How to batch change key names in php arrays
In PHP, array is a very practical data structure that can store and process multiple values. However, in actual development, we often need to batch modify the key names of arrays. In this case, we need to use the array-related functions to complete.
Below, I will introduce in detail how to batch modify array key names in PHP. Specifically, we will use the following function:
- array_combine(): Use the value of one array as the key of the new array, and the value of the other array as the value of the new array;
- array_keys(): Returns all the key names in the array;
- array_values(): Returns all the values in the array;
- array_map(): Apply the specified function to each array element, And returns a new array;
- array_walk(): Apply a user-defined function to each element in the array.
Now, we assume there is an array $arr, which contains several elements, each element has a numeric key name. We want to convert them into string key names, such as replacing "0" with "apple", replacing "1" with "banana", etc. So, what to do? Please look at the following code:
$arr = array('apple', 'banana', 'orange', 'peach', 'lemon'); $key_names = array('apple', 'banana', 'orange', 'peach', 'lemon'); $new_arr = array_combine($key_names, $arr); print_r($new_arr);
In this code, we first define an $arr array and a $key_names array, whose number of elements is the same. Next, we use the array_combine() function to use the value of the $arr array as the value of the new array and the value of the $key_names array as the key of the new array. Finally, we print out the contents of the new array $new_arr through the print_r() function, and we can see the result we want:
Array ( [apple] => apple [banana] => banana [orange] => orange [peach] => peach [lemon] => lemon )
In this way, we successfully converted the key name from a number to a string . But in some cases, our needs may be more complex. For example, we need to convert all key names from uppercase letters to lowercase letters. At this time, we can use the array_keys() and array_map() functions to achieve this. The code is as follows:
$arr = array( 'Apple' => 'red', 'Banana' => 'yellow', 'Orange' => 'orange', 'Peach' => 'pink', 'Lemon' => 'yellow' ); $lowercase_keys = array_map('strtolower', array_keys($arr)); $new_arr = array_combine($lowercase_keys, array_values($arr)); print_r($new_arr);
In this code, we first define an $arr array and capitalize the first letter of its key name. Next, we use the array_keys() function to return all the keys of the $arr array. We then use the array_map() function to apply the strtolower() function to each key name, converting them all to lowercase. Finally, we combine the new key name and the value of the $arr array using the array_combine() function to form a new array $new_arr. Use the print_r() function to print out the contents of the new array $new_arr, and you can see the modified result:
Array ( [apple] => red [banana] => yellow [orange] => orange [peach] => pink [lemon] => yellow )
In this way, we have successfully converted all key names into lowercase letters. It should be noted that when using the array_combine() function, make sure that the key names and the number of values of the new array are equal, otherwise an error will occur. Here, we use the array_values() function to get all the values of the $arr array to ensure that their number is the same as the number of $lowercase_keys array.
Of course, the above methods are only for demonstration. In actual use, you need to choose the appropriate functions and methods according to the specific situation.
In addition to the methods introduced above, there is also a more flexible way, which is to use the array_walk() function. This function can customize the callback function to operate on each element in the array, so it can meet more diverse needs.
Below, we implement a simple example to add the prefix "fruit_" to all key names in the array. The code is as follows:
$arr = array( 'apple' => 'red', 'banana' => 'yellow', 'orange' => 'orange', 'peach' => 'pink', 'lemon' => 'yellow' ); function add_prefix(&$value, $key, $prefix) { $value = $prefix . '_' . $key; } array_walk($arr, 'add_prefix', 'fruit'); print_r($arr);
In this code, we first define a $arr array, and defines an add_prefix() function. The add_prefix() function has three parameters, which are the value to be operated on, the key name of the current value and the specified prefix. Inside the function, we concatenate the key name and prefix of the current value to generate a new key name and assign it to the key name of the current value. Then, we use the array_walk() function to apply the add_prefix() function to each element in the $arr array, and finally print out the modified array:
Array ( [fruit_apple] => red [fruit_banana] => yellow [fruit_orange] => orange [fruit_peach] => pink [fruit_lemon] => yellow )
In this way, we have successfully implemented batch modification of array keys. name operation. In general, there are many built-in functions in PHP that can operate on arrays, and you can choose the most appropriate method according to your actual needs.
The above is the detailed content of How to batch change key names in php arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


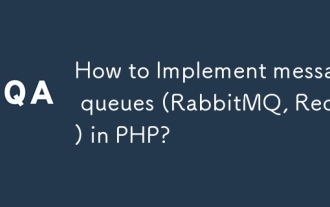
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
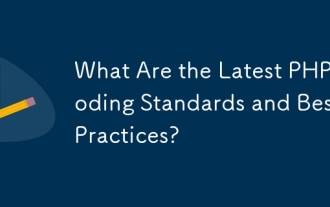
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
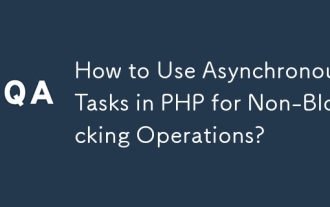
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
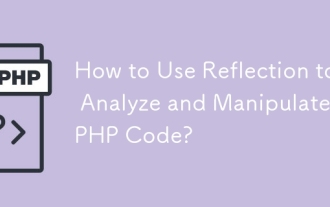
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
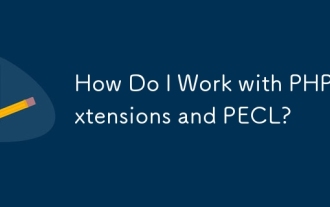
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
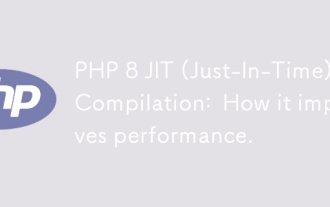
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
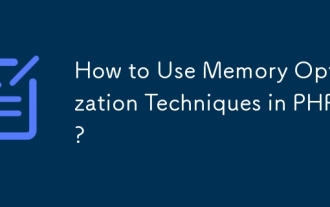
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
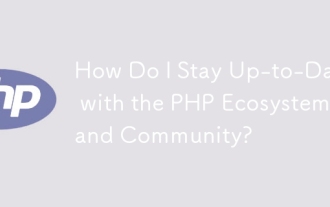
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
