Discuss in detail how to dynamically add instructions in Vue
Apr 12, 2023 am 09:18 AMVue.js is the most widely used framework among front-end development engineers. The framework provides us with many development conveniences, especially its command system. Vue directives can implement very convenient and flexible data binding and event listening. They are core concepts of the Vue template language, and these directives allow us to manipulate DOM elements just like in JavaScript. During development, we often encounter situations where we need to dynamically add instructions. Next, we will discuss in detail how to implement Vue to dynamically add instructions.
Vue dynamically adds demand scenarios for instructions
In many cases, we need to change the behavior of dynamic instructions based on changes in data. For example, in a form page, the content and number of form items may change depending on the form type selected by the user. And we need to dynamically add some instructions, such as form validation instructions, Tab switching instructions, etc.
The core issue in implementing dynamic addition instructions is: how to bind dynamic data to DOM elements and trigger corresponding behaviors when the data changes. Next, we will teach you two methods to dynamically add instructions in Vue.
Option 1: Use Vue.directive()
Vue creates a custom directive through Vue.directive()
. We can call this function and pass a directive name and definition object. This definition object can have some hook functions, such as bind()
, inserted()
, update()
, componentUpdated()
and unbind()
, these hook functions can be called when instructions are bound, inserted into elements, updated elements and components update and unbind instructions respectively.
Now, let's see how to dynamically add instructions through Vue.directive()
. We can use a computed property during data binding, depending on the value in the required directive list property.
This is an example:
Vue.directive('bg-color', { bind: function (el, binding) { el.style.backgroundColor = binding.value } })
In the above example, we defined a custom directive named bg-color
, where the directive is bound to the element , the element's background color is updated to the value bound to the directive. Here you can pass a value binding.value
(also called binding value). In this example, the background color is dynamically bound to the component's data value.
Option 2: Use the Vue plug-in
In addition to using Vue.directive()
, we can also use the Vue plug-in to dynamically add instructions. Vue plugins can be installed and run globally, which provides us with many opportunities to add new capabilities to Vue and its subcomponents that call component global configuration. A plug-in usually exposes several options on itself, allowing us to customize some of the plug-in's behavior.
For example, in a large project, you may need to add some validation functions to each form element. You can use plugins to add these validation functions to the elements that require these validations.
import Vue from 'vue' const MyPlugin = {} MyPlugin.install = function (Vue, options) { Vue.directive('bg-color', { bind: function (el, binding) { el.style.backgroundColor = binding.value } }) } Vue.use(MyPlugin)
In this example, we import Vue and create a plugin named MyPlugin
, and then define bg-color in the
install() method
Behavior of the directive. Finally, we register the plugin using the Vue.use() method. This plugin can now pass arbitrary options in the application and be referenced into any component.
Summary
The core of Vue's dynamic addition of instructions is to use Vue.directive() or Vue plug-in form to implement dynamic instruction binding. In actual development, different solutions are selected according to demand scenarios, and the required functions can be better achieved in terms of detailed implementation. The above two implementation methods can better meet the needs of dynamic instruction binding development.
The above is the detailed content of Discuss in detail how to dynamically add instructions in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
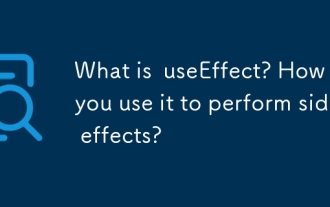
What is useEffect? How do you use it to perform side effects?
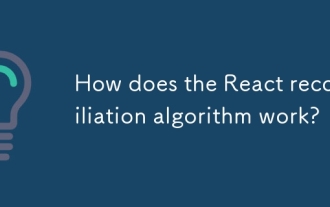
How does the React reconciliation algorithm work?
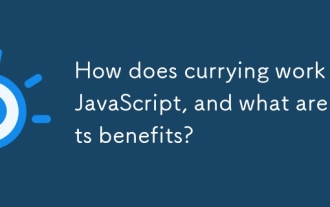
How does currying work in JavaScript, and what are its benefits?

What are higher-order functions in JavaScript, and how can they be used to write more concise and reusable code?
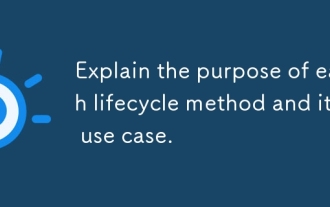
Explain the purpose of each lifecycle method and its use case.
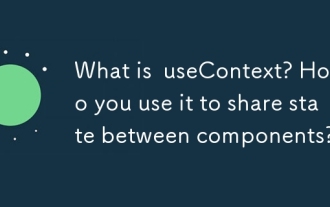
What is useContext? How do you use it to share state between components?
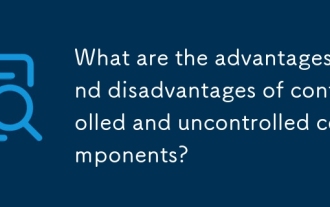
What are the advantages and disadvantages of controlled and uncontrolled components?
