How to create a simple PHP project using the HBuilder editor
PHP is a very popular programming language used to develop many web applications. In this article, we will learn how to use the HBuilder editor to create a simple PHP project, including adding, deleting, and modifying operations.
In order to start writing PHP code, you need to install PHP and the HBuilder editor. You can download PHP from the official website and HBuilder editor from here and install them on your computer.
Step One: Create HTML File
First, we will create an HTML file that will serve as the home page of our web application. Create a file named "index.html" under HBuilder, which should include the following HTML code:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>简单 PHP 项目:增删改 HBuilder</title> </head> <body> <h1>欢迎来到我的简单 PHP 项目</h1> <p>这个项目包括增删改功能。</p> </body> </html>
This HTML file will serve as the home page of our web application and contain basic information about the project.
Step 2: Create a database
Next, we need to create a MySQL database instance to store our data. We will use the phpMyAdmin tool to create and manage our database.
Using the tool, create a database named "php_project" and create a table named "users" with the following fields:
- id: for unique Identify each user;
- name: the user’s name;
- email: the user’s email address;
- phone: the user’s phone number;
Step 3: Create a PHP file
Now, we will create a PHP file to connect to the database and perform operations such as select, insert, update, and delete. Create a file called "index.php" in the HBuilder editor and use the following code:
<?php $host = "localhost"; $user = "root"; $password = ""; $database = "php_project"; $conn = mysqli_connect($host, $user, $password, $database); if(!$conn){ die("<h3>连接数据库失败:" . mysqli_connect_error() . "</h3>"); } echo "<h3>成功连接到数据库!</h3>"; ?>
This code will connect to the database and display a message, if the connection is successful, it will display "Successful connection To database!" message.
Next, let’s write the code to select, insert, update, and delete users:
<?php $host = "localhost"; $user = "root"; $password = ""; $database = "php_project"; $conn = mysqli_connect($host, $user, $password, $database); if(!$conn){ die("<h3>连接数据库失败:" . mysqli_connect_error() . "</h3>"); } // 选择用户 $select_query = "SELECT * FROM users"; $result = mysqli_query($conn, $select_query); if(mysqli_num_rows($result) > 0){ while($row = mysqli_fetch_assoc($result)){ echo "<p>ID:" . $row['id'] . "<br>"; echo "名称:" . $row['name'] . "<br>"; echo "邮箱:" . $row['email'] . "<br>"; echo "电话:" . $row['phone'] . "<br></p>"; } }else{ echo "<p>没有找到用户。</p>"; } // 插入新用户 $name = "张三"; $email = "zhangsan@example.com"; $phone = "13111111111"; $insert_query = "INSERT INTO users (name, email, phone) VALUES ('$name', '$email', '$phone')"; if(mysqli_query($conn, $insert_query)){ echo "<p>新用户添加成功!</p>"; }else{ echo "<p>添加用户时出错:" . mysqli_error($conn) . "</p>"; } // 更新用户信息 $id = 1; $new_phone = "13222222222"; $update_query = "UPDATE users SET phone='$new_phone' WHERE id=$id"; if(mysqli_query($conn, $update_query)){ echo "<p>用户信息更新成功!</p>"; }else{ echo "<p>更新用户信息时出错:" . mysqli_error($conn) . "</p>"; } // 删除用户信息 $id = 2; $delete_query = "DELETE FROM users WHERE id=$id"; if(mysqli_query($conn, $delete_query)){ echo "<p>用户信息删除成功!</p>"; }else{ echo "<p>删除用户信息时出错:" . mysqli_error($conn) . "</p>"; } mysqli_close($conn); ?>
This code will select, insert, update, and delete user information and output the results so that we can View in browser.
Step 4: Test your application
Now, you have created an HTML file, a database, and a PHP file for database connections and data operations. Let's test your application by visiting "http://localhost/PATH_TO_PROJECT/index.html" and see the output.
If your output matches the code above, you have successfully created a simple PHP project, including additions, deletions, and modifications.
Now you can use the HBuilder editor to optimize your code and continue to improve your web application to better match your needs and goals.
The above is the detailed content of How to create a simple PHP project using the HBuilder editor. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


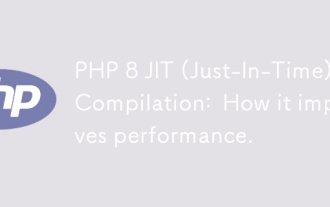
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
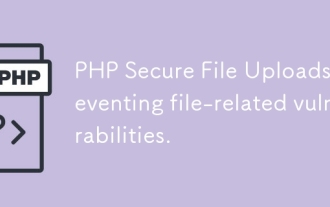
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
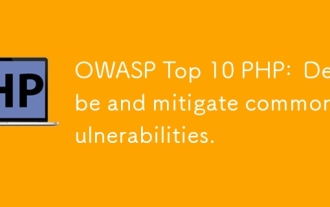
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
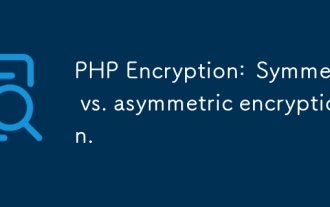
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
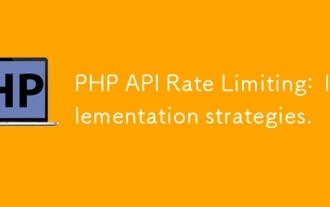
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
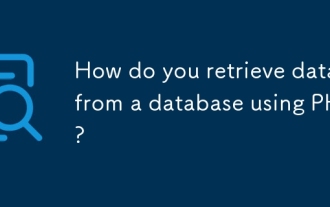
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
