How to delete fixed subscript string in JAVA
需要修改的报文
当你拿到的报文是这样的
{ "input": { "sdfsn": "23u4209350-2", "fsfs": "128412094", "sgsgsg": "15821059", "inssgsuplc_admdfdfdvs": "125125332", "dgh": "125215312", "dfgdfg": "215215", "sdhdsh": "", "sdfsn": "", "shdfshdshdsh": "shsdh", "sdhdsh": "shsh.0", "shsdhsd": "1", "shsdh": "1607", "input": { "data": { "dhfsdhsd": "235325", "shsdhsdh": "03", "dgd": "BE0445360", "dfhfdh": "11", "dshshsd": 76.56, "ghjrfgj": "01", "grjf": "234623626", "hjfd": "236436", "djfdfgjdfj": "45634", "exp_Content": "{"gdsg":"01","gjfj":"658568","fjfj":"5675467","ghfjfgkj":"68568","vmgfvj":"658568","gfhjgfyk":"0","fghkfghkg":"5474567"}", "dfjgdfj": "", "dfjdfjgdfj": "56745745", "dfgjdfgjh": 45756758, "jdfgjhfdgj": 0, } } }, "output": { "output": { "r757": { "dhfsdhsd": "235325", "shsdhsdh": "03", "dgd": "BE0445360", "dfhfdh": "11", "dshshsd": 76.56, "ghjrfgj": "01", "grjf": "234623626", "hjfd": "236436", "djfdfgjdfj": "45634", "exp_content": "", "dfjgdfj": "", "dfjdfjgdfj": "56745745", "dfgjdfgjh": 45756758, "jdfgjhfdgj": 0, }, "sdfgsdfg": [ { "sgasgag": "4673476", "agasgdas": 5675467, "asgasgasg": "", "asdgasgas": 4567456754, "dhsdsxchsdh": 54675467, "sdfhsdhsdh": "5674756457" } ] }, "erherth": 0, } }
这一看就知道上边的报文在postman里边肯定会报错,因为exp_Content,因此他又没有用到,所以你想把他删掉。其实也没那么难删
也就是用到了流转字符串。字符串固定字符查找,然后进行字符串转字符流,删掉字符流中固定字符,之后再转回来。因为字符串已经是final了所以很多用法都是使用字符串转字符流实现的
实现代码如下
private JSONObject resolveApplicationJson(HttpServletRequest request) { InputStream is = null; String json = null; try { is = request.getInputStream(); json = IOUtils.toString(is, "UTF-8"); json=json.replaceAll("\\r|\n|\t",""); int index= json.indexOf("exp_Content"); int indexfirst=json.indexOf("{", index); int indexlast=json.indexOf("}",index); if (index!=-1 && indexlast !=-1 &&indexfirst !=-1) { StringBuffer stringBuffer = new StringBuffer(json); stringBuffer.delete(indexfirst,indexlast+1); json=stringBuffer.toString(); } } catch (IOException e) { throw new RuntimeException("CANNOT get reader from request!", e); } finally { if (is != null) { try { is.close(); } catch (IOException e) { e.printStackTrace(); } } } try { return new JSONObject(json); } catch (JSONException e) { throw new RuntimeException("CANOT CONVET JSON:[" + json + "] to JSONObject!", e); } }
多存在多个不符合规定的数据然后你要删掉怎么操作呢?
当你拿到的报文是这样的。
{ "input": { "sdfsn": "23u4209350-2", "fsfs": "128412094", "sgsgsg": "15821059", "inssgsuplc_admdfdfdvs": "125125332", "dgh": "125215312", "dfgdfg": "215215", "sdhdsh": "", "sdfsn": "", "shdfshdshdsh": "shsdh", "sdhdsh": "shsh.0", "shsdhsd": "1", "shsdh": "1607", "input": { "data": { "dhfsdhsd": "235325", "shsdhsdh": "03", "dgd": "BE0445360", "dfhfdh": "11", "dshshsd": 76.56, "ghjrfgj": "01", "grjf": "234623626", "hjfd": "236436", "djfdfgjdfj": "45634", "exp_Content": "{"gdsg":"01","gjfj":"658568","fjfj":"5675467","ghfjfgkj":"68568","vmgfvj":"658568","gfhjgfyk":"0","fghkfghkg":"5474567"}", "dfjgdfj": "", "dfjdfjgdfj": "56745745", "dfgjdfgjh": 45756758, "jdfgjhfdgj": 0, } } }, "output": { "output": { "r757": { "dhfsdhsd": "235325", "shsdhsdh": "03", "dgd": "BE0445360", "exp_Content": "{"gdsg":"01","gjfj":"658568","fjfj":"5675467","ghfjfgkj":"68568","vmgfvj":"658568","gfhjgfyk":"0","fghkfghkg":"5474567"}", "dfhfdh": "11", "dshshsd": 76.56, "ghjrfgj": "01", "grjf": "234623626", "hjfd": "236436", "djfdfgjdfj": "45634", "exp_content": "{"gdsg":"01","gjfj":"658568","fjfj":"5675467","ghfjfgkj":"68568","vmgfvj":"658568","gfhjgfyk":"0","fghkfghkg":"5474567"}", "dfjgdfj": "", "dfjdfjgdfj": "56745745", "dfgjdfgjh": 45756758, "jdfgjhfdgj": 0, }, "sdfgsdfg": [ { "sgasgag": "4673476", "agasgdas": 5675467, "asgasgasg": "", "asdgasgas": 4567456754, "dhsdsxchsdh": 54675467, "sdfhsdhsdh": "5674756457" } ] }, "erherth": 0, } }
解决方式如下:根据需要写出正则表达式,再使用正则看看有几个这样的不需要的符号,然后循环遍历几次,之后找到这部分,最后删除掉。
实现代码如下
# 全局变量 private static String REGEX = "exp_[c,C]ontent"; # 方式方法 Pattern p = Pattern.compile(REGEX); // 获取 matcher 对象 Matcher m = p.matcher(JSON); List<Integer> list = new ArrayList(); while(m.find()){ list.add(m.start()); } int index=0; int indexfirst=0; int indexlast=0; for (int j = 0; j < list.size(); j++) { index=JSON.indexOf("exp_",indexlast); indexfirst=JSON.indexOf("{", index); indexlast=JSON.indexOf("}",index); StringBuffer stringBuffer =null; if (indexlast !=-1 &&indexfirst !=-1) { stringBuffer = new StringBuffer(JSON); stringBuffer.delete(indexfirst,indexlast+1); } JSON=stringBuffer.toString(); }
The above is the detailed content of How to delete fixed subscript string in JAVA. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
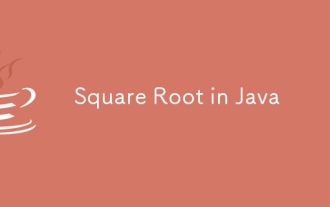
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
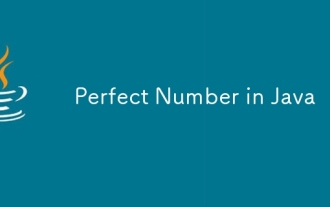
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
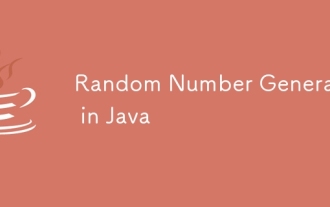
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
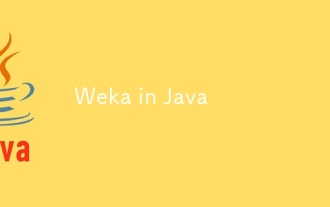
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
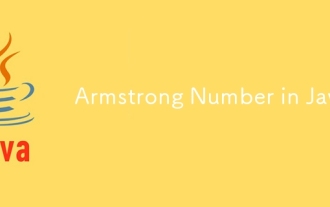
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
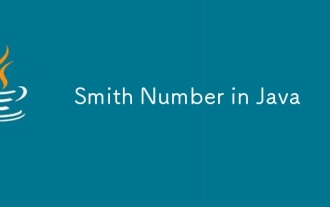
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
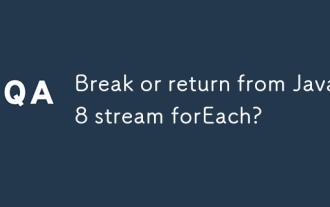
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
