How to pass variables in thinkphp controller
thinkphp is a very popular PHP development framework. It has the advantages of simplicity, ease of use, powerful functions, and excellent performance. It has been widely used in the development of many Web applications. In the thinkphp framework, the controller is the core part responsible for processing user requests. It implements data extraction and processing through the controller, and finally returns the results to the user. In this process, how the controller transfers and processes variables is a very critical issue. This article will delve into how to transfer variables in the thinkphp controller and how to ensure the correctness and efficiency of the transfer process.
1. Variable transfer method
- GET method
The controller can obtain the parameters passed by the client through the GET method. Pass parameters in GET mode and put the parameters in the URL, such as http://www.example.com/index.php?Parameter name=Parameter value. Use the GET method to pass parameters. The parameters will be exposed in the URL, so it is not suitable for passing sensitive data. You need to be careful when using it.
To obtain the parameters passed by GET method, you can use the input method provided by the thinkphp framework to receive the parameters passed by get method in the URL address. For example:
$id = input('id')
- POST method
Use POST method to pass parameters. The parameters will not appear in the URL, so it is suitable for transmitting sensitive data, such as the user's account password, bank Card number, etc. Use the POST method to pass parameters, and you can also use the input method to receive them in the controller. For example:
$name = input('post.name');
- Routing method
The routing method is more flexible in passing parameters. You can customize the URL address and put the parameters in the URL. In the routing configuration of the thinkphp framework, :id can be passed to the controller as a parameter in the form of 'router' => ['/:id' => 'index/hello']
. For example:
Routing configuration:
'router' => [ '/user/:id' => 'User/index' ],
Obtaining parameters in the controller:
public function index($id) { echo 'User ID:' . $id; }
2. How to avoid the error of not passing variables?
- Add default values to parameters
In the controller of the thinkphp framework, we can set a default value for each parameter that receives a variable to ensure that even if it is not passed There will be no errors with the parameters. For example:
public function test($id='',$name='',$age='') { echo $id,$name,$age; }
- Determine whether the variable exists
In the controller, we can use the isset() function to determine whether the parameter is passed:
if(isset($_REQUEST['id'])) { $id=$_REQUEST['id']; } else { $id=0; }
But in the thinkphp framework, we recommend using the has() method of the request class to determine whether parameters are passed. For example:
if(request()->has('id')) { $id=request()->param('id'); } else { $id=0; }
3. Think about the efficiency of controller variable transfer?
The transfer of variables will also affect the operating efficiency of the controller. Passing variables requires memory space overhead, and the operating efficiency of the controller directly affects the response speed of the entire system. Therefore, we need to make passing variables as efficient as possible.
- Use static variables
You can use static variables to store frequently used variables to reduce the time spent on passing the same variables. For example:
class OrderController extends Controller { protected static $userId; public function initialize() { self::$userId = input('userId'); } public function index() { // 利用self::$userId使用静态变量 } }
- Use global variables
You can use global variables among variables that you want to use frequently, and different controllers can use them. Using global variables does not require variable parameter passing and can also reduce memory overhead. For example:
$GLOBALS['userId'] = input('userId'); class OrderController extends Controller { public function index() { echo $GLOBALS['userId']; } } class UserController extends Controller { public function index() { echo $GLOBALS['userId']; } }
- Use parameter binding
Through parameter binding, the parameters are directly bound to the function parameters, which reduces the process of variable transfer and improves the program operating efficiency. For example:
class OrderController extends Controller { public function index($userId) { // 直接使用 $userId,避免了变量传递 } }
In general, passing variables correctly and efficiently in the thinkphp framework can speed up the running speed of the program and reduce resource usage. Using the above delivery methods and techniques, we can better improve code quality and develop better web applications.
The above is the detailed content of How to pass variables in thinkphp controller. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


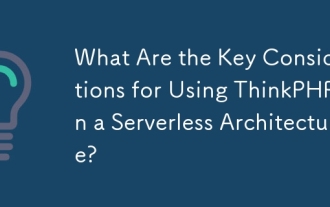
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
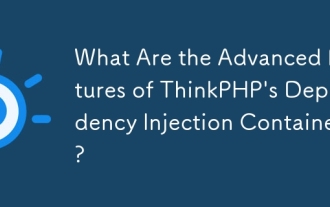
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
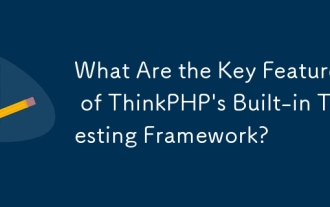
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
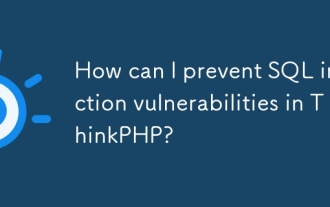
The article discusses preventing SQL injection vulnerabilities in ThinkPHP through parameterized queries, avoiding raw SQL, using ORM, regular updates, and proper error handling. It also covers best practices for securing database queries and validat
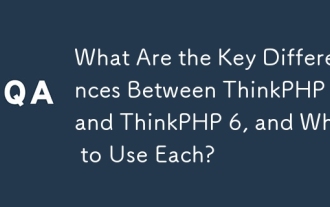
The article discusses key differences between ThinkPHP 5 and 6, focusing on architecture, features, performance, and suitability for legacy upgrades. ThinkPHP 5 is recommended for traditional projects and legacy systems, while ThinkPHP 6 suits new pr
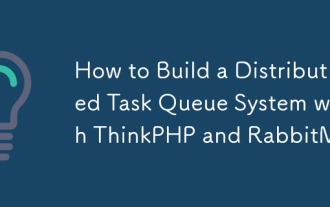
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
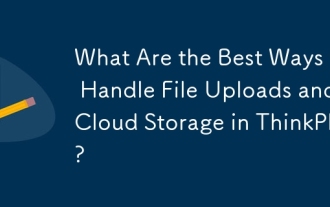
The article discusses best practices for handling file uploads and integrating cloud storage in ThinkPHP, focusing on security, efficiency, and scalability.
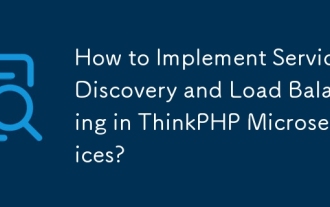
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
