How to enable 'disable batch assignment' feature in laravel
In the Laravel framework, non-batch assignment is an important security feature, which helps prevent malicious users from tampering with database data. However, this feature sometimes has unclear uses, causing confusion among many programmers.
During batch assignment, the programmer saves the form data directly into the database through the create or update method. If no verification is performed, there will be great risks due to serious threats such as hacker attacks and injections. To solve this problem, Laravel introduced a feature that disables batch assignment.
The non-batch assignment means that when using the create or update method, if the fields allowed to be saved are not specified, the program will automatically filter out all illegal fields. This feature not only improves the security of the program, but also strengthens the development constraints on programmers: only explicitly allowed fields can be saved to the database.
This feature can be enabled with a very simple line of code. Use the $guarded attribute in models that need to prohibit batch assignment.
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { protected $guarded = []; }
In the example, the $guarded property is an empty array, which means that all fields are editable.
If you want to allow only specific fields to be saved, you can set the $guarded attribute to an array containing all fields that are not allowed to be edited, or use the $fillable attribute.
<?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { protected $fillable = ['name', 'email', 'password']; }
A better approach is to perform data validation in the controller and then save it to the database after validation. This can avoid some misoperations and safety issues.
<?php namespace App\Http\Controllers; use App\User; use Illuminate\Http\Request; class UserController extends Controller { public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string', 'email' => 'required|email|unique:users', 'password' => 'required|confirmed', ]); $user = User::create($validatedData); return back()->with('success', 'User created successfully.'); } }
All input fields are verified here through the validate method. If the verification is successful, it is saved to the database. The above code is not only highly secure, but also very elegant.
In general, Laravel's non-batch assignment is a perfect mechanism that can effectively improve the security of the program. We should take advantage of this feature as much as possible to avoid unnecessary risks in development.
The above is the detailed content of How to enable 'disable batch assignment' feature in laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


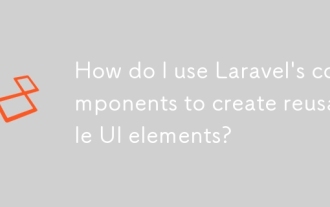
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
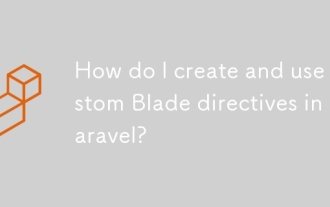
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
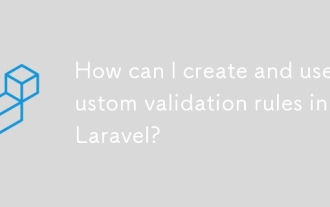
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
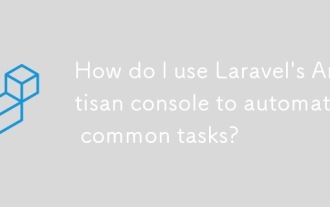
Laravel's Artisan console automates tasks like generating code, running migrations, and scheduling. Key commands include make:controller, migrate, and db:seed. Custom commands can be created for specific needs, enhancing workflow efficiency.Character
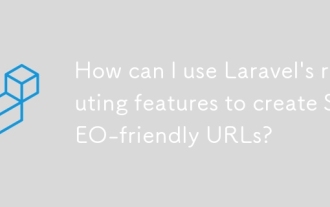
The article discusses using Laravel's routing to create SEO-friendly URLs, covering best practices, canonical URLs, and tools for SEO optimization.Word count: 159

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
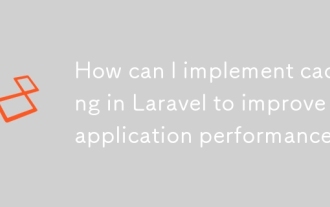
The article discusses implementing caching in Laravel to boost performance, covering configuration, using the Cache facade, cache tags, and atomic operations. It also outlines best practices for cache configuration and suggests types of data to cache
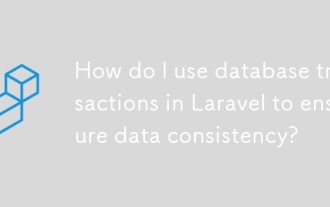
The article discusses using database transactions in Laravel to maintain data consistency, detailing methods with DB facade and Eloquent models, best practices, exception handling, and tools for monitoring and debugging transactions.
