How to delete files using Golang
Golang is a fast, reliable and very popular programming language. Its syntax is simple and powerful. It has been loved by more and more developers in recent years. When using Golang for file operations, you often need to delete files. This article will introduce how to use Golang to delete files.
There are two ways to delete files in Golang, namely os.Remove() and os.RemoveAll(). The main difference between them is that the former can only delete a single file, while the latter can delete directories and directories. All files and subdirectories.
First, let's take a look at the sample code that uses the os.Remove() function to delete a single file:
package main import ( "fmt" "os" ) func main() { //定义要删除的文件路径 var filePath string = "./test.txt" //删除文件 err := os.Remove(filePath) if err != nil { fmt.Printf("删除文件失败:%s\n", err) } else { fmt.Println("删除文件成功") } }
In the above sample code, the path of the file to be deleted is defined as ". /test.txt" and then use the os.Remove() function to delete the file. If an error occurs during the deletion process, an error message will be printed, otherwise "File deleted successfully" will be printed. It should be noted that if the file to be deleted does not exist, an error will be returned. You can use the os.IsNotExist(err) function to determine whether the file does not exist.
Next, let’s take a look at how to use the os.RemoveAll() function to delete all files in a directory and its subdirectories. Let's take deleting the subdirectory "testdir" in the current directory as an example:
package main import ( "fmt" "os" ) func main() { //定义要删除的目录路径 var dirPath string = "./testdir" //删除目录 err := os.RemoveAll(dirPath) if err != nil { fmt.Printf("删除目录失败:%s\n", err) } else { fmt.Println("删除目录成功") } }
In the above example code, the directory path to be deleted is defined as "./testdir", and then use os.RemoveAll() Function to delete all files in this directory and its subdirectories. Similarly, if an error occurs during the deletion process, an error message will be printed, otherwise "Directory deletion successful" will be printed. It should be noted that if the directory to be deleted does not exist, an error will be returned. You can use the os.IsNotExist(err) function to determine whether the directory does not exist.
In short, using the os.Remove() and os.RemoveAll() functions in Golang can easily delete a single file and all files in a directory and its subdirectories. Whether it is just deleting a file or clearing the entire directory and all its files, it can be quickly achieved through these two functions.
The above is the detailed content of How to delete files using Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


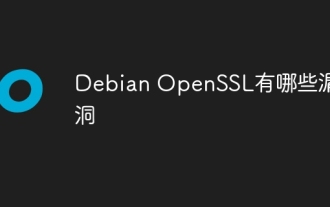
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
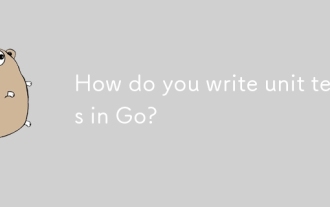
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
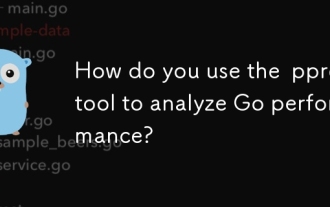
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
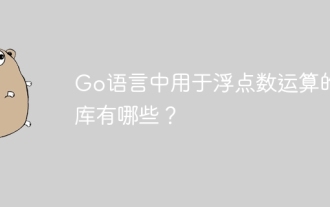
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
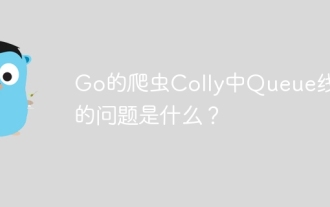
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
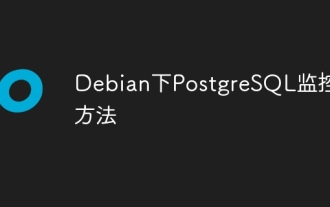
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
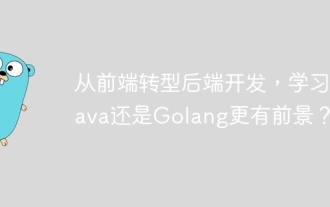
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
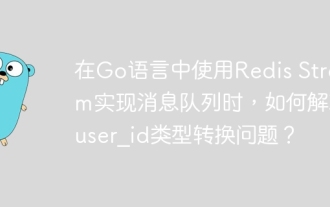
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
