How to use Laravel's fill data function
Laravel is a popular PHP framework that provides many useful functions and tools, one of the important features is filling data. Filling data refers to filling the data in the database table with some predefined values for testing and development. This article will introduce how to use Laravel's fill data function.
1. Preparation
Before using Laravel's data filling function, you need to create a database table and an Eloquent model. The following is a simple example:
php artisan make:model User -m
The above command will create a User
model and a database migration file xxxx_xx_xx_xxxxxx_create_users_table.php# in the
app directory. ##. We need to define the table structure in the migration file:
public function up() { Schema::create('users', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); }
php artisan migrate
users.
db:seed command for filling data. We can create a Seeder class in the
database/seeds directory, inherit the
Illuminate\Database\Seeder class, and implement the
run method.
UserSeeder.php:
use Illuminate\Database\Seeder; use App\User; class UserSeeder extends Seeder { public function run() { factory(User::class, 50)->create(); } }
User models, and store them into the database.
run method, we can use Laravel's query builder and Eloquent model to operate the database. In this example, we use the
factory function to create 50
User model instances and save them to the database.
database/seeds/DatabaseSeeder.php file. This class is a main seeder, which is used to call other seeder classes.
use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { public function run() { $this->call(UserSeeder::class); } }
UserSeeder class, we can execute the
db:seed command through the command line to fill in the data:
php artisan db:seed
Seeder classes and run their
run methods.
User model instances. Laravel's factory pattern can help us easily generate the data required for testing and development.
make and
create methods. The
make method is used to create a temporary model instance that is not saved to the database, and the
create method is used to create a model instance that is saved to the database.
Factory class to define, register and use factories. The use of the
Factory class is very simple. We only need to create a factory file in the
database/factories directory and define a model and its attributes through the
define method. Here is a simple example
UserFactory.php:
use Faker\Generator as Faker; use App\User; $factory->define(User::class, function (Faker $faker) { return [ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'password' => bcrypt('password'), ]; });
Faker class to generate random model data and simulate real users data. This factory defines three attributes:
name,
email, and
password.
use App\User; $user = factory(User::class)->create();
User model instance and save it to the database . We can also use the
make method to create a model instance that is not saved to the database:
use App\User; $user = factory(User::class)->make();
The above is the detailed content of How to use Laravel's fill data function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


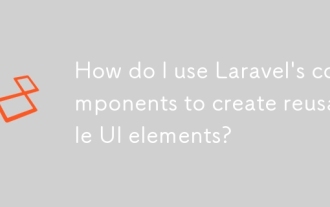
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
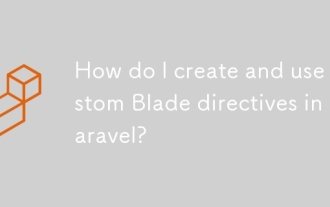
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
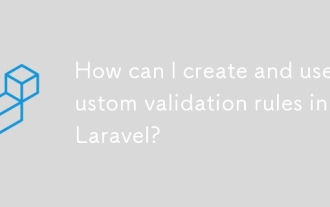
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
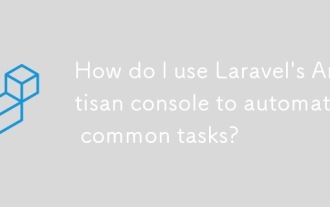
Laravel's Artisan console automates tasks like generating code, running migrations, and scheduling. Key commands include make:controller, migrate, and db:seed. Custom commands can be created for specific needs, enhancing workflow efficiency.Character
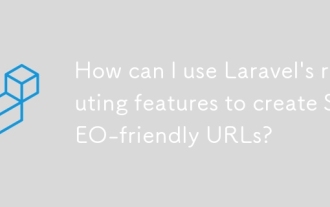
The article discusses using Laravel's routing to create SEO-friendly URLs, covering best practices, canonical URLs, and tools for SEO optimization.Word count: 159

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
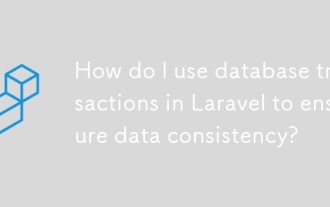
The article discusses using database transactions in Laravel to maintain data consistency, detailing methods with DB facade and Eloquent models, best practices, exception handling, and tools for monitoring and debugging transactions.
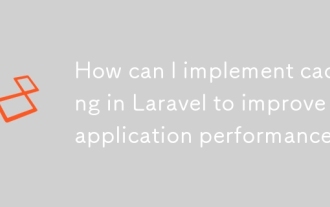
The article discusses implementing caching in Laravel to boost performance, covering configuration, using the Cache facade, cache tags, and atomic operations. It also outlines best practices for cache configuration and suggests types of data to cache
