How Golang uses RPC to implement forwarding services
RPC is a remote calling protocol used to implement method calls between different processes. In microservices architecture, it is widely used for communication between services. In Golang, we can easily implement RPC calls through the net/rpc package provided in the standard library. This article will introduce how to use RPC to implement forwarding services in Golang.
First of all, we need to understand the basic concepts of RPC. In RPC, there are two important roles: client and server. The client calls the methods exposed by the server, and the server receives the request from the client and returns the corresponding result. To implement RPC in Golang, we need to first define a service object:
type ForwardService struct {} func (s *ForwardService) Forward(req string, resp *string) error { // 处理请求,将结果写入resp中 return nil }
This ForwardService is the service we need to expose. A Forward method is defined in it to receive requests from the client and process the results. Write to resp. Here we directly set the data type of the request and response to the string type, which can be adjusted as needed in actual situations.
Next, we need to register this service object with the RPC service:
func main() { // 创建服务对象 service := new(ForwardService) // 注册服务 rpc.Register(service) // 启动服务 listener, err := net.Listen("tcp", ":8080") if err != nil { log.Fatal("Listen error: ", err) } for { conn, err := listener.Accept() if err != nil { log.Fatal("Accept error: ", err) } go rpc.ServeConn(conn) } }
In this code, we first create a ForwardService object named service, and then pass rpc. Register registers it into the RPC service. Finally, we started a TCP listener and continuously accepted connection requests from the client, and started a new goroutine to handle RPC requests on this connection.
With this service object and RPC service, we can start to implement the forwarding service. In this forwarding service, we need to forward the request from the client to the specified target service and obtain the response, and then return the response to the client. The following is a simple forwarding service implementation:
func Forward(req string) (string, error) { // 连接目标服务 conn, err := net.Dial("tcp", "target:8080") if err != nil { return "", err } defer conn.Close() // 构造RPC请求 client := rpc.NewClient(conn) var resp string err = client.Call("ForwardService.Forward", req, &resp) if err != nil { return "", err } return resp, nil }
This forwarding method receives a request req, then connects to the RPC address of the target service through net.Dial, and then constructs an RPC request and calls the client.Call method Send a request and get a response. Finally, the forwarding method returns the fetched response.
In actual use, we can wrap the Forward method into an HTTP interface to facilitate client calls:
func ForwardHandler(w http.ResponseWriter, r *http.Request) { req, _ := ioutil.ReadAll(r.Body) resp, err := Forward(string(req)) if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return } w.Write([]byte(resp)) } func main() { http.HandleFunc("/", ForwardHandler) log.Fatal(http.ListenAndServe(":8080", nil)) }
This HTTP interface receives requests from the client and forwards them by calling the forwarding method Forward. The request is forwarded to the specified target service and the obtained response is returned to the client. Throughout the process, we used the net/rpc package provided in the Golang standard library to implement a simple and efficient RPC forwarding service.
To summarize, this article mainly introduces the method of using RPC to implement forwarding services in Golang. We first defined a ForwardService service object and registered it in the RPC service. Then, connect to the RPC address of the target service through net.Dial, and implement request forwarding and response acquisition through RPC requests. Finally, we wrap the forwarding method into an HTTP interface to facilitate client calls.
The above is the detailed content of How Golang uses RPC to implement forwarding services. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


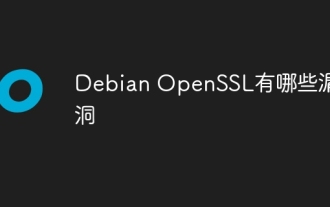
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
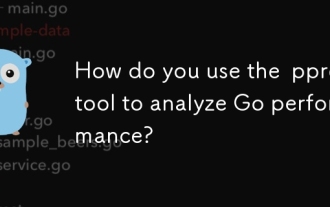
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
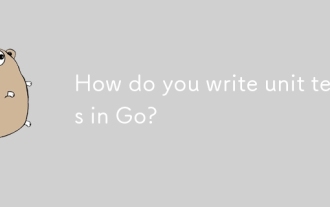
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
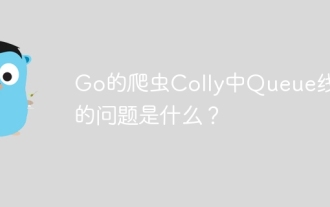
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
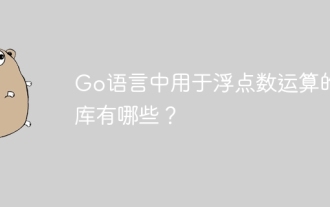
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
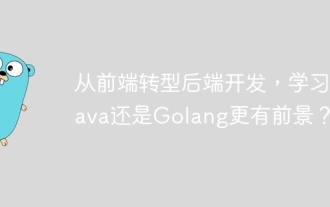
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
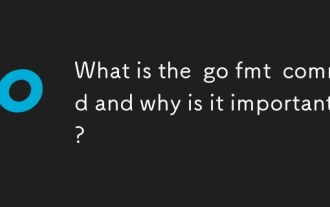
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
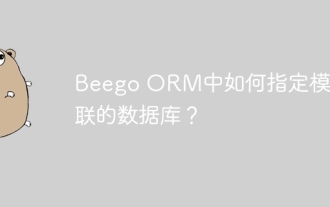
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
