Deque in Python: Implementing efficient queues and stacks
Deque in Python is a low-level, highly optimized double-ended queue, useful for implementing elegant and efficient Pythonic queues and stacks, which are the most common list-based data types in computing.
In this article, Mr. Yun Duo will learn the following together with you:
- Start using deque
- Effectively pop up and append elements
- Access deque Any element in
- Use deque to build an efficient queue
Start using Deque
The operation of appending elements to the right end of the Python list and popping elements is generally very efficient. If time complexity is expressed in Big O, then we can say that they are O(1). When Python needs to reallocate memory to increase the underlying list to accept new elements, these operations will slow down and the time complexity may become O(n).
In addition, the operations of appending and popping elements to the left end of the Python list are also very inefficient, with a time complexity of O(n).
Since lists provide .append() and .pop() operations, they can be used as stacks and queues. The performance issues of appending and popping operations on the left and right ends of the list will greatly affect the overall performance of the application.
Python’s deque was the first data type added to the collections module as early as Python 2.4. This data type is specifically designed to overcome the efficiency issues of .append() and .pop() in Python lists.
Deques are sequence-like data types designed as a generalization of stacks and queues. They support memory-efficient and fast append and pop operations on both ends of the data structure.
Append and pop operations on both ends of a deque object are stable and equally efficient because the deque is implemented as a doubly linked list. Additionally, append and pop operations on deque are also thread-safe and memory-efficient. These features make deque particularly useful for creating custom stacks and queues in Python.
If you need to save the last seen element list, you can also use deque, because the maximum length of deque can be limited. If we do this, then once the deque is full, it will automatically discard the elements at one end when we add new elements at the other end.
The following is a summary of the main features of deque:
- Stores elements of any data type
- Is a variable data type
- Supports in The member operations of the operator
- support indexing, such as a_deque[i]
- does not support slicing, such as a_deque[0:2]
- supports sequences and iterable objects. Built-in functions for operations, such as len(), sorted(), reversed(), etc.
- Does not support inplace sorting
- Supports normal iteration and reverse iteration
- Supports the use of pickle
- Ensure fast, memory efficient, and thread-safe pop and append operations on both ends
Creating a deque instance is relatively simple. Just import deque from the collection and call it with an optional iterator as argument.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
|
If you instantiate deque without providing iterable as a parameter, you will get an empty deque. If an iterable is provided and entered, the deque initializes a new instance with its data. Initialization proceeds from left to right using deque.append().
Deque initializer requires the following two optional parameters.
- iterable An iterator that provides initialization data.
- maxlen is an integer specifying the maximum length of deque.
As mentioned before, if you don't provide an iterable, then you will get an empty deque. If you provide a value for maxlen, then your deque will only store the items up to maxlen.
Finally, you can also use unordered iterable objects, such as collections, to initialize deque. In these cases, there will be no predefined order of elements in the final deque.
Efficiently popping and appending elements
The most important difference between Deque and List is that the former can perform efficient appending and popping operations at both ends of the sequence. The Deque class implements specialized .popleft() and .appendleft() methods to directly operate on the left end of the sequence.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
Here, use .popleft() and .appendleft() to pop up and increase the left end value of numbers respectively. These methods are designed for deque, and list has no such method.
Deque also provides .append() and .pop() methods like list to operate on the right end of the sequence. However, the behavior of .pop() is different.
1 2 3 4 5 6 7 8 9 10 |
|
Here, .pop() removes and returns the last element in the deque container. This method does not accept an index as a parameter, so it cannot be used to remove arbitrary items from the deque. You can only use it to remove and return the rightmost item.
We think deque is a doubly linked list. Therefore, each item in a given deque container holds a reference (pointer) to the previous and next item in the sequence.
Double linked lists make the operation of adding and popping elements from both ends simple and efficient, because only the pointers need to be updated, therefore, the two operations have similar performance, both are O(1). They are also predictable in terms of performance because there is no need to reallocate memory and move existing items to accept new items.
从常规 Python 列表的左端追加和弹出元素需要移动所有元素,这最终是一个 O(n) 操作。此外,将元素添加到列表的右端通常需要Python重新分配内存,并将当前项复制到新的内存位置,之后,它可以添加新项。这个过程需要更长的时间来完成,并且追加操作从 O(1)传递到 O(n)。
考虑以下关于在序列左端添加项的性能测试,deque vs list。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
在这个脚本中,average_time() 计算了执行一个给定次数的函数(func)的平均时间。如果我们在命令行中运行该脚本,那么我们会得到以下输出。
1 2 3 |
|
在这个例子中,deque 上的 .appendleft() 要比 list 上的 .insert() 快几倍。注意 deque.appendleft() 执行时间是常量O(1)。但列表左端的 list.insert() 执行时间取决于要处理的项的数量O(n)。
在这个例子中,如果增加 TIMES 的值,那么 list.insert() 会有更高的时间测量值,而 deque.appendleft() 会得到稳定(常数)的结果。如果对 deque 和 list 的 pop 操作进行类似的性能测试,那么可以展开下面的练习块。
Exercise:测试 deque.popleft() 与 list.pop(0) 的性能
可以将上面的脚本修改为时间deque.popleft()与list.pop(0)操作并估计它们的性能。
Solution:测试 deque.popleft() 与 list.pop(0) 的性能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
1 2 3 4 5 |
|
Deque 数据类型的设计是为了保证在序列的两端进行有效的追加和弹出操作。它是处理需要在 Python 中实现队列和堆栈数据结构的问题的理想选择。
访问Deque中的任意元素
Python 的 deque 返回可变的序列,其工作方式与列表相当类似。除了可以有效地从其末端追加和弹出元素外,deque 还提供了一组类似列表的方法和其他类似序列的操作,以处理任意位置的元素。下面是其中的一些。
选项 | 描述 |
.insert(i, value) | 在索引为i的deque容器中插入一个名为value的元素。 |
.remove (value) | 删除第一个出现的 value ,如果 value 不存在则引发ValueError |
a_deque[i] | 从一个deque容器中检索索引为 i 的项。 |
del a_deque[i] | Removes the item with index i from the deque container. |
我们可以使用这些方法和技术来处理 deque 对象内部任何位置的元素。下面是如何做到这一点的。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
在这里,首先将"c"插入到位置 2的letters中。然后使用 .remove() 从deque容器中移除"d"。Deque 还允许索引来访问元素,在这里使用它来访问索引1处的b。最后,你可以使用 del 关键字从 deque 中删除任何存在的项。请注意, .remove() 允许按值删除项,而del则按索引删除项。
尽管 deque 对象支持索引,但它们不支持切片,即不能像常规列表一样使用切片语法, [start:stop:step] 从现有的 deque 中提取:
1 2 3 4 5 6 |
|
Deque支持索引,却不支持分片。通常来说在一个链表上执行切片非常低效。
虽然 deque 与 list 非常相似,但 list 是基于数组的,而 deque 是基于双链表的。
Deque 基于双链表,在访问、插入和删除任意元素都是无效操作。如果需要执行这些操作,则解释器必须在deque中进行迭代,直到找到想要的元素。因而他们的时间复杂度是O(n)而不是O(1)。
下面演示了在处理任意元素时 deques 和 list 的行为。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
|
这个脚本对插入、删除和访问一个 deque 和一个 list 中间的元素进行计时。如果运行这个脚本,得到如下所示的输出:
1 2 3 |
|
Deque并不像列表那样是随机访问的数据结构。因此,从 deque 的中间访问元素的效率要比在列表上做同样的事情低。这说明 deque 并不总是比列表更有效率。
Python 的 deque 对序列两端的操作进行了优化,所以它们在这方面一直比 list 好。另一方面,列表更适合于随机访问和固定长度的操作。下面是 deque 和 list 在性能上的一些区别。
对于列表,当解释器需要扩大列表以接受新项时,.append()的性能优势受到内存重新分配的影响而被降低。此操作需要将所有当前项复制到新的内存位置,这将极大地影响性能。
此总结可以帮助我们为手头的问题选择适当的数据类型。但是,在从列表切换到 deque 之前,一定要对代码进行剖析,它们都有各自的性能优势。
用Deque构建高效队列
Deque 是一个双端队列,提供了堆栈和队列的泛化。在本节中,我们将一起学习如何使用deque以优雅、高效和Pythonic的方式在底层实现我们自己的队列抽象数据类型(ADT)。
注意: 在 Python 标准库中,queue 模块实现了多生产者、多消费者的队列,可以在多个线程之间安全地交换信息。
如果你正在处理队列,那么最好使用那些高级抽象而不是 deque ,除非你正在实现自己的数据结构。
队列是元素的collections,可以通过在一端添加元素和从另一端删除元素来修改队列。
队列 以先入先出(FIFO)的方式管理元素,像一个管道一样工作,在管道的一端推入新元素,并从另一端弹出旧元素。向队列的一端添加一个元素称为 enqueue 操作;从另一端删除一个元素称为 dequeue。
为了更好地理解队列,以餐厅为例,餐馆里有很多人在排队等着点餐。通常情况下,后来的人将排在队列的末端。一旦有了空桌子,排在队伍开头的人就会离开队伍进去用餐。
下面演示了使用一个原始的deque对象来模拟这个过程。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
首先创建一个空的 deque 对象来表示到达餐厅的人的队列。person排队放入队列,可以使用.append(),将单个条目添加到右端。要从队列中取出一个person,可以使用.popleft() ,删除并返回deque容器左侧的各个条目。
用队列模拟工作,然而,由于deque是一个泛化,它的API]不匹配常规的队列API。例如,不是.enqueue(),而是.append()。还有.popleft() 而不是.dequeue()。此外,deque 还提供了其他一些可能不符合特定需求的操作。
我们可以创建具有特定功能的自定义队列类。可以在内部使用 deque 来存储数据,并在自定义队列中提供所需的功能。我们可以把它看作是适配器设计模式的一个实现,在这个模式中,把 deque 的接口转换成看起来更像队列接口的东西。
例如,需要一个自定义的队列抽象数据类型,提供以下功能。
- 排列元素
- 去排队的元素
- 返回队列的长度
- 支持成员资格测试
- 支持正常和反向迭代
- 提供一个方便用户的字符串表示法
此时可以写一个Queue类。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
._items 是一个 deque 对象,可以存储和操作队列中的元素。Queue使用 deque.append() 实现了 .enqueue(),将元素添加到队列的末端。还用 deque.popleft() 实现了 .dequeue(),以有效地从队列的开头删除元素。
支持以下特殊方法
运作 | | |
通过索引访问任意的元素 | O(n) | O(1) |
在左端弹出和追加元素 | O(1) | O(n) |
Pop and append elements at the right end | O (1) | ##O(1) Redistribute |
Insert and delete elements in the middle | O(n) | O(n) |
理想情况下,.__repr__()返回一个字符串,代表一个有效的 Python 表达式。可以用这个表达式以相同的值重新创建这个对象。
然而,在上面的例子中,目的是使用方法的返回值在 interactive shell 上优雅地显示对象。可以通过接受初始化可迭代对象作为.__init__() 的参数并从中构建实例,从而从这个特定的字符串表示形式构建 Queue 实例。
有了这些补充,Queue 类就完成了。要在我们的代码中使用这个类,我们可以做如下事情。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
|
总结
队列和堆栈是编程中常用的 抽象数据类型。它们通常需要在底层数据结构的两端进行有效的 pop 和 append 操作。Python 的 collections 模块提供了一种叫做 deque 的数据类型,它是专门为两端的快速和节省内存的追加和弹出操作而设计的。
有了deque,我们可以用优雅、高效和Pythonic的方式在低层次上编写我们自己的队列和堆栈。
总结下本文所学内容:
- 如何在代码中创建和使用Python的deque
- 如何有效地从deque的两端追加和弹出项目
- 如何使用deque来构建高效的队列和堆栈
- 什么时候值得使用deque而不是list
The above is the detailed content of Deque in Python: Implementing efficient queues and stacks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


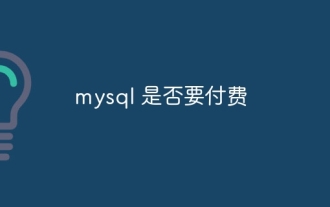
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.

HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
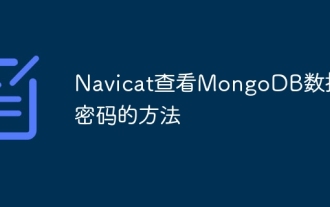
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
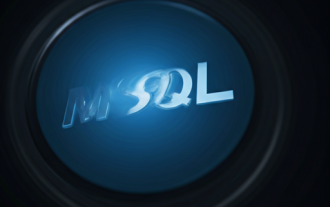
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
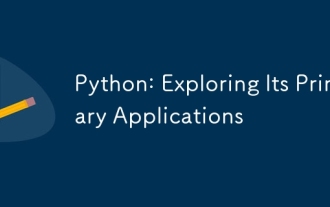
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
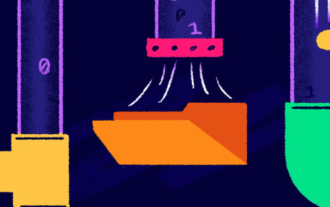
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
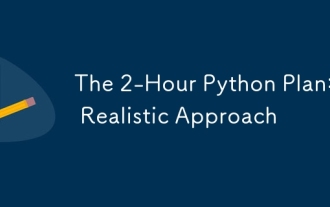
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
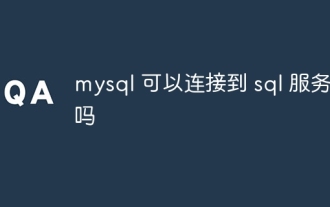
No, MySQL cannot connect directly to SQL Server. But you can use the following methods to implement data interaction: Use middleware: Export data from MySQL to intermediate format, and then import it to SQL Server through middleware. Using Database Linker: Business tools provide a more friendly interface and advanced features, essentially still implemented through middleware.

Method | Support |
| length of |
## .__contains__( ) | Member test with in |
.__iter__() | Regular iteration |
.__reversed__() | Reverse iteration |
.__repr__() | String representation |