How to use jquery to implement map positioning
With the popularization of mobile Internet, map positioning has become an increasingly popular service and a basic function of many online and mobile applications. As a commonly used JavaScript library, jquery also provides a rich map positioning API. Let's introduce how to use jquery to implement map positioning.
1. Introduction of jquery library and map API
Before using jquery for map positioning, we need to introduce jquery library and map API into the page. Under normal circumstances, we can import it directly from the Internet:
<script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script> <script src="https://webapi.amap.com/maps?v=1.4.15&key=您申请的key值"></script>
In the above code, we introduced the jquery library and Amap API, and used a key value applied by ourselves for identity verification.
2. Create a map container
Create a container for displaying the map on the page, which can be a div, canvas and other tags, for example:
<div id="mapContainer" style="width: 100%; height: 300px;"></div>
In the above code, We created a div tag with the id "mapContainer" to display the map, and set the width and height.
3. Initialize the map
After completing the creation of the map container, we need to use jquery to initialize the map. The code is as follows:
var map = new AMap.Map("mapContainer", { resizeEnable: true, zoom: 10, //设置地图缩放级别 center: [116.397428, 39.90923] //设置地图中心点坐标 });
In the above code, we use AMap. The Map() function initializes the map and performs some configurations, such as setting the zoom level, center point coordinates, etc.
4. Obtain the current location through the browser
After completing the initialization of the map, we need to obtain the location information of the current device, which can be achieved through the API provided by the browser. The code is as follows:
if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function (position) { var longitude = position.coords.longitude; //获取经度 var latitude = position.coords.latitude; //获取纬度 var accuracy = position.coords.accuracy; //获取精度 map.setZoomAndCenter(18, [longitude, latitude]); //设置地图缩放级别和中心坐标 var marker = new AMap.Marker({ position: [longitude, latitude], //设置标记的坐标 map: map //设置标记所属的地图对象 }); },function (error) { switch (error.code) { case 1: console.log("位置服务被拒绝"); break; case 2: console.log("暂时获取不到位置信息"); break; case 3: console.log("获取信息超时"); break; case 4: console.log("未知错误"); break; } }); }
In the above code, we determine whether the browser supports obtaining geographical location information. If supported, we obtain the longitude, latitude, accuracy and other information of the current location through the getCurrentPosition() function, and set the center coordinates and markers of the map through the setZoomAndCenter() function and AMap.Marker() function.
5. The complete code for map positioning
The following is the complete code for jquery to implement map positioning:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>jquery实现地图定位</title> <style type="text/css"> #mapContainer{ width: 100%; height: 300px; } </style> </head> <body> <div id="mapContainer"></div> <script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script> <script src="https://webapi.amap.com/maps?v=1.4.15&key=您申请的key值"></script> <script> $(function () { //初始化地图 var map = new AMap.Map("mapContainer", { resizeEnable: true, zoom: 10, center: [116.397428, 39.90923] }); //获取当前位置 if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function (position) { var longitude = position.coords.longitude; //获取经度 var latitude = position.coords.latitude; //获取纬度 var accuracy = position.coords.accuracy; //获取精度 map.setZoomAndCenter(18, [longitude, latitude]); //设置地图缩放级别和中心坐标 var marker = new AMap.Marker({ position: [longitude, latitude], map: map }); },function (error) { switch (error.code) { case 1: console.log("位置服务被拒绝"); break; case 2: console.log("暂时获取不到位置信息"); break; case 3: console.log("获取信息超时"); break; case 4: console.log("未知错误"); break; } }); } }); </script> </body> </html>
6. Conclusion
This article introduces how to use The method and code for implementing map positioning in jquery, I hope it will be helpful to everyone in your study or work. Of course, the map positioning API provided by jquery is far more than this. It has more and richer functions. You can learn more about it by viewing the jquery official documentation.
The above is the detailed content of How to use jquery to implement map positioning. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
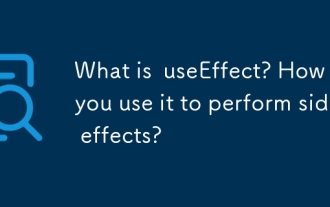
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
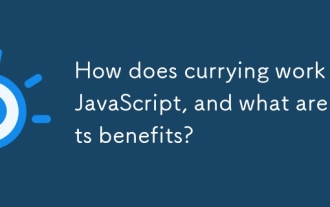
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
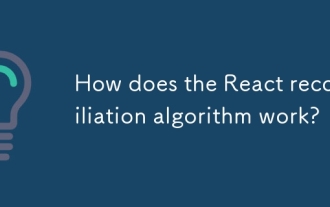
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
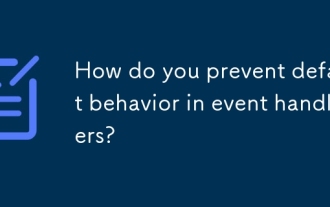
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
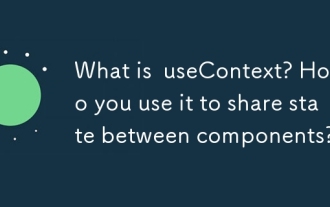
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
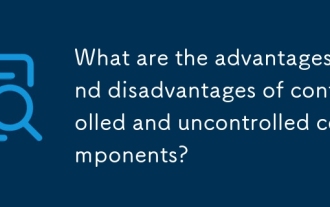
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
