Explore how to use Node.js to query the database
In recent years, with the continuous development of Internet technology, more and more companies have begun to transform to the Internet. As a hot topic in Internet technology, Node.js has always attracted much attention. Node.js is a JavaScript running environment based on the Chrome V8 engine. It uses an event-driven, non-blocking I/O model to gain the advantages of high performance and scalability.
In Node.js application development, it is often necessary to process the database. In the process of using the database, process management is particularly important. This article will explore how to use Node.js to query the database process.
1. Node.js and database connection
Before using Node.js to query the database, you need to install the corresponding database driver package. For example, to connect to a MySQL database, you can use the mysql driver package. After installing the mysql driver package, we need to use the following code to establish a connection with the MySQL database:
var mysql = require('mysql'); var connection = mysql.createConnection({ host : 'localhost', user : 'root', password : '', database : 'test' }); connection.connect();
In the above code, we use the mysql module to create a connection with the MySQL database named test . Among them, host represents the IP address of the host where the database is located, user represents the username to connect to the database, password represents the password to connect to the database, and database represents the name of the connected database. The last line of code uses connect() to establish the connection. If the connection is successful, we can use the connection object to operate the database.
2. Query the data in the MySQL database
Before using Node.js to query the MySQL database, we need to first understand the SELECT statement in MySQL. The SELECT statement is used to query data from the database. Its basic syntax is:
SELECT column_name,column_name FROM table_name WHERE column_name operator value
Among them, column_name represents the name of the column to be queried, table_name represents the name of the table to be queried, and the WHERE keyword is used to set query conditions. operator The operator represents the query condition, and value represents the value of the query condition.
When using Node.js to query the MySQL database, we need to first create a SELECT statement. The following is a sample code that queries all data in the test table:
connection.query('SELECT * FROM test', function(error, results, fields) { if (error) throw error; console.log('The solution is: ', results[0].solution); });
In the above code, we use the query() method of the connection object. The query() method accepts two parameters: one is the SELECT statement to be executed, and the other is the callback function. The callback function contains three parameters: error, results and fields. Among them, error represents the error information that occurred during the query process, results represents the query result set, and fields represents the field information of the query results. If the query is successful, we can use the results parameter to obtain the query results. For example, you can use the following code to output the solution column of the first element in the query result set:
console.log('The solution is: ', results[0].solution);
3. Query the data in the MongoDB database
Before using Node.js to query the MongoDB database , you need to install the MongoDB database driver package mongoose. After installing the mongoose driver package, we need to use the following code to establish a connection with the MongoDB database:
var mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/test', {useNewUrlParser: true});
In the above code, we use the mongoose module to establish a connection with the MongoDB named test through the connect() method. Database connection. Among them, 'mongodb://localhost/test' represents the connection address of the MongoDB database, which is similar to the parameters when connecting to the MySQL database.
To query data in the MongoDB database, we need to first understand the find() method in MongoDB. The find() method is used to query data from the database. Its basic syntax is:
db.collection.find(query, projection)
Among them, db.collection represents the target collection of the query, query represents the query condition, and projection represents the field to be returned. If the query condition is empty, all data in the collection will be returned. The following is a sample code that queries all data in the test collection:
var db = mongoose.connection; db.on('error', console.error.bind(console, 'connection error:')); db.once('open', function() { console.log("MongoDB连接成功!"); var kittySchema = new mongoose.Schema({ name: String }); var Kitten = mongoose.model('Kitten', kittySchema); Kitten.find(function (err, kittens) { if (err) console.error(err); console.log(kittens); }); });
In the above code, we define a schema object named kittySchema to specify the document structure of the MongoDB collection test. Then, we use the mongoose.model() method to create a Kitten model to operate the collection in MongoDB. Finally, use the Kitten.find() method to query all data in the test collection. If the query is successful, the corresponding results can be returned.
4. Summary
For Node.js application development, frequent operations on the database are required. When querying the database, we can use Node.js driver packages and modules to make data query more efficient, fast and accurate. This article introduces the method of querying MySQL and MongoDB databases with Node.js. I hope it will be helpful to your development work.
The above is the detailed content of Explore how to use Node.js to query the database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


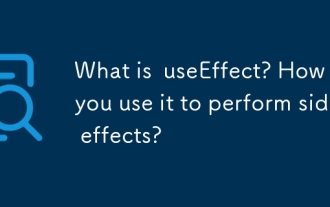
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
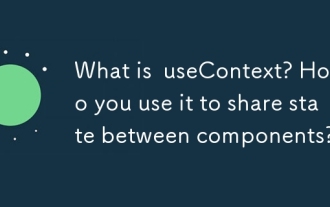
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
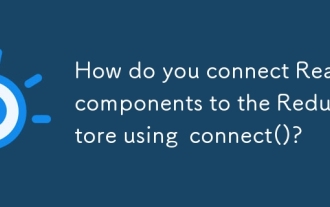
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
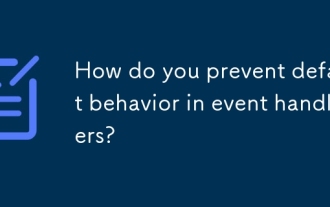
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
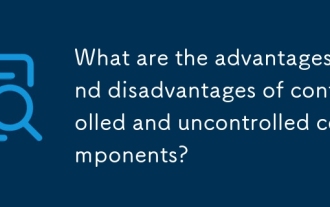
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
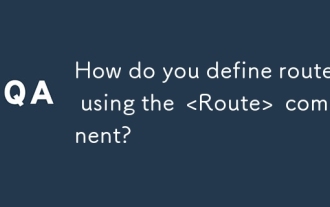
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
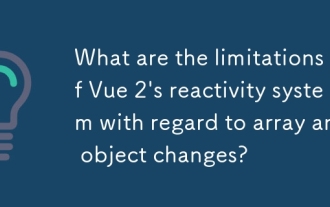
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
