A brief analysis of the use of .env files in laravel
When developing applications using the Laravel framework, you may need to set some environment variables. In a Laravel application, you can set these environment variables through the .env
file. This article will introduce how to use Laravel .env
file.
What is an .env file
The .env
file is a file that stores environment variables. In the Laravel framework, the .env
file usually stores some configuration information in the development environment, such as database connection information, email configuration information, etc. This information can be used anywhere in the application.
By default, the Laravel framework provides a .env.example
file in the root directory of the application. This file contains all available environment variables and their default values. You can make a copy and rename it to .env
, and then modify the variable values in it.
You can also use the putenv()
function that comes with PHP to set environment variables, but this method is not as convenient as the .env
file. When using this method, if you need to modify the environment variable value, you need to modify the code manually.
.env file format
The .env
file is a plain text file. It's very simple, just a set of key-value pairs, one per row. Keys and values are separated by =
. Each key-value pair needs to occupy one line, and there can be no spaces between the key name and the key value. For example:
APP_NAME=Laravel APP_ENV=local APP_DEBUG=true APP_URL=http://localhost DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=homestead DB_USERNAME=homestead DB_PASSWORD=secret
In this example, we set some basic environment variables, such as APP_NAME
, APP_ENV
, etc. These environment variables are usually used when your project is running.
How to use .env files
Reading environment variables in a Laravel application is very simple. You can access these variables using the built-in env()
function. This function accepts a key as a parameter and returns the value corresponding to the key. If the specified key is not found, returns the specified default value, if available.
The following is an example of using the .env
file:
// 从 .env 文件中获取 APP_ENV 环境变量的值 $env = env('APP_ENV'); // 从 .env 文件中获取 APP_DEBUG 环境变量的值 $debug = env('APP_DEBUG', false);
In this example, we use the env()
function to read The values of the APP_ENV
and APP_DEBUG
environment variables in the .env
file. For APP_DEBUG
, we also passed in a default value false
, indicating that this default value is used when the variable is not set in .env
.
The Laravel framework also provides a concise syntax to directly access variables in the .env
file, as shown below:
// 访问 APP_ENV 环境变量的值 $env = config('app.env'); // 访问 APP_DEBUG 环境变量的值 $debug = config('app.debug');
Here, we use ## directly #config() function, pass
app.env and
app.debug as arguments to it to access variables in the
.env file. Comments in the
.env file, you can add a
# before each key-value pair. Number. Everything after this symbol will be treated as a comment and ignored. For example:
# 这是一个注释 APP_NAME=Laravel APP_ENV=local # 这是另一个注释 APP_DEBUG=true APP_URL=http://localhost
.env file is used to store information about environment variables. These variables can be used anywhere in the application. You can use the
env() function or the
config() function to read these variables.
.env The file format is very simple, just a set of key-value pairs, one per line. If you need to add comments, you can add
# at the beginning of each line.
The above is the detailed content of A brief analysis of the use of .env files in laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


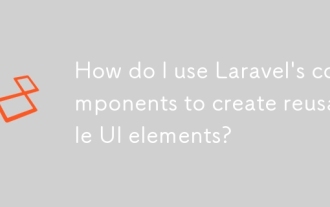
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
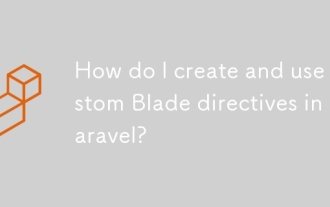
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
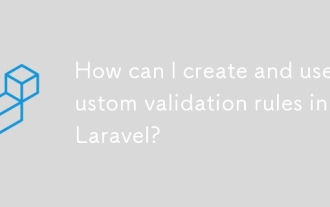
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
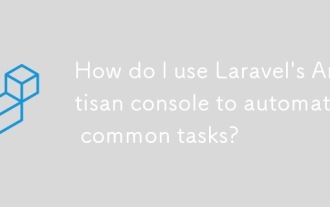
Laravel's Artisan console automates tasks like generating code, running migrations, and scheduling. Key commands include make:controller, migrate, and db:seed. Custom commands can be created for specific needs, enhancing workflow efficiency.Character
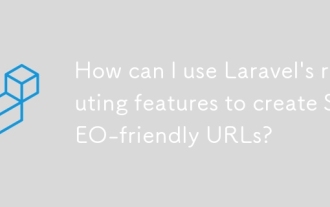
The article discusses using Laravel's routing to create SEO-friendly URLs, covering best practices, canonical URLs, and tools for SEO optimization.Word count: 159
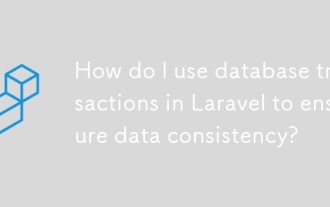
The article discusses using database transactions in Laravel to maintain data consistency, detailing methods with DB facade and Eloquent models, best practices, exception handling, and tools for monitoring and debugging transactions.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
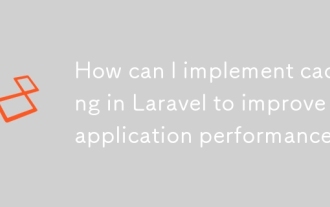
The article discusses implementing caching in Laravel to boost performance, covering configuration, using the Cache facade, cache tags, and atomic operations. It also outlines best practices for cache configuration and suggests types of data to cache
