How does nodejs request tomcat
Node.js and Tomcat are two different servers, suitable for front-end and back-end development respectively. Front-end developers often use Node.js to build applications and websites, and back-end developers often use Tomcat to build Java web applications. There is no direct connection between the two servers, so some special setup is required when requesting Tomcat via Node.js.
First, to use Node.js to request Tomcat, you need to use the http module. In Node.js, the http module provides core functionality for building HTTP server and client applications. Therefore, before using Node.js to request Tomcat, you need to ensure that the http module has been installed.
Secondly, in order for Node.js to connect to Tomcat, CORS needs to be enabled in Tomcat's configuration file. CORS (Cross-Origin Resource Sharing) is a mechanism that allows web applications in one domain to use resources in another domain. On the Tomcat server, the method of configuring CORS is very simple. You only need to add the following content to Tomcat's web.xml file:
<filter> <filter-name>CorsFilter</filter-name> <filter-class>org.apache.catalina.filters.CorsFilter</filter-class> <init-param> <param-name>cors.allowed.origins</param-name> <param-value>*</param-value> </init-param> <init-param> <param-name>cors.allowed.methods</param-name> <param-value>GET, POST, HEAD, OPTIONS, PUT, DELETE, PATCH</param-value> </init-param> </filter> <filter-mapping> <filter-name>CorsFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
The above code will allow HTTP requests from any source, and supports GET, POST, HEAD, OPTIONS, PUT, DELETE and PATCH methods.
After the configuration is completed, you can use Node.js to send requests to Tomcat. The following is a code example of Node.js sending a GET request:
const http = require('http'); const options = { hostname: 'localhost', port: 8080, path: '/your/tomcat/path', method: 'GET' } const req = http.request(options, res => { console.log(`statusCode: ${res.statusCode}`) res.on('data', d => { process.stdout.write(d) }) }) req.on('error', error => { console.error(error) }) req.end()
In the above code, the options object contains the details of the request, including Tomcat's address and port number, the requested path and the requested method (GET, POST etc.). The req object is returned from the http.request() function and can be used to send requests to Tomcat. The result of the request is passed through the callback function res and output to the console using process.stdout.write().
In addition to GET requests, you can also use Node.js to send POST requests. The following is a code example for Node.js to send a POST request:
const http = require('http'); const data = JSON.stringify({ message: 'Hello, Tomcat!' }) const options = { hostname: 'localhost', port: 8080, path: '/your/tomcat/path', method: 'POST', headers: { 'Content-Type': 'application/json', 'Content-Length': data.length } } const req = http.request(options, res => { console.log(`statusCode: ${res.statusCode}`) res.on('data', d => { process.stdout.write(d) }) }) req.on('error', error => { console.error(error) }) req.write(data) req.end()
In the above code, the data object contains the data that needs to be sent, and is serialized using JSON.stringify(). In addition to the same properties as the GET request, the options object also includes request headers (Content-Type and Content-Length) to indicate the type and length of the data being sent. Like GET requests, the results of POST requests are passed through the res callback function.
The above is the basic method of using Node.js to request Tomcat, and these sample codes can be adapted to most situations. However, it needs to be modified or added according to the specific situation when using it.
The above is the detailed content of How does nodejs request tomcat. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics





React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
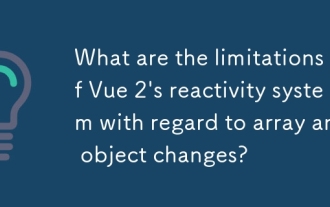
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
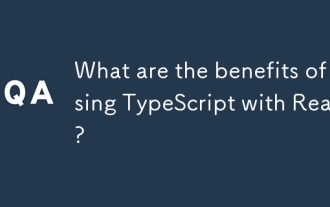
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
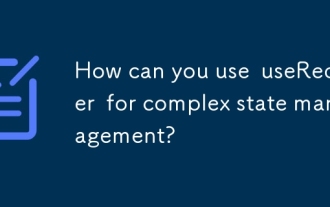
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
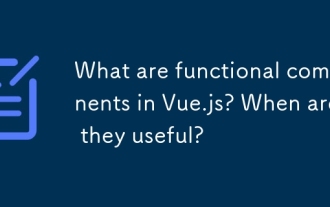
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a
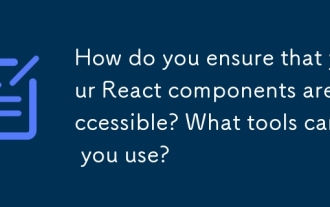
The article discusses strategies and tools for ensuring React components are accessible, focusing on semantic HTML, ARIA attributes, keyboard navigation, and color contrast. It recommends using tools like eslint-plugin-jsx-a11y and axe-core for testi
