Why doesn't golang escape strings?
In modern programming languages, strings are often escaped to ensure that special characters and symbols can be correctly represented in the program. However, Golang provides a very convenient way to avoid this escaping. This feature can be very practical in some scenarios, especially when we need to process text in certain formats. Next, we will explore this feature of unescaped strings in Golang.
In Golang, not escaping a string can be achieved by adding a backtick
symbol before the string. This means that you can include arbitrary characters and symbols in the string without additional escaping. This is very useful when dealing with some complex formatted text.
Let us illustrate this feature with a specific example. Suppose we need to process an HTML file that contains some JavaScript code and we need to process a specific part of it. An example of this HTML file is as follows:
<!DOCTYPE html> <html> <head> <title>Example</title> <script> var hello = "Hello, World!"; // 需要进行处理的部分 alert(hello); </script> </head> <body> </body> </html>
We can easily extract this JavaScript code through Golang’s unescaped string feature. The code example is as follows:
package main import ( "fmt" "strings" ) func main() { html := ` <!DOCTYPE html> <html> <head> <title>Example</title> <script> var hello = "Hello, World!"; // 需要进行处理的部分 alert(hello); </script> </head> <body> </body> </html> ` start := strings.Index(html, "<script>") end := strings.Index(html, "</script>") js := html[start:end] fmt.Println(js) }
As shown in the above code, we use a backtick symbol to define a string containing the entire HTML file, and then use the function provided by the strings
package to Extract the JavaScript code inside. This method is very convenient and the code is more readable and maintainable.
Of course, there are some details to pay attention to when using unescaped strings. For example, the backtick symbol cannot appear again within the content of a string, otherwise the compiler will not be able to parse the string correctly. In addition, the length of the string may also change, since any characters in it do not need to be escaped, which will also affect the length calculation of the string.
After all these considerations, using Golang's unescaped string feature can make it more convenient for us to handle strings containing text in specific formats without being troubled by complex escape symbols. Especially in some processing scenarios that have strict requirements on string format, we can use this feature to complete the task more efficiently.
The above is the detailed content of Why doesn't golang escape strings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


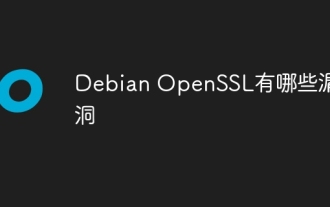
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
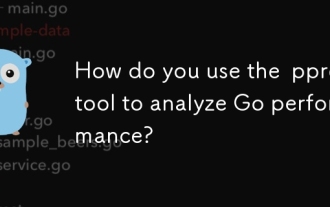
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
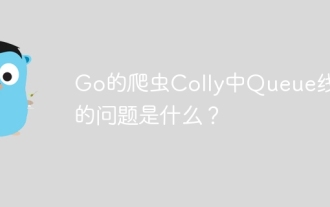
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
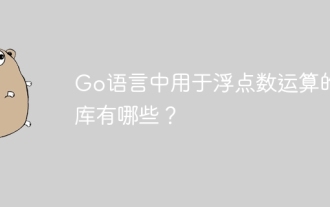
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
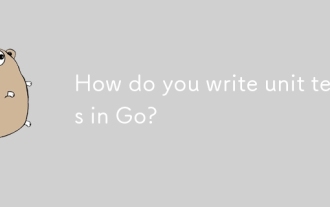
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
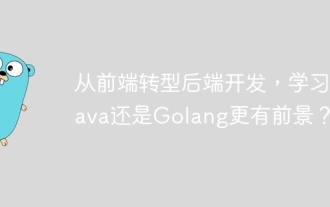
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
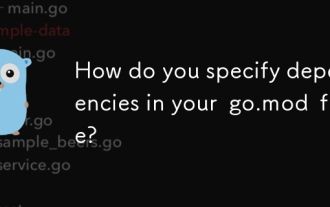
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
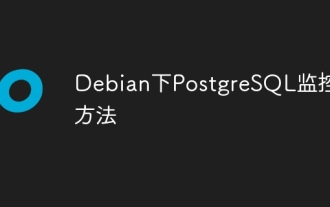
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
