How to compare different elements of an array in php
In PHP, comparing different elements between two arrays is a common need, for example:
- Compare whether their page paths are different between two different versions of the website Part
- Compare different rows between two CSV files
- Compare the table structure between two different databases to see if there are any differences
This article will introduce Several ways to compare different elements of an array in PHP.
Method 1: Use the array_diff function
The array_diff function is a built-in function in PHP that compares array differences. The usage of this function is as follows:
array array_diff ( array $array1 , array $array2 [, array $... ] )
This function accepts two arrays Parameter, returns a new array containing the elements in the first array that do not appear in the second array.
For example, the following code compares the difference between two arrays:
$arr1 = [1, 2, 3, 4, 5]; $arr2 = [4, 5, 6, 7, 8]; $diff = array_diff($arr1, $arr2); print_r($diff); // 输出 [1, 2, 3]
Although the array_diff function can find the different elements of two arrays, if there are duplicate elements in the array, the function may Incorrect results will occur.
For example:
$arr1 = [1, 2, 3, 3, 3, 4, 5]; $arr2 = [3, 4, 5, 5, 5, 6, 7]; $diff = array_diff($arr1, $arr2); print_r($diff); // 输出 [1, 2]
In this example, the result of the $diff array should be [1, 2, 3], but because there are multiple "3" in both the $arr1 and $arr2 arrays ” and “5”, so the array_diff function cannot compare the differences correctly.
Method 2: Use array_diff_assoc function
The array_diff_assoc function is also a PHP built-in function, but this function compares whether the keys and values in the two arrays are equal.
The usage of this function is as follows:
array array_diff_assoc ( array $array1 , array $array2 [, array $... ] )
Accepts two or more array parameters and returns all elements in the first array that do not exist in other arrays or whose keys and values are not equal.
For example:
$arr1 = ["a" => 1, "b" => 2, "c" => 3]; $arr2 = ["a" => 1, "b" => 2, "c" => 4]; $diff = array_diff_assoc($arr1, $arr2); print_r($diff); // 输出 ["c" => 3]
In this example, the result of the $diff array is ["c" => 3], because the value corresponding to the "c" key in the $arr1 array is 3 , there is no such key in the $arr2 array or the value is not equal to 3.
Method 3: Use a custom function
Using a custom function to compare different elements of two arrays is a versatile and customizable method.
For example, the following is a function that compares different elements in two arrays:
function array_diff_custom($arr1, $arr2) { $diff = []; foreach ($arr1 as $key => $value) { if (!in_array($value, $arr2)) { $keyInArr2 = array_search($value, $arr2); if ($keyInArr2 !== false) { unset($arr2[$keyInArr2]); } $diff[$key] = $value; } } foreach ($arr2 as $key => $value) { $diff[$key] = $value; } return $diff; }
This function implements the following functions:
- Traverse all elements in $arr1 The element, if not in $arr2, adds it to the $diff array, otherwise removes it from $arr2.
- Traverse all elements in $arr2 and add them to the $diff array.
For example:
$arr1 = [1, 2, 3, 3, 3, 4, 5]; $arr2 = [3, 4, 5, 5, 5, 6, 7]; $diff = array_diff_custom($arr1, $arr2); print_r($diff); // 输出 [0 => 1, 1 => 2, 2 => 3, 5 => 4, 6 => 5, 7 => 6, 8 => 7]
Because the custom function uses traversal, its efficiency is low, and performance problems may occur when the array is large.
Conclusion
This article introduces three ways to compare different elements of an array in PHP, array_diff function, array_diff_assoc function and custom function. They each have their own advantages and disadvantages. Choosing the appropriate method depends on Application scenarios and specific needs.
The above is the detailed content of How to compare different elements of an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


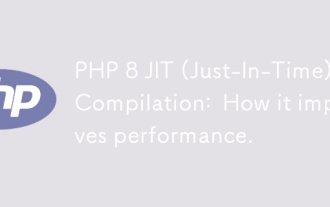
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
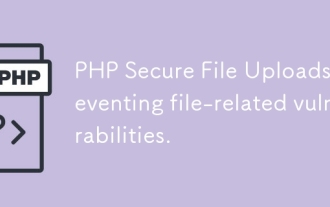
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
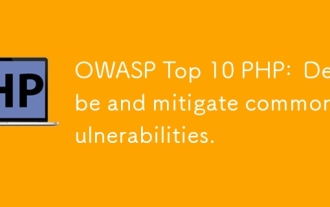
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
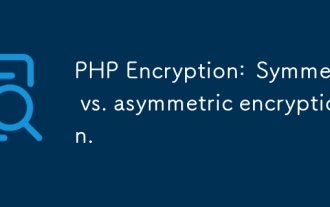
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
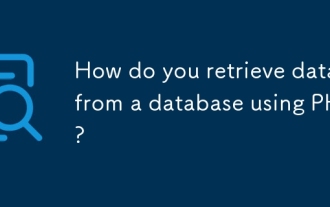
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
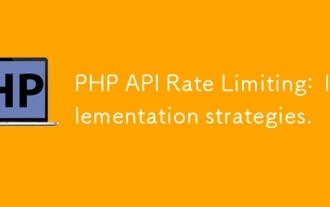
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
