


Python Dictionary: Is there any advanced gameplay that I don't know how to do?
Generate dictionary from sequence
We convert the following sequence into dict type.
lst = [('a', 1), ('b', 2), ('c', 3)]
Common writing method
for k, v in lst: dic[k] = v
More pythonic writing method
Use dictionary derivation to quickly generate a dictionary.
{k: v for k, v in lst}
Default value of key
When the specified key does not exist, set the value to 0.
Ordinary writing method
if key not in dct: dct[key] = 0
pythonic writing method
dct[key] = dct.get(key, 0)
Exchange key and value
Ordinary writing method
dic = {'Python': 1, 'Java': 2} new_dic = {} for k, v in dic.items(): new_dic[v] = k
More pythonic writing method
dic = {'Python': 1, 'Java': 2} new_dic = {v: k for k, v in dic.items()}
Sequence modification and initialization
Sample data
lst = [('a', 1), ('b', 2), ('c', 3)] dic = {'a': [0]}
If we need to update the data in dic based on lst, when the key exists, the value will be added to the end of the original sequence , otherwise initialize value and save it in sequence.
Ordinary writing method
for key, value in lst: if key in dic: dic[key].append(value) else: dic[key] = [value]
More pythonic writing method
for (key, value) in lst: group = dic.setdefault(key, []) group.append(value) # dic:{'a': [0, 1], 'b': [2], 'c': [3]}
setdefault(key, default) will first determine whether the key exists. If it exists, it will return dct[key], if it does not exist, it will return Then set dct[key] to [] and return.
Key, items set operation
If we now need to obtain the mapping information of the intersection of the keys of two dictionaries.
Ordinary writing method
dic1 = {'Python': 1, 'Java': 2, 'C': 3} dic2 = {'Python': 3, 'Java': 2, 'C++': 1} new_dic = {} for k, v in dic1.items(): if k in dic2.keys(): new_dic[k] = v print(new_dic) # {'Python': 1, 'Java': 2}
More pythonic writing method
dic1 = {'Python': 1, 'Java': 2, 'C': 3} dic2 = {'Python': 3, 'Java': 2, 'C++': 1} print({k: dic1[k] for k in dic1.keys() & dic2.keys()}) # {'Python': 1, 'Java': 2}
The dic1.keys() & dic2.keys() here use keys() for set operations , items() can also perform set operations.
If we now want to obtain the same key and value parts in two dictionaries
dic1 = {'Python': 1, 'Java': 2, 'C': 3} dic2 = {'Python': 3, 'Java': 2, 'C++': 1} print(dic1.items() & dic2.items()) # {('Java', 2)}
Flexibly use the characteristics of keys and items() set operations to quickly extract the content we want.
Sort the dictionary by key or value
Use the sorted() function to quickly sort the key or value.
dic = {'a': 2, 'b': 1, 'c': 3, 'd': 0} lst1 = sorted(dic.items(), key=lambda x: x[0], reverse=False) # [('a', 2), ('b', 1), ('c', 3), ('d', 0)] lst2 = sorted(dic.items(), key=lambda x: x[1], reverse=False) # [('d', 0), ('b', 1), ('a', 2), ('c', 3)] print('按照键降序:', {key: value for key, value in lst1}) print('按照值降序:', {key: value for key, value in lst2}) # 按照键降序: {'a': 2, 'b': 1, 'c': 3, 'd': 0} # 按照值降序: {'d': 0, 'b': 1, 'a': 2, 'c': 3}
Multiple dictionary sorting
If a sequence contains multiple dictionaries, these dictionaries must now be sorted according to conditions. This can also be achieved using the sorted() function.
dict_list = [ {'letter': 'B', 'number': '2'}, {'letter': 'A', 'number': '3'}, {'letter': 'B', 'number': '1'} ] # 按 letter 排序 print(sorted(dict_list, key=lambda dic: dic['letter'])) # 按 letter, number 排序 print(sorted(dict_list, key=lambda dic: (dic['letter'], dic['number']))) # [{'letter': 'A', 'number': '3'}, {'letter': 'B', 'number': '2'}, {'letter': 'B', 'number': '1'}] # [{'letter': 'A', 'number': '3'}, {'letter': 'B', 'number': '1'}, {'letter': 'B', 'number': '2'}]
Of course, if you know itemgetter(), the above code can be changed and the execution speed will be faster.
from operator import itemgetter print(sorted(dict_list key=itemgetter('letter'))) print(sorted(dict_list, key=itemgetter('letter', 'number')))
itemgetter() does not obtain a value, but defines a function through which it is applied to the target object.
The above is the detailed content of Python Dictionary: Is there any advanced gameplay that I don't know how to do?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


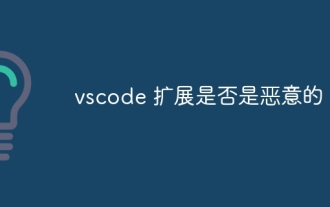
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
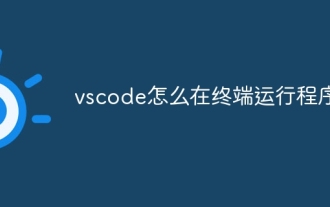
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
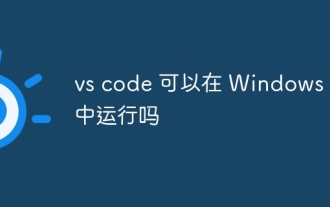
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
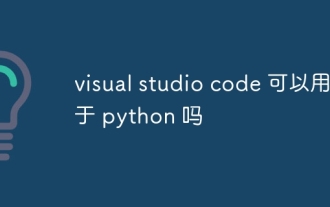
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
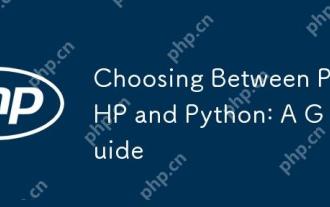
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
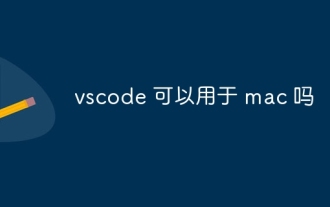
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
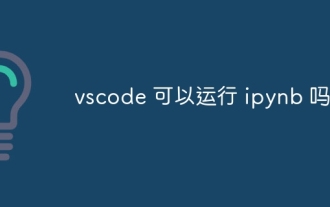
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
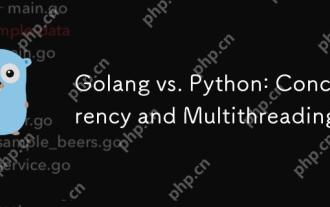
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
