How to use goroutine to implement parallel requests in go language
Golang is a very popular programming language. It has many unique features, one of the important features is that it supports parallel processing. In today's world, network requests have become part of our daily lives. If we need to request multiple APIs, how can we complete this task in the shortest possible time? This is exactly what the topic of golang parallel requests is about.
The so-called parallel request simply means initiating multiple network requests at the same time to improve the efficiency of the request. In golang, we can use goroutine to implement parallel requests to quickly process a large number of requests.
First, let’s take a look at how to use goroutine to implement parallel requests.
package main import ( "fmt" "net/http" ) func main() { urls := []string{ "http://example.com/1", "http://example.com/2", "http://example.com/3", } for _, url := range urls { go func(url string) { res, err := http.Get(url) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Response:", res.Status) }(url) } fmt.Scanln() }
In this example, we define a URLs array, which contains the URLs we need to request. We iterate over this array and start a new goroutine on each URL using the go
keyword. This goroutine sends an HTTP GET request and outputs the response to the terminal after the request is completed.
Such simultaneous requests will make our program faster, but there will also be some problems. First, if we start too many goroutines, we may exceed the maximum concurrency allowed by the operating system. Secondly, we need to wait for all requests to complete before we can get the results, which may cause the response time to be too long, which means we need some more efficient processing methods.
Next, let’s take a look at how to use goroutine and channel to achieve more efficient parallel requests.
package main import ( "fmt" "net/http" ) func main() { urls := []string{ "http://example.com/1", "http://example.com/2", "http://example.com/3", } ch := make(chan string) for _, url := range urls { go func(url string) { res, err := http.Get(url) if err != nil { fmt.Println("Error:", err) return } ch <- fmt.Sprintf("Response from %s: %s", url, res.Status) }(url) } for i := 0; i < len(urls); i++ { fmt.Println(<-ch) } }
In this example, we define a channel named ch
, and we send the results to the channel in each goroutine. In the main thread, we use a loop to receive all the results in the channel and print them to the terminal.
The advantage of using channels is that we can control the number of goroutines, and we do not need to wait for all goroutines to complete the request to get the result. Compared with waiting for all requests to complete, such parallel requests can allow us to get results faster, and also avoid lags caused by long response times for requests.
In summary, golang parallel request is a method that uses the characteristics of the golang language to process multiple network requests at the same time, thereby improving request efficiency. In implementing parallel requests, we can use goroutine and channel to control the concurrency of the program and return the results immediately after receiving the response of each request, allowing us to process a large number of requests in the shortest time.
The above is the detailed content of How to use goroutine to implement parallel requests in go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


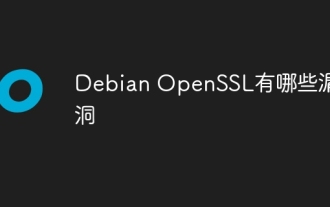
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
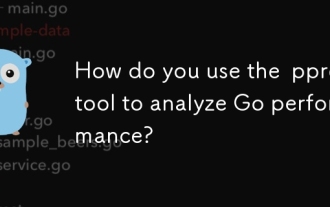
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
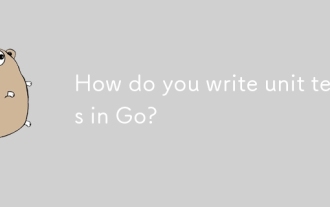
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
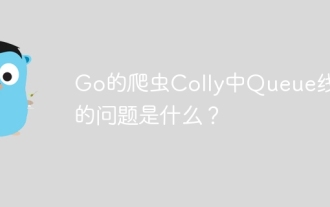
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
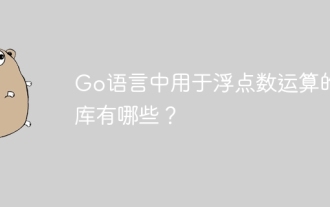
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
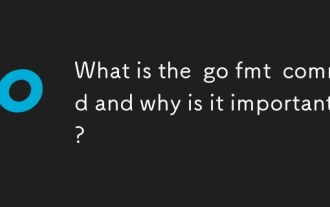
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
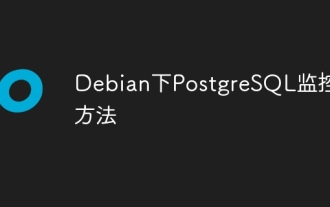
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
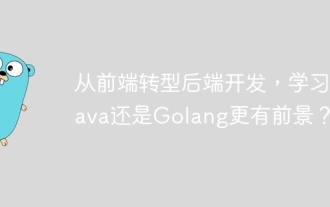
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
