Detailed explanation of how to stop Golang program
Golang is an open source programming language widely used in enterprise application development. During Golang program development, sometimes you need to manually stop the running program. So, how to stop the Golang program?
- Use Ctrl C
When running a Golang program on the command line, you can use the Ctrl C key combination to stop the program. When the program is running, pressing Ctrl C will trigger an interrupt signal, thus ending the running of the program. This is a simple yet effective way to stop a Golang program.
- By setting channel
In Golang, you can use channel to stop the program. The specific steps are as follows:
- Define a channel variable to receive the stop signal
stop := make(chan bool)
- Use a for loop in the program to wait for the stop signal
for { // 等待停止信号 select { case <-stop: return default: // 执行程序 } }
- When you need to stop the program, send a stop signal to the channel
go func() { stop <- true }()
When the stop signal is received, the program will exit the loop and end its operation.
- Using the context package
Golang's context package provides an elegant way to stop the program. When using the context package, you need to first create a context object and call the WithCancel method to return a cancel function and cancellation signal. Then, use this context object in the program, and once the cancellation signal is received, the program will stop running gracefully. The specific steps are as follows:
- Create a context object
ctx, cancel := context.WithCancel(context.Background())
- Use the context object in the program
for { select { case <-ctx.Done(): return default: // 执行程序 } }
- When you need to stop the program, call the cancel function
go func() { cancel() }()
. The above are three ways to stop the Golang program. Whether you use Ctrl C, set a channel, or use the context package, they are all very practical methods. When writing a Golang program, you can choose the most appropriate method to stop the program according to actual needs.
The above is the detailed content of Detailed explanation of how to stop Golang program. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
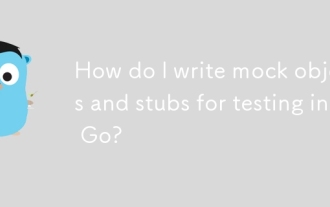
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
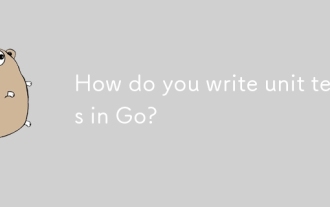
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
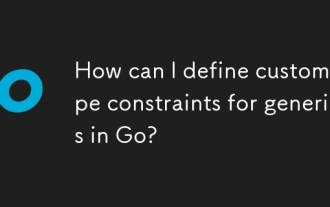
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
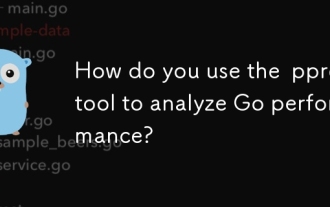
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
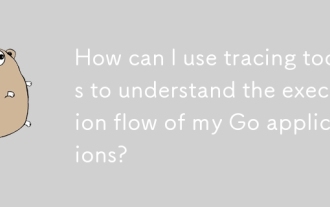
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
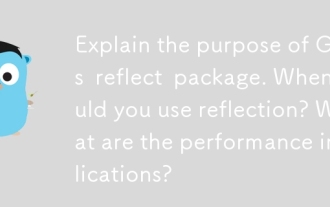
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
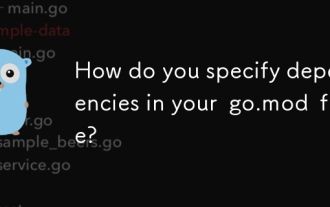
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
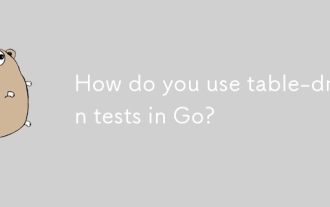
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
