How to call a method in UniApp and get the return value
UniApp is a cross-platform development framework that can develop iOS, Android and Web applications in one code base. Calling methods and getting return values is a common requirement in UniApp. This article will introduce how to call methods in UniApp and get the return value.
Calling methods in UniApp
The process of calling methods in UniApp can be divided into two steps:
- Calling methods in JavaScript code.
- Execute the method in Native code and return the result.
The following is a simple example that demonstrates how to call a Native method in UniApp:
- Call a Native method in JavaScript:
uni.invokeMethod("testPlugin", "testMethod", "args", function(res){ console.log(res); })
-
uni.invokeMethod(plugin, method, args, callback)
The method is used to call Native methods. -
plugin
The parameter refers to the ID of the Native plug-in, such as "testPlugin". -
method
The parameter refers to the name of the Native method, such as "testMethod". -
args
Parameters refer to the parameters required by the method, such as "args". -
callback
The parameter refers to the callback function after the method is executed, where the res parameter represents the return value.
- Asynchronous execution method in Native:
public class TestPlugin implements IModule { @JSMethod(uiThread = false) public void testMethod(JSCallback callback, String args){ String result = "Hello " + args; callback.invoke(result); } }
- Define a class in Java
TestPlugin
for implementing Native plugin. -
@JSMethod(uiThread = false)
The annotation indicates that this method will be executed in a non-UI thread. -
JSCallback
is a callback interface used to return results in JavaScript. -
invoke
method is used to return the result to JavaScript.
Get the return value of the calling method
In order to get the return value of the calling method, we need to use the callback function in the Native code to return the value. The parameters in the callback function are the method's return value. In JavaScript code, we need to use the return value in the callback function to perform corresponding processing.
The following is an example of code that obtains the return value of a calling method:
- Use Promise in JavaScript to call Native methods:
export function testMethod(args){ return new Promise((resolve, reject) => { uni.invokeMethod("testPlugin", "testMethod", args, function(res){ resolve(res); }) }) }
- We use Promise in ES6 to asynchronously obtain the return value of the method. The
-
resolve
function represents a processing function that returns asynchronous results.
- Execute the method asynchronously in Native and return the result:
public class TestPlugin implements IModule { @JSMethod(uiThread = false) public void testMethod(JSCallback callback, String args){ String result = "Hello " + args; callback.invoke(result); } }
- In the callback function we use the
invoke
method to return result.
- Using return values in JavaScript:
testMethod('world') .then(res => { console.log(res); }) .catch(error => { console.error(error); });
- We use Promise’s
then
method after calling the method asynchronously Get the return value and process it in the callback function. - If an error occurs, we use Promise's
catch
method to capture the error and output it to the console.
Summary
Calling methods and getting return values is a common requirement in UniApp. Although UniApp allows us to use callback functions to obtain the results of calling methods, using Promise and async/await can make the code more concise and readable. In order to get the return value of the calling method, we can use Promise in JavaScript code to handle asynchronous results, and use callback functions in Native code to return the results.
The above is the detailed content of How to call a method in UniApp and get the return value. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


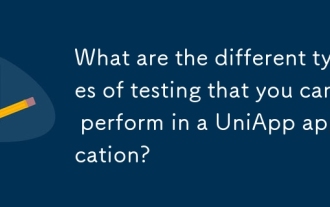
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
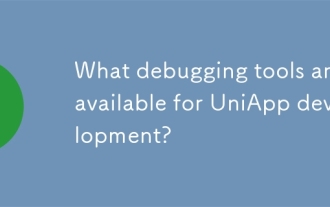
The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.
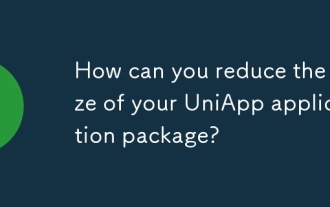
The article discusses strategies to reduce UniApp package size, focusing on code optimization, resource management, and techniques like code splitting and lazy loading.
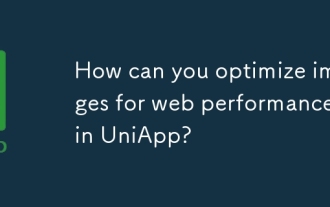
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.
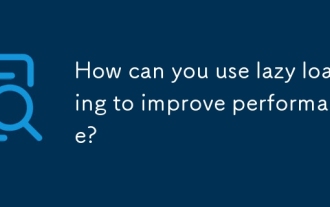
Lazy loading defers non-critical resources to improve site performance, reducing load times and data usage. Key practices include prioritizing critical content and using efficient APIs.
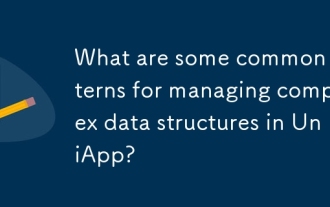
The article discusses managing complex data structures in UniApp, focusing on patterns like Singleton, Observer, Factory, and State, and strategies for handling data state changes using Vuex and Vue 3 Composition API.
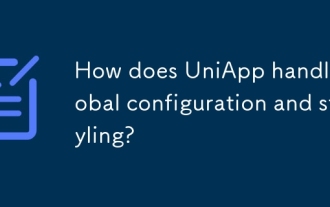
UniApp manages global configuration via manifest.json and styling through app.vue or app.scss, using uni.scss for variables and mixins. Best practices include using SCSS, modular styles, and responsive design.
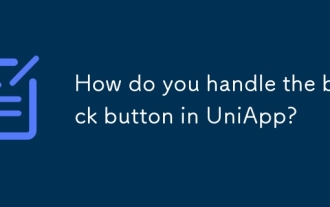
The article discusses handling the back button in UniApp using the onBackPress method, detailing best practices, customization, and platform-specific behaviors.
