How to change multiple database data in PHP
When developing web applications, it is often necessary to modify multiple data in the database. For PHP developers, being proficient in how to change multiple data can not only improve development efficiency, but also reduce the chance of errors. This article will introduce techniques on how to change data in multiple databases in PHP.
1. Use SQL statements to change multiple data
If you need to change multiple data in the database, the easiest way is to use SQL statements to change them all at once. Multiple data can be changed by setting the WHERE clause and the SET clause of the update statement.
For example, if you want to change the status of all records in a table to "processed", you can use the following SQL statement:
UPDATE table_name SET status='已处理' WHERE 1;
where table_name
is the value that needs to be changed The table name, status
is the name of the status field that needs to be changed.
This SQL statement uses the UPDATE
keyword to indicate that records in the table are to be updated, and the SET
clause to specify the fields that need to be changed and their corresponding new values. , use the WHERE
clause to specify the records that need to be changed.
It should be noted that 1
is used in the WHERE
clause of this SQL statement, which is a condition that is always true. This will match all records in the table and change their status to Processed.
2. Use foreach loop to change multiple data
In addition to using SQL statements, we can also use PHP’s foreach loop to change multiple data.
Suppose there is an array $data
, each element of which corresponds to the primary key of a database record and the field that needs to be changed and its corresponding new value. We can use the following code to batch change these records:
foreach ($data as $id => $values) { foreach ($values as $field => $value) { // 使用更新语句更新每个字段 $sql = "UPDATE table_name SET $field='$value' WHERE id='$id'"; // 执行更新语句 $result = mysqli_query($conn, $sql); // 错误处理 if (!$result) { die("Error: " . mysqli_error($conn)); } } }
In this code, we use a two-level foreach loop. The first level loop traverses each element in the $data
array. , the second layer loops through the fields that need to be changed and the corresponding new values in each element.
In the inner loop, we use an update statement to change the value of each field. It should be noted that $id
is used in the update statement to specify the primary key value of the record that needs to be changed.
3. Use batch update statements to change multiple data
In addition to the above two methods, you can also use batch update statements (Batch Update) to change multiple data. This method is suitable for situations where multiple fields need to be updated at the same time.
For example, if you want to change the status and scores of all records in a table to new values, you can use the following code:
// 生成批量更新语句 $sql = "UPDATE table_name SET status=?, score=? WHERE 1"; $stmt = mysqli_prepare($conn, $sql); // 绑定参数 $status = '已处理'; $score = 100; mysqli_stmt_bind_param($stmt, "ss", $status, $score); // 执行更新语句 mysqli_stmt_execute($stmt);
In this code, we first generate a batch update statement , which contains the fields and placeholders that need to be changed. Where ?
represents a placeholder, indicating that it needs to be replaced with the actual value when the update statement is executed.
Next, we use the mysqli_prepare
function to convert this SQL statement into a prepared statement. We then use the mysqli_stmt_bind_param
function to bind the parameters and replace the placeholders ?
with the actual values.
Finally, we use the mysqli_stmt_execute
function to execute this update statement.
It should be noted that when using batch update statements, if there are many fields to be changed, you need to ensure that their order in the SQL statement is consistent with the order when binding parameters, otherwise the update will fail.
Summary
This article introduces three methods on how to change multiple database data in PHP, including using SQL statements, using foreach loops, and using batch update statements. It is necessary to choose the corresponding method according to the actual situation, and pay attention to avoid data consistency problems caused by updates.
The above is the detailed content of How to change multiple database data in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
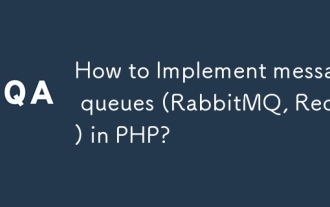
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
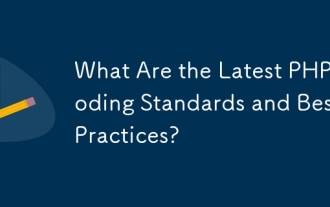
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
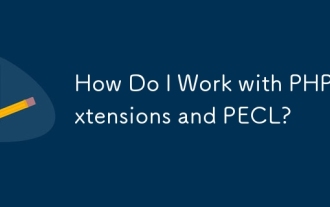
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
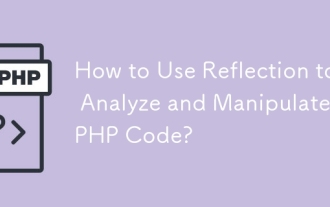
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
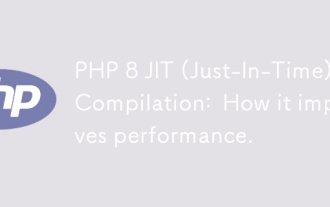
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
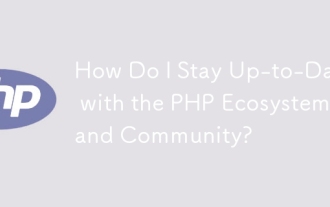
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
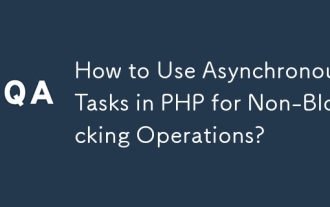
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
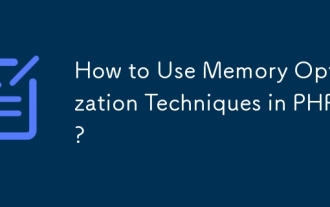
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
