How to use Golang and Kafka together
Kafka is an open source distributed message queue that is often used to build real-time data stream processing applications in big data applications. Golang is a programming language developed by Google and is known for its efficient concurrency, powerful libraries and ecosystem. So, how to use Golang to combine with Kafka?
First, we need to import the github.com/Shopify/sarama package. This is a Golang client library that supports Kafka. During the installation process, you need to run the following command:
go get github.com/Shopify/sarama
Next, we need to create a producer. First, create the configuration:
config := sarama.NewConfig() config.Producer.RequiredAcks = sarama.WaitForAll config.Producer.Retry.Max = 5 config.Producer.Return.Successes = true
Here we set the producer to wait for all ACKs, try up to 5 retries, and return a success message to the producer after success.
Next, we need to create a producer instance:
producer, err := sarama.NewSyncProducer([]string{"localhost:9092"}, config) if err != nil { panic(err) } defer producer.Close()
We need to specify a Kafka broker address as the service endpoint to connect to Kafka. Here we are connecting to the local Kafka server. We also call the .Close()
method to ensure cleanup when the producer exits.
Now we are ready to start publishing messages to the Kafka topic:
msg := &sarama.ProducerMessage{ Topic: "test", Value: sarama.StringEncoder("Hello World!"), } part, offset, err := producer.SendMessage(msg) if err != nil { fmt.Printf("Error publishing message: %v", err) } else { fmt.Printf("Message published successfully. Partition: %v, Offset: %v\n", part, offset) }
In this example, we publish a message to the topic named "test". If there are no errors, it prints out the successfully published partition and offset.
Now we have created a producer that publishes a message to Kafka. Next, let's take a look at how to create a consumer.
First, we need to create the consumer configuration:
config := sarama.NewConfig() config.Consumer.Return.Errors = true
Here we set the receiving error.
Next, we need to create a consumer instance:
consumer, err := sarama.NewConsumer([]string{"localhost:9092"}, config) if err != nil { panic(err) } defer consumer.Close()
Here we also specify a Kafka broker address. We also need to call the .Close()
method to ensure that the consumer will clean up when it exits.
Now we are ready to read messages from the Kafka topic:
partitionConsumer, err := consumer.ConsumePartition("test", 0, sarama.OffsetOldest) if err != nil { panic(err) } defer partitionConsumer.Close() for { select { case msg := <-partitionConsumer.Messages(): fmt.Printf("Received message from partition %d with offset %d: %s = %s\n", msg.Partition, msg.Offset, string(msg.Key), string(msg.Value)) case err := <-partitionConsumer.Errors(): fmt.Println("Error: ", err.Error()) } }
In this example, we subscribe to the topic named "test". Then we read the offset of the first partition. We then read messages from that partition infinitely in a loop. The select
statement in the loop will always monitor the message and error channels and print them respectively.
So far, we have introduced how to use Golang and Kafka to combine. With this simple example, you should have mastered the basic usage of Golang and Kafka.
The above is the detailed content of How to use Golang and Kafka together. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
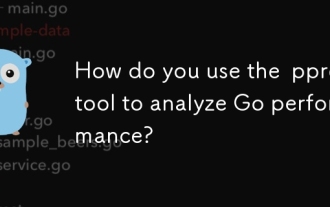
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
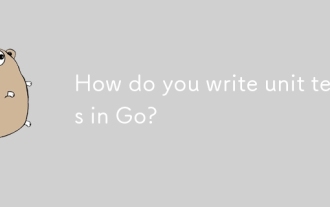
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
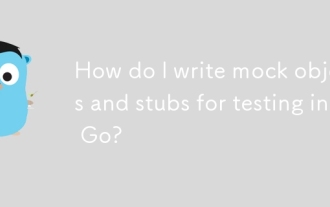
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
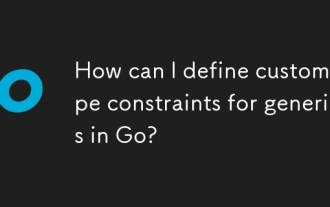
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
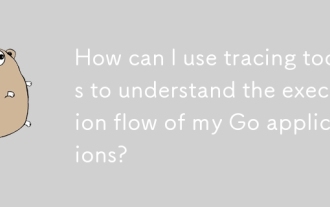
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
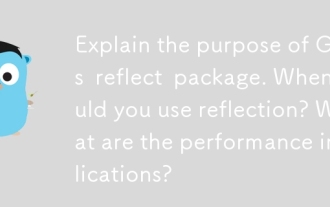
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
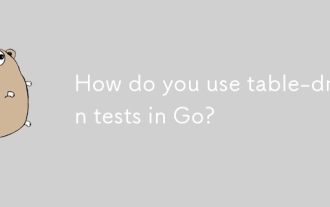
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
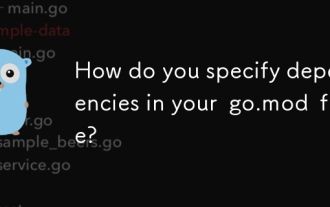
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
