Let's talk about the implementation mechanism of Vue data binding
Vue.js is a modern JavaScript framework, one of the most important features is two-way data binding. It means that when the data changes, the view will automatically update, and when the view changes, the data will also automatically update. This feature brings great convenience to developers, allowing us to focus more on the implementation of business logic instead of constantly manually updating views and data. In Vue, the implementation mechanism of data binding has the following aspects:
- Responsive object
The reactive implementation in Vue is by defining a reactive object. Mark certain properties on an object as monitorable. When these properties change, Vue updates the related views. The specific implementation uses the Object.defineProperty method of ES5 to hijack the getter and setter methods of the property.
First, we define a simple template. The template contains an input and a span element. The value attribute of the input and the textContent attribute of the span are data bound:
<div id="app"> <label>输入内容:</label> <input v-model="message"> <p>输出内容:{{ message }}</p> </div>
Then We define a reactive object in JavaScript:
var vm = new Vue({ el: '#app', data: { message: 'Hello Vue!' } })
When executing this code, Vue parses the instructions in the template, marks the message property as a reactive property, and then uses the Object.defineProperty method to set its getter and The setter method is hijacked to automatically update the corresponding view when the message attribute changes.
- Template compilation
In Vue.js, when a Vue instance is created, Vue will parse the instructions in the template and generate a DOM rendering function, and then pass this DOM Render function to generate real DOM elements.
The template in Vue.js is actually HTML code. Vue can parse the HTML code into an AST abstract syntax tree, generate the corresponding vnode through static analysis of the abstract syntax tree, and then generate DOM rendering based on the vnode. function.
For example, in the template code mentioned above, Vue will parse it into the following abstract syntax tree:
{ type: 'element', tag: 'div', attrsList: [], attrsMap: {}, children: [ { type: 'element', tag: 'label', attrsList: [], attrsMap: {}, children: [ { type: 'text', text: '输入内容:' } ] }, { type: 'element', tag: 'input', attrsList: [ { name: 'v-model', value: 'message' } ], attrsMap: { 'v-model': 'message' }, children: [] }, { type: 'element', tag: 'p', attrsList: [], attrsMap: {}, children: [ { type: 'text', text: '输出内容:' }, { type: 'expression', text: '_s(message)', tokens: [ { '@binding': 'message' } ] } ] } ] }
The v-model directive binds the message attribute to the value attribute of the input element. is determined, and {{ message }} binds the message attribute to the textContent attribute of the p element.
- Implementation of components
In Vue, components are one of the important concepts. Components allow us to split the application into small, reusable parts, which can effectively improve the reusability and maintainability of the code.
The implementation of components is also inseparable from the data binding mechanism. Inside the component, we can use the props provided by Vue to define the properties of the component, and then use these properties inside the component to achieve two-way binding of data.
For example, we define a simple component:
Vue.component('my-component', { props: ['title'], template: '<h1>{{ title }}</h1>' })
Then use this component in the template:
<my-component title="Hello Vue!"></my-component>
In the template, we pass in an attribute title to the component, Inside the component, the title attribute is defined and used through props.
Summary:
The mechanism of Vue.js to implement data binding is to use responsive objects, template compilation and component implementation. By defining responsive objects and hijacking getter and setter methods, and parsing templates to generate DOM rendering functions, automatic data updates are achieved. Inside the component, we define the properties of the component through props and use these properties to implement two-way binding of data. This series of mechanisms makes Vue.js a modern JavaScript framework and provides developers with a good development experience and convenience.
The above is the detailed content of Let's talk about the implementation mechanism of Vue data binding. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
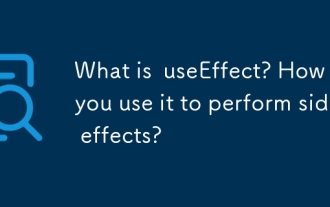
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
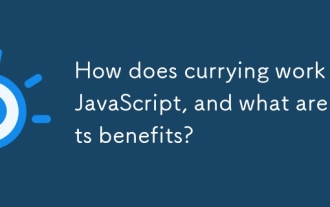
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
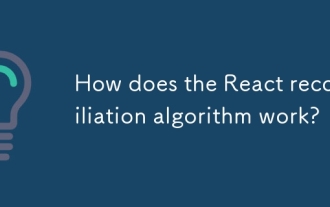
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
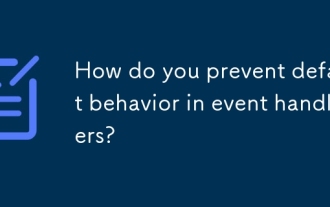
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
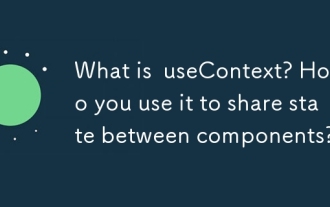
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
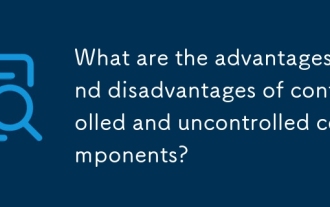
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
