How to detect if an array has been assigned a value in php
In PHP programming, arrays are often used to store a series of data, but in some cases, we need to check whether the array has been assigned a value. In this article, we will introduce how to detect whether an array has been assigned a value, as well as some methods of detecting whether an array is empty.
1. PHP array
In PHP, array is a basic data structure. An array is an ordered collection of data consisting of one or more key-value pairs. For example, the following code creates an array with three elements:
$fruits = array("apple", "orange", "banana");
In PHP, arrays can be defined in two forms: numeric arrays and associative arrays.
Numeric arrays are arrays whose elements are accessed through integer indexes. For example, the following code creates a numeric array:
$numbers = array(1, 2, 3, 4);
An associative array is an array whose elements are accessed by specifying a string key. For example, the following code creates an associative array:
$person = array("name" => "John", "age" => 30, "gender" => "male");
2. Determine whether the PHP array has been assigned a value
In PHP, to detect whether the array has been assigned a value, we can use isset() function. The isset() function checks whether the variable has been set and is not null or empty. Here is an example:
$fruits = array(); if(isset($fruits)){ echo "数组已赋值。"; } else { echo "数组未赋值。"; }
If the $fruits array has been assigned, the above code will output "Array has been assigned.", otherwise it will output "Array has not been assigned.". In the above code, the isset() function returns true to indicate that the array has been assigned a value, and false to indicate that the array has not been assigned a value.
If you want to check whether an element in the array exists, we can use the array_key_exists() function. The array_key_exists() function accepts two parameters: the key to search and the array to search. Here is an example:
$fruits = array("apple", "orange", "banana"); if(array_key_exists(1, $fruits)){ echo "数组中存在第二个元素。"; } else { echo "数组中不存在第二个元素。"; }
The above code checks whether the second element in the $fruits array exists. If it exists, it will output "The second element exists in the array.", otherwise it will output "The second element does not exist in the array.".
3. Determine whether the PHP array is empty
In PHP, there are several ways to check whether the array is empty.
The first method is to use the empty() function. The empty() function checks whether a variable is empty, including empty string, empty array, 0, false, etc. Here is an example:
$fruits = array(); if(empty($fruits)){ echo "数组为空。"; } else { echo "数组不为空。"; }
The above code will output "Array is empty." because the $fruits array has not been assigned a value.
The second method is to use the count() function. The count() function is used to get the number of elements in an array. If there are no elements in the array, the count() function returns 0. Here is an example:
$fruits = array(); if(count($fruits) == 0){ echo "数组为空。"; } else { echo "数组不为空。"; }
The above code will output "Array is empty." because the $fruits array has not been assigned a value.
The third method is to use the sizeof() function. The sizeof() function has the same function as the count() function and is used to get the number of elements in an array. Here is an example:
$fruits = array(); if(sizeof($fruits) == 0){ echo "数组为空。"; } else { echo "数组不为空。"; }
The above code will output "Array is empty." because the $fruits array has not been assigned a value.
Summary
In PHP programming, arrays are very important data structures. To check whether an array has been assigned a value, use the isset() function. To check if an element in an array exists, you can use the array_key_exists() function. To check if an array is empty, you can use the empty() function, count() function, or sizeof() function. In actual development, we should choose the appropriate method according to specific needs.
The above is the detailed content of How to detect if an array has been assigned a value in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


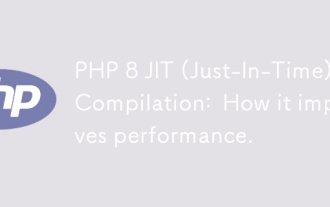
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
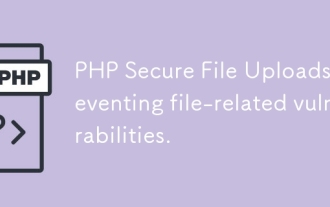
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
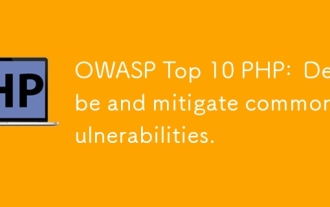
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
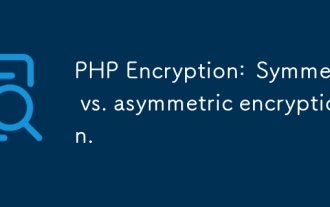
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
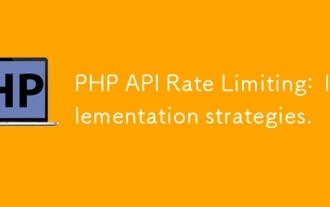
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
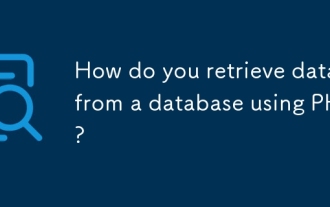
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
