Get and store time series data with Python
##Translator| Bugatti
Reviewer| Sun Shujuan
This tutorial will introduce how to Use Python to get time series data from the OpenWeatherMap API and convert it into a Pandas DataFrame. Next, we will use the InfluxDB Python Client to write this data to the time series data platform InfluxDB. We will convert the JSON response from the API call into a Pandas DataFrame as this is the easiest way to write data to InfluxDB. Since InfluxDB is a purpose-built database, our writes to InfluxDB are designed to meet the high requirements in terms of ingestion of time series data. RequirementsThis tutorial is completed on a macOS system that has Python 3 installed via Homebrew. It is recommended to install additional tools such as virtualenv, pyenv or conda-env to simplify the installation of Python and Client. The full requirements are here:1 2 3 4 |
|
- Created the bucket. You can think of buckets as the highest level of data organization in a database or InfluxDB. Token created.
1 2 3 4 5 |
|
1 2 3 4 5 6 7 |
|
1 2 3 4 5 6 7 |
|
- InfluxDB BucketInfluxDB OrganizationInfluxDB TokenInfluxDB URLOpenWeatherMap URLOpenWeatherMap Token
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
|
Figure 2. Navigate to the Script Editor and uncomment or delete the aggregateWindow() function to view the raw weather data
Conclusion
Hopefully this article helped you get the most out of InfluxDB Python Client library, obtains time series data and stores it in InfluxDB. If you want to learn more about using the Python Client library to query data from InfluxDB, I recommend you take a look at this article (https://thenewstack.io/getting-started-with-python-and-influxdb/). It's also worth mentioning that you can use Flux to get data from the OpenWeatherMap API and store it into InfluxDB. If you use InfluxDB Cloud, this means that the Flux script will be hosted and executed periodically, so you can get a reliable stream of weather data fed into the instance. To learn more about how to use Flux to obtain weather data on a user-defined schedule, please read this article (https://www.influxdata.com/blog/tldr-influxdb-tech-tips-handling-json-objects-mapping- arrays/?utm_source=vendor&utm_medium=referral&utm_campaign=2022-07_spnsr-ctn_obtaining-storing-ts-pything_tns).
The above is the detailed content of Get and store time series data with Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




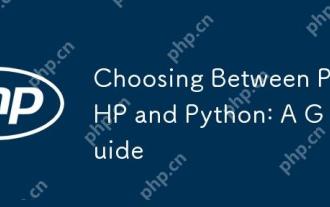
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
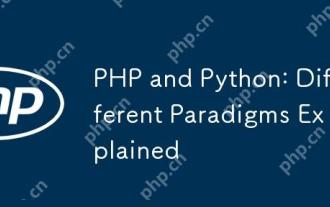
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
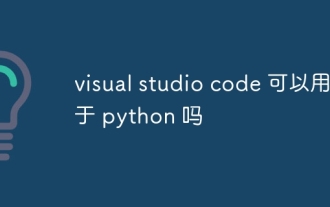
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
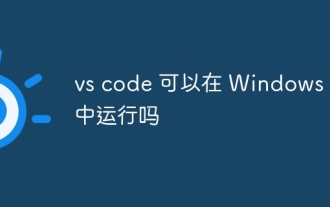
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
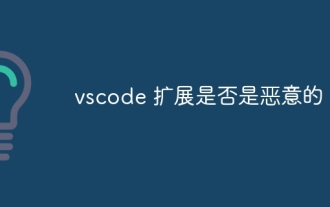
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
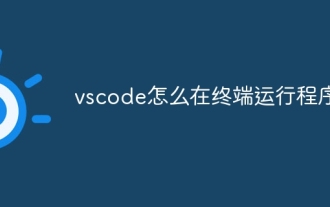
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
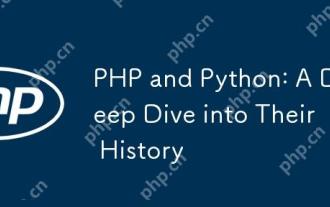
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
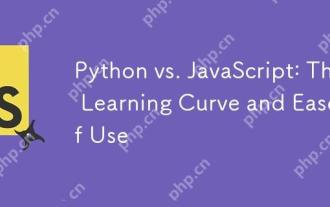
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
