How to set an auto-increment key in PHP
In PHP development, looping is an important operation. Loops allow a program to repeatedly execute a block of statements according to a certain pattern. In a loop, sometimes it is necessary to count the number of loops. In this case, it is necessary to set an auto-increment key to realize the counting function. This article will explain how to set an auto-increment key in PHP.
In PHP, the commonly used loop structures are for loop and foreach loop. In a for loop, the number of loops can be controlled through three parts: initialization, conditions, and operations. In the foreach loop, you can directly traverse an array or object to implement the loop.
It is very simple to set an auto-incrementing key in a for loop. You can define a variable before the loop starts, and then increment the variable after each loop. The specific implementation is as follows:
for($i=0; $i<10; $i++){ echo $i . "<br>"; }
In the above example, the variable $i is initialized to 0, and increases by 1 each time in the loop until $i is greater than or equal to 10, and the loop ends. In the loop, you can use the variable $i to implement the counting function.
If you want to set an auto-increment key in the foreach loop, you can use the special variable $key in PHP. The $key variable stores the key value of the currently traversed array or object. During traversal, you can use the symbol to increment the $key variable. The specific implementation is as follows:
$array = array("apple", "banana", "orange"); foreach ($array as $key => $value) { echo $key . ": " . $value . "<br>"; $key++; }
In the above example, each element in the array $array is traversed once. When traversing, the variable $key is automatically assigned a value based on the array index, and then increments after each loop. In this way, the variable $key can implement the counting function.
It should be noted that modifying the $key variable in the foreach loop may cause some problems. Because the $key variable is a special variable in PHP, modifying it may cause code exceptions or errors in some cases. If it is not necessary, it is recommended not to modify the value of the $key variable, but to use an additional counting variable to implement the counting function.
In actual development, you need to choose which loop and counting method to use based on specific needs. Whether you use a for loop or a foreach loop, you can implement the counting function through the increment operation. Hope this article is helpful to PHP developers.
The above is the detailed content of How to set an auto-increment key in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


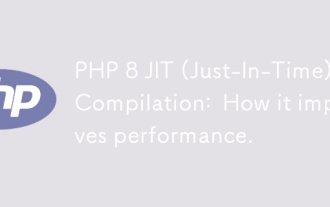
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
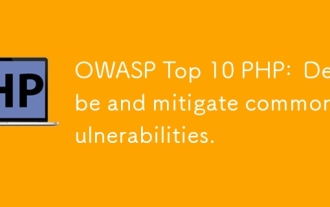
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
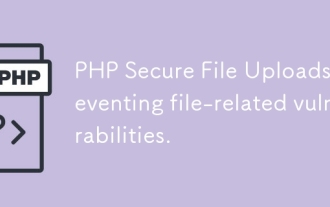
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
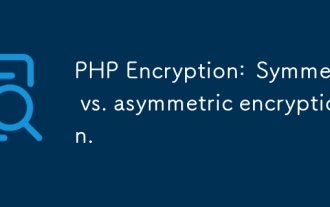
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
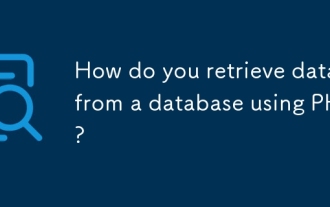
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
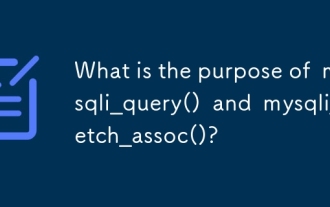
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
