How to implement keyword search for mysql data in php
With the development of the Internet, search engines have become one of the main ways for people to obtain information. In website development, implementing keyword search functions has gradually become a necessary requirement. This article will introduce how to use the PHP language to implement the function of keyword search for MySQL data.
1. Preparation work
Before we start writing code, we need to do some preparation work. First, we need to make sure we already have a mysql database with some data in it.
Next, create a test data table in the mysql database. We can use the following SQL statement to create a data table named "test":
CREATE TABLE test (
id
int(11) NOT NULL AUTO_INCREMENT,
title
varchar(255) NOT NULL,
content
text NOT NULL,
PRIMARY KEY (id
)
) ENGINE=InnoDB AUTO_INCREMENT =1 DEFAULT CHARSET=utf8mb4;
This data table contains three fields: id, title and content. Among them, the id field is an auto-incrementing field and is used to uniquely identify each piece of data. The title field represents the title of the data, and the content field represents the content of the data.
2. Implement the search function
Generally speaking, the keyword search function can consist of two steps: input keywords and query data. In php, we can implement the search function by getting the keywords entered by the user and matching them with the data in the database.
1. Get the keywords entered by the user
First, we need to add a form containing an input box and a submit button to the page to receive the keywords entered by the user. The code is as follows:
This form contains a text box and a submit button. When the user clicks the submit button, the form will submit the keywords entered by the user to a PHP file named "search.php" for processing.
In the file named "search.php", we need to first obtain the keywords entered by the user. The code is as follows:
$keyword = $_GET['keyword'];
This code uses PHP's built-in $_GET variable to obtain the file submitted to "search.php" in GET mode data in. In this example, the keyword entered by the user is stored in the $keyword variable.
2. Query data
After obtaining the keyword entered by the user, we need to query the data containing the keyword in the mysql database. The code is as follows:
//Connect to the database
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = " dbname";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $ conn->connect_error);
}
//Query data
$sql = "SELECT * FROM test WHERE title LIKE '%$keyword%' OR content LIKE '%$keyword%' ";
$result = $conn->query($sql);
// Output result
if ($result->num_rows > 0) {
while( $row = $result->fetch_assoc()) {
echo "标题:" . $row["title"] . "<br>内容:" . $row["content"] . "<br><br>";
}
} else {
echo "No relevant data found";
}
here In this code, we first connected to the mysql database and used PHP's built-in mysqli class to execute SQL query statements. The SQL statement uses the LIKE operator to match data containing $keyword in the title and content fields.
Next, we used PHP’s built-in query() method to execute the above SQL statement and save the result in the $result variable. If the data is queried, use a while loop to output each piece of queried data to the page in turn.
If no relevant data is found, the prompt message "No relevant data found" will be output.
3. Complete code
By combining the codes of the previous two steps, the search function can be completely realized. The complete php code is as follows:
$keyword = $_GET['keyword'];
$servername = "localhost";
$username = " username";
$password = "password";
$dbname = "dbname";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM test WHERE title LIKE '%$keyword%' OR content LIKE '%$keyword%'";
$result = $conn->query($sql);
if ($result->num_rows > ; 0) {
while($row = $result->fetch_assoc()) {
echo "标题:" . $row["title"] . "<br>内容:" . $row["content"] . "<br><br>";
}
} else {
echo "No relevant data found";
}
$conn->close();
?>
4. Summary
Through the introduction of this article, we have learned how to use the php language to implement the function of keyword search for mysql data. In the process of implementing this function, we need to master how to obtain user input data, how to connect to the database, how to execute SQL query statements and output the queried data to the page.
In actual website development, the implementation of search functions is often more complicated. We need to comprehensively use multiple languages and technologies such as php, mysql, javascript, etc. to complete this task. However, after mastering the basic search function implementation methods, we can better understand and participate in more complex website development.
The above is the detailed content of How to implement keyword search for mysql data in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


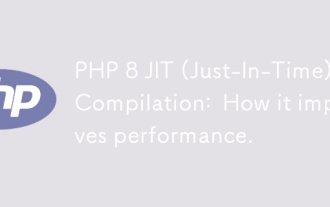
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
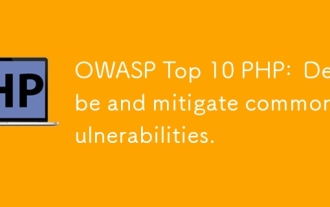
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
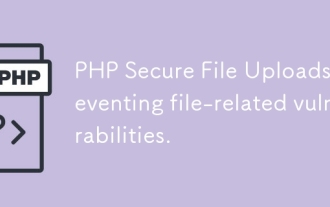
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
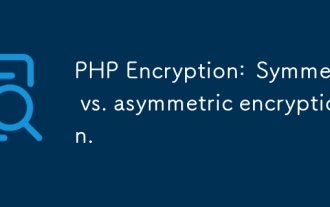
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
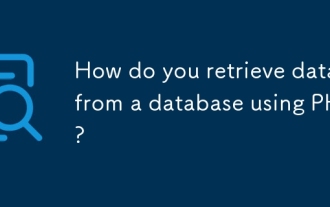
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
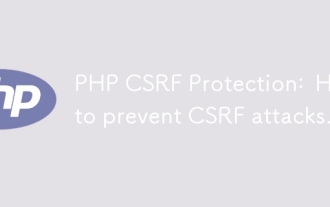
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
