How to implement click event throttling in vue
In Vue project development, we may encounter situations where click events are frequently triggered. For example, if a user continuously clicks a button, it may cause abnormal behavior on the page and affect the user experience. Therefore, in this case, we need to throttle the click event to avoid affecting the user experience. This article will introduce how to implement click event throttling in Vue.
1. What is click event throttling
Click event throttling is a method to optimize front-end performance. It allows us to limit an action to only be executed once within a certain period of time. For example, when a user triggers click events multiple times in a short period of time, we want to only execute the last click event instead of executing it every time. In this case, we can use click event throttling to deal with the problem.
2. Why use click event throttling
In web development, we often encounter pages similar to "waterfall flow". Users need to click frequently to load more pages. data. If we do not use throttling, multiple requests will be sent to the backend in a short period of time, which will not only cause performance problems, but also affect the user experience.
Using throttling can limit the frequency of user operations, avoid abnormal page behavior, and optimize the user experience.
3. How to implement throttling in Vue
Vue provides a very convenient instruction v-throttle
to implement click event throttling. Next, let’s take a look at the implementation of v-throttle
.
- Installation
v-throttle
We can install v-throttle
through npm, use the following command:
npm install --save v-throttle
- Using
v-throttle in Vue
First, we need to introduce the v-throttle
directive in the Vue component, And use this directive where throttling operations are required. For example, in the following example, we create a button and use the v-throttle
directive to limit the click frequency of the button:
<template> <button v-throttle:click="btnClick">Click Me!</button> </template> <script> import throttle from 'v-throttle'; export default { methods: { btnClick: throttle(function() { console.log('click') }, 1000) } } </script>
In the above example, we pass a The function gives the v-throttle
instruction and specifies a time interval of 1000 milliseconds. This means that when the user clicks the button, the click event is executed at most once every 1000 milliseconds.
4. Implementation Principle
In Vue, the implementation principle of the v-throttle
instruction is to utilize the closure feature of functions in Javascript. Specifically, the click event processing function is encapsulated in a closure, and the time when the function was last executed is recorded at the same time. Each time the user clicks the button, we will check whether the current time meets the time interval requirements, execute the function if it does, and update the time record of the last time the function was executed.
Next, let’s take a look at the code implementation of the v-throttle
instruction:
import throttle from 'lodash-es/throttle'; export default { bind(el, binding) { const delay = parseInt(binding.arg) || 500; const method = binding.value; const throttled = throttle(method, delay); el.addEventListener('click', throttled); }, unbind(el, binding) { const method = binding.value; el.removeEventListener('click', method); } }
In the above code, we first introduced the in the Lodash library throttle
function and combine this function with the processing function bound to the instruction. Then, when the directive is bound, we add a hook function bind
that will be executed when the directive is bound to the element. In this hook function, we use the addEventListener
function to bind the throttled
function to the element's click
event.
Finally, when the directive is unbind, we added another hook function unbind
, which will be executed when the directive is unbind from the element, and utilize removeEventListener
Function to remove the event handler from the element's event listener. This ensures that the directive is unbound from the element.
5. Summary
Using click event throttling can avoid frequent events and optimize front-end performance. The Vue framework provides the v-throttle
directive to facilitate us to implement this function. Through the introduction and analysis of the implementation principle of this directive, we can better understand how throttling works and how to implement click event throttling in Vue.
The above is the detailed content of How to implement click event throttling in vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
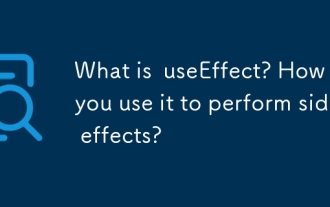
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
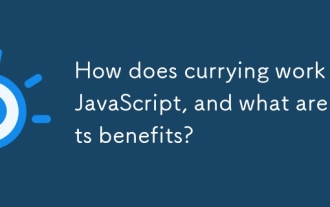
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
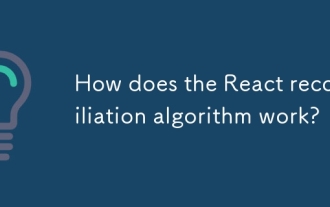
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
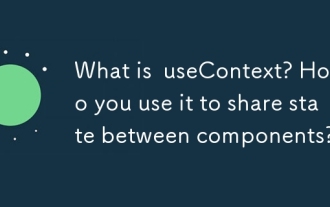
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
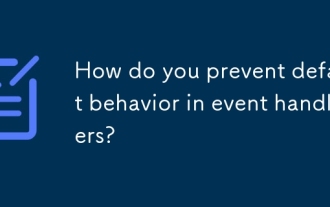
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
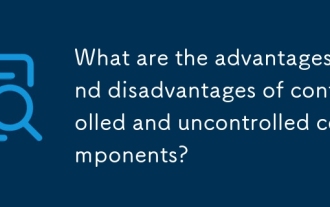
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
