What is the method for verifying ID number in php
In the process of website development, we often involve the verification of user information, including the verification of the user's ID number. In actual development, we usually use PHP to verify the legitimacy of the ID number to ensure that the information entered by the user is correct. Below we will introduce in detail the method of verifying the ID number in PHP.
1. The basic format of the ID number
Before verifying the ID number, we first need to understand the basic format of the ID number.
The 18-digit ID number consists of 17 digits and 1 digit check code. Among them, the first 6 digits represent the household registration area code; the 7th to 14th digits represent the date of birth; the 15th to 17th digits represent the sequence code; and the 18th digit represents the check code.
2. How to verify ID number in PHP
php has a ready-made function that can verify the legitimacy of ID number, that is, the regular expression function preg_match() in PHP. Let's take a look at the specific implementation method:
- First, introduce the regular expression pattern of the ID number.
$id_card_pattern = '/^\d{17}[0-9xX]$/';
- Call the preg_match() function for verification.
preg_match($id_card_pattern, $id_card_number);
Among them, $id_card_pattern is the regular expression pattern of the ID number, and $id_card_number is the ID number that needs to be verified.
- Judge the verification result and output the error message
if (! preg_match($id_card_pattern, $id_card_number)) {
echo "The format of the ID number is incorrect! ";
} else {
// Process the verification results and output success information
}
Through the above three steps, we can verify the ID number in PHP. At the same time, we can further improve the verification logic, such as obtaining date of birth, gender and other information based on the ID number to facilitate further operations.
3. Complete code example
$id_card_number = '510101198912123456';
$id_card_pattern = '/^\d{17}[0-9xX] $/';
if (! preg_match($id_card_pattern, $id_card_number)) {
echo "The format of the ID number is incorrect!";
} else {
// Get the identity Date of birth, gender and other information in the card number
$birthday = substr($id_card_number, 6, 8);
$gender = substr($id_card_number,16,1) % 2 == 0? 'Female' : 'Male';
echo "The format of the ID number is correct! Birthday: ".$birthday." Gender: ".$gender;
}
?>
Through the above code, we can complete the verification of the ID number and obtain the date of birth, gender and other information.
Summary:
The above is the method and code example of PHP verification of ID number. Using the regular expression function preg_match() can easily verify the ID number. In practical applications, we can improve the verification logic of ID numbers based on business needs to ensure that user information input is accurate. Of course, we can also use third-party tool libraries to complete ID number verification, such as the Validator class library that comes with the Laravel framework, making development more convenient.
The above is the detailed content of What is the method for verifying ID number in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


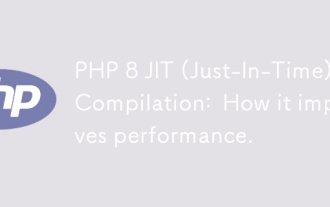
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
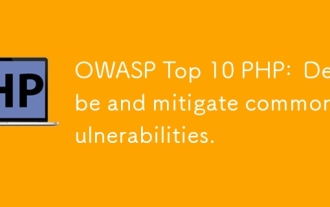
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
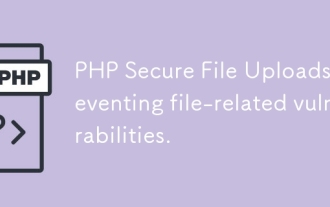
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
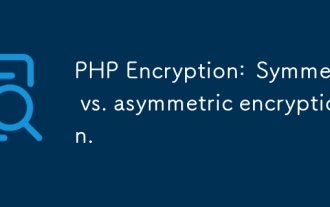
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
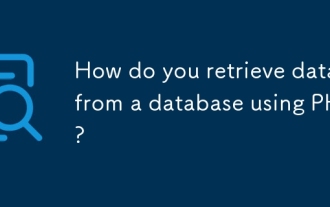
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
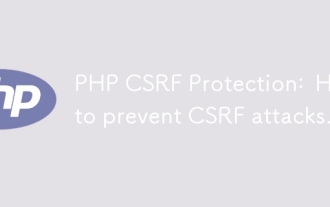
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.
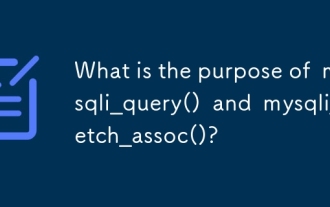
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
