How to generate random array in php
Random arrays are very common in PHP programming and are usually used in various scenarios such as testing, data simulation, custom scripts, and data manipulation. There are many ways to implement random arrays, but this article will focus on how PHP generates random arrays, which is a relatively simple and effective way.
1. Generate a random array using a loop method
You can use the rand function that comes with PHP to generate random numbers. According to the number of random numbers, the random numbers are generated in a loop and then stored in the array. . The specific implementation is as follows:
<?php function randArray($arrLen,$maxValue){ $arr = array(); for($i=0;$i<$arrLen;$i++){ $arr[$i]=mt_rand(0,$maxValue); } return $arr; } $arrLen = 5; $maxValue = 20; $arr = randArray($arrLen,$maxValue); var_dump($arr); ?>
The running results are as follows:
array(5) { [0]=> int(3) [1]=> int(10) [2]=> int(5) [3]=> int(14) [4]=> int(6) }
2. Use the array_fill function to generate a random array
PHP’s array_fill function is a function used to fill an array. Can be used to generate an array of specified length and value. Using its features, you can generate an array of specified length with one line of code.
<?php $arrLen = 5; $maxValue = 20; $arr = array_fill(0, $arrLen, $maxValue); var_dump($arr); ?>
The running results are as follows:
array(5) { [0]=> int(20) [1]=> int(20) [2]=> int(20) [3]=> int(20) [4]=> int(20) }
The arrays generated in the above examples are all fixed-value, but we can add changes in random numbers during generation to change the value of the random array. regularity and scope.
3. Add random factors
Through the above method, we generated arrays with specified lengths and values, but these arrays are a bit too regular and monotonous and do not meet our needs and requirements. .
Below we can add random factors to generate a random array. Different random numbers can be added to the array through the rules and range of random numbers to achieve the purpose of a random array.
<?php function randArray($arrLen,$minValue,$maxValue){ $arr = array(); for($i=0;$i<$arrLen;$i++){ $arr[$i]=mt_rand($minValue,$maxValue); } return $arr; } $arrLen = 5; $minValue = 0; $maxValue = 20; $arr = randArray($arrLen,$minValue,$maxValue); var_dump($arr); ?>
The running results are as follows:
array(5) { [0]=> int(1) [1]=> int(13) [2]=> int(9) [3]=> int(11) [4]=> int(19) }
The above method can generate random numbers and random arrays within the specified length and range, and realize the positioning and problem diagnosis of code errors and online problems. .
Summary:
There are many ways to generate random arrays in PHP. You can choose different methods according to your needs, including loop methods, array_fill function, and methods to generate high-quality random data. However, it must be used flexibly according to specific needs to achieve better results. It is recommended that when generating random arrays, attention should be paid to the addition of random factors to avoid unnecessary problems and harm in the project.
The above is the detailed content of How to generate random array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
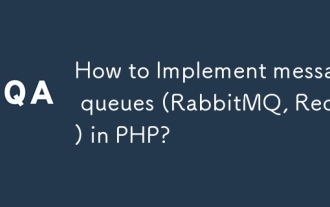
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
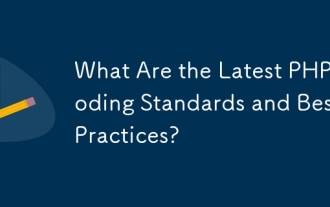
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
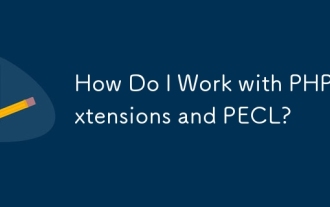
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
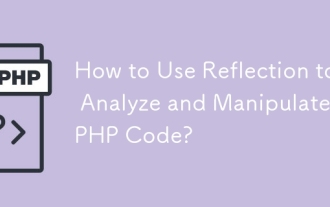
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
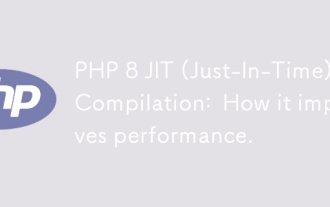
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
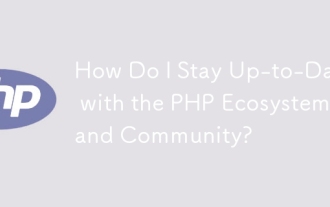
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
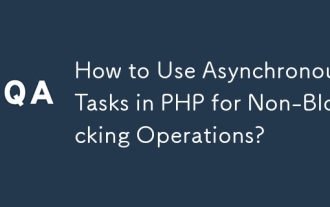
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
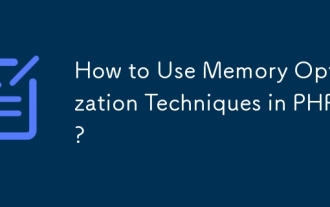
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
