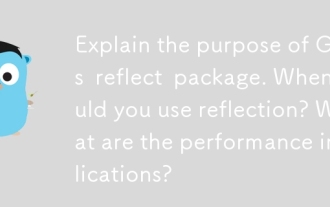
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
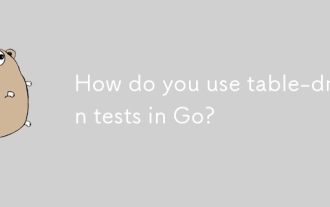
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
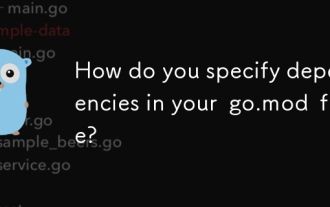
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
