How to sort elements in an array in php
In PHP, for sorting of 2-dimensional arrays, you can use the built-in functions usort()
and uasort()
to achieve it. Both functions can sort elements in an array, but the implementation is slightly different. The
usort()
function will sort the original array and return a Boolean value, while the uasort()
function will sort the original array but retain the key-value pairs The association between them means that no new index array is created. Below we will introduce how to use these two functions respectively.
usort()
Function
usort()
The first parameter of the function is the array that needs to be sorted, and the second parameter is a comparison Function, which is implemented by exchanging the positions of elements in the array that needs to be sorted and returning a Boolean value.
//示例数组$students $students = array( array("name" => "张三", "age" => 22), array("name" => "李四", "age" => 20), array("name" => "王五", "age" => 25) ); //比较函数cmp function cmp($a, $b){ if ($a["age"] == $b["age"]) { return 0; } return ($a["age"] < $b["age"]) ? -1 : 1; } //使用usort()排序 usort($students, "cmp"); //输出结果 var_dump($students);
In the above code, we first define a 2-dimensional array $students containing three elements, each element contains two key-value pairs "name" and "age".
Next, we define a comparison function cmp
, which determines the relative order between elements by comparing the values of two elements on the "age" key-value pair. If the "age" of the two elements is equal, 0 is returned; if the "age" of $a is less than the "age" of $b, then -1 is returned, indicating that $a is in front of $b; otherwise, 1 is returned, indicating that $a After $b.
Finally, we use the usort()
function to sort the array $students according to the rules defined by the cmp
function. From the output, you can see that the elements in the array have been sorted from small to large according to the "age" key-value pair.
uasort()
Function
uasort()
The implementation of the function is similar to usort()
, but different It retains the association between key-value pairs and does not create a new index array. Therefore, it requires a more complex comparison function that not only compares element sizes but also maintains the association of key-value pairs.
//示例数组$students $students = array( "stu1" => array("name" => "张三", "age" => 22), "stu2" => array("name" => "李四", "age" => 20), "stu3" => array("name" => "王五", "age" => 25) ); //比较函数cmp function cmp($a, $b){ if ($a["age"] == $b["age"]) { return 0; } return ($a["age"] < $b["age"]) ? -1 : 1; } //使用usort()排序 uasort($students, "cmp"); //输出结果 var_dump($students);
In the above code, we first define a 2-dimensional associative array $students containing three elements, each element contains two key-value pairs "name" and "age". The difference is that here we use the strings "stu1", "stu2" and "stu3" as the key values of the array instead of the previous numeric index.
The way to define the comparison function cmp is the same as usort()
. The difference is that when we call the uasort()
function, we pass the array $students and the comparison function cmp
as parameters. This function will sort the array according to the cmp
rules, and the sorted result retains the original joint key-value relationship.
Finally, we output the sorting results through the var_dump() function. As you can see, the output result is still an associative array, but the elements have been sorted from small to large according to the "age" key-value pair.
In addition to usort()
and uasort()
, PHP also provides a series of other array sorting functions, such as asort()
, arsort()
, ksort()
, krsort()
, etc. Developers can choose the appropriate function according to actual needs.
The above is the detailed content of How to sort elements in an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
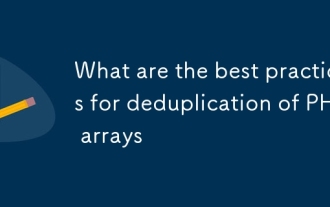
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
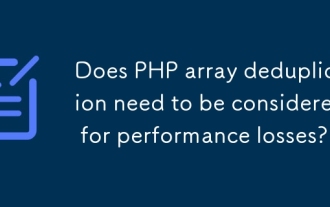
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
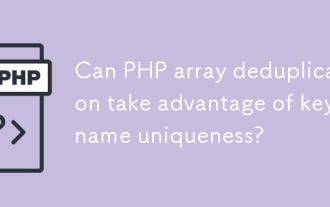
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
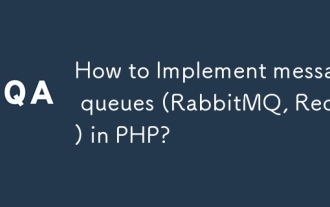
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
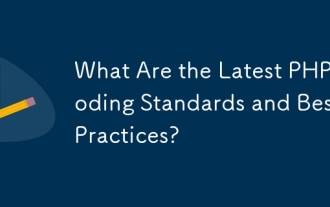
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
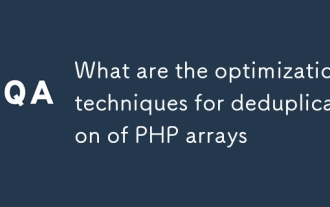
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
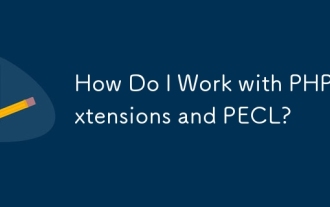
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
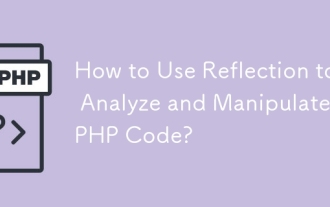
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
