


How to determine whether a collection contains a specified element in php
To determine whether a collection contains an element in PHP, you first need to understand what collections and elements are. A set is an unordered collection of elements, which are the individual items in the set. In computer science, a set is a data structure that can be used to store a group of individual items.
In PHP, we can use arrays to simulate sets, and use the in_array() function to determine whether the set contains specific elements. At the same time, PHP also provides the set class to implement collection-like functions. This method will be covered in a later article.
Using the in_array() function
In PHP, you can use the in_array() function to check whether an array contains a specific element. The syntax of this function is as follows:
bool in_array ( mixed $needle , array $haystack [, bool $strict = FALSE ] )
where needle specifies the element to be found, and haystack is the array to be searched. strict is an optional parameter. If it is set to true, the in_array() function will compare the types of needle and haystack, otherwise it will only compare their values.
The following is an example that demonstrates how to use the in_array() function to determine whether an array contains a specific element:
$fruit = array('apple', 'banana', 'orange', 'grape'); if (in_array('apple', $fruit)) { echo "Found apple!"; } else { echo "Apple not found."; }
In the above example, we first define an array of fruits, and then Use the in_array() function to check if the array contains an 'apple' element. Since the array contains 'apple' elements, the output is as follows:
Found apple!
We can also use the strict parameter to compare value types.
$number = array('1', 2, '3'); if (in_array(2, $number, true)) { echo "Found 2!"; } else { echo "2 not found."; }
In the above example, we defined an array of numbers and used the in_array() function to check whether the array contains the '2' element. We set the strict parameter to true in the function call, which means that the in_array() function will compare the types of needle and haystack, not just their values. Since the array contains the number 2, and we specified a number in the needle parameter, the array contains the element, so the output result is as follows:
Found 2!
Use the set class
in php5. In versions 6 and later, PHP provides the set class to implement collection-like functions. The set class defines multiple functions for manipulating collections. Specifically, collections can be manipulated using methods such as add(), delete(), contains(), isEmpty(), and count().
$set = new \Ds\Set(['apple', 'banana', 'orange']); if ($set->contains('apple')) { echo "Found apple!"; } else { echo "Apple not found."; }
In the above example, we first create a fruit collection and use the contains() method to check whether the collection contains the 'apple' element. Since the collection contains 'apple' elements, the output is as follows:
Found apple!
We can also use the add() and delete() methods to add and delete elements:
$set = new \Ds\Set(['apple', 'banana', 'orange']); $set->add('grape'); $set->delete('banana'); print_r($set->toArray());
In the above In the example, we first create a fruit collection, and then use the add() method to add an element to the collection. Next, we use the delete() method to delete the 'banana' element. Finally, we use the toArray() method to convert the collection to an array and print it out. The output is as follows:
Array ( [0] => apple [1] => orange [2] => grape )
Conclusion
Determining whether a set contains a certain element is a basic operation that we often need to use in programming. In PHP, we have many ways to implement this operation, including using the in_array() function and the set class. Which method we choose depends on our specific needs and preferences.
The above is the detailed content of How to determine whether a collection contains a specified element in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


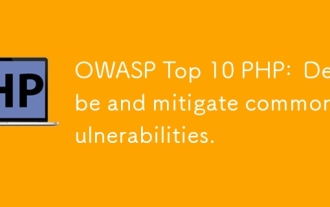
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
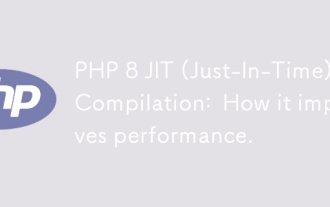
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
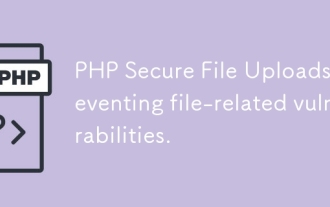
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
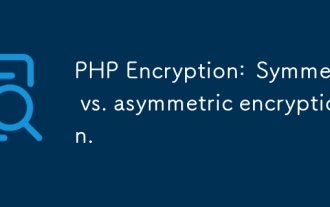
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
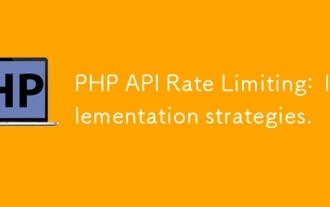
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
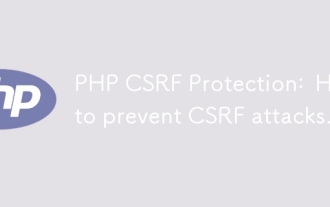
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
