How to remove a key from an array in PHP
In PHP, processing arrays is one of the common tasks. The powerful PHP function library provides various methods to deal with arrays. During development, sometimes you need to eliminate a specific key in an array. This article will introduce how to remove a key from an array in PHP.
What is an array?
An array is a data structure used to store a series of values or elements in a single variable. The elements of an array can be any type of data, including numbers, strings, objects, and other arrays. Each element is accessed through a unique key.
In PHP, array keys can be integers or strings. If the keys are integers, the array is called an indexed array, otherwise it is called an associative array.
The following is a simple PHP array example:
$fruits = array("apple", "banana", "orange", "kiwi");
This array has four elements, each element has a key (0,1,2,3). Array elements can be accessed using these keys:
echo $fruits[0]; // 输出 "apple"
In an associative array, you can use strings as keys. The following is an example of an associative array:
$person = array("姓名" => "张三", "年龄" => 30, "职业" => "程序员");
In this array, "name", "age" and "occupation" are all keys of the associative array. You can use these keys to access the corresponding values:
echo $person["姓名"]; // 输出 "张三"
unset() function in PHP
In PHP, you can use the unset() function to delete elements from an array. However, it should be noted that this function does not return a new array, but eliminates elements in the original array.
unset($fruits[0]); // 删除数组中的第一个元素
In the above example, the unset() function is used to delete the first element of the array $fruits. After this operation, the first element in the $fruits array no longer exists.
Eliminate a key in an array
Deleting an array element may destroy the structure and numeric index of the array. If you wish to remove an element from an array and retain the original array index, you can use the PHP function array_splice() to remove a key.
The following is how to delete a specific key in an associative array:
function remove_key($arr, $key) { if(array_key_exists($key, $arr)) { $keys = array_keys($arr); $index = array_search($key, $keys); if($index !== false) { array_splice($arr, $index, 1); } } return $arr; }
The array_key_exists() function in the above function is used to check whether the specified key exists. If it exists, use the array_keys() function to return all keys of the array, and then use the array_search() function to find the position of the specified key in the key array.
Finally, use the array_splice() function to remove the specified key from the array. This function is used to add or remove elements from an array, keeping the original array index unchanged.
The following is how to delete a specific key in the index array:
function remove_index($arr, $index) { if(isset($arr[$index])) { array_splice($arr, $index, 1); } return $arr; }
The isset() function in the above function is used to check whether the specified index exists. If present, the specified index is removed from the array using the array_splice() function. Unlike the previous function, this function does not need to find the position of the specified key in the key array, because in the index array, the array elements can be accessed directly using the index.
Example
Now, let’s see how to use the above function in practice. Below is an example that demonstrates how to use these functions to remove specified keys from associative and indexed arrays.
// 删除指定键的关联数组元素 $person = array("姓名" => "张三", "年龄" => 30, "职业" => "程序员"); $person = remove_key($person, "职业"); print_r($person); // 输出 Array ( [姓名] => 张三 [年龄] => 30 ) // 删除指定键的索引数组元素 $fruits = array("apple", "banana", "orange", "kiwi"); $fruits = remove_index($fruits, 1); print_r($fruits); // 输出 Array ( [0] => apple [1] => orange [2] => kiwi )
In the above example, we first created an associative array $person and an index array $fruits. Then, we use the remove_key() function to delete the element "occupation" in the $person array, and use the remove_index() function to delete the element "banana" with index 1 in the $fruits array.
Finally, we used the print_r() function to output the modified array.
Conclusion
In this article, we learned how to remove a key from an array in PHP, retaining the original array index. Array elements can be deleted using the unset() function, but this method may destroy the structure and numerical indexing of the array.
If you want to delete an element from an array and retain the original array index, you can use the PHP function array_splice() to delete a key. At the same time, we provide two functions remove_key() and remove_index() for deleting the specified keys in the associative array and index array respectively.
Finally, be careful when using PHP array functions to avoid unpredictable modifications to the array.
The above is the detailed content of How to remove a key from an array in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


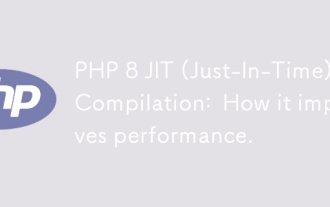
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
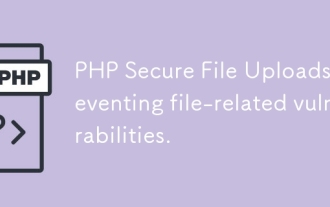
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
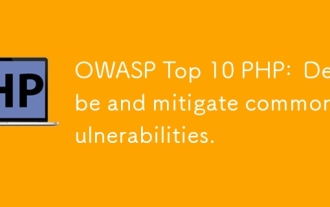
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
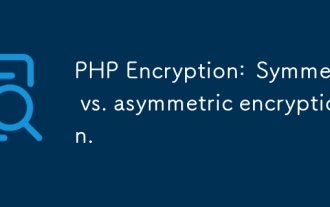
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
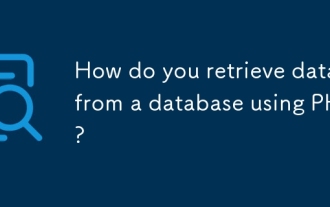
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
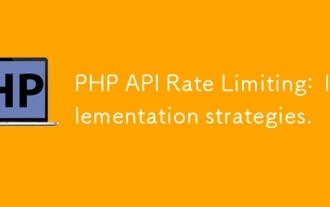
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
