How to use Python program to store images to MySQL
Environment
Python 3.7.4 pymysql 8.0.11 MySQL Community Server
Read pictures
Read pictures in binary format
with open("./test.jpg", "rb") as file: image = file.read()
Create a table to store pictures
The attributes of the picture fields are longblog
, that is, long binary large object
def create_image_table(self): sql = 'create table if not exists picture ( \ image longblob);' try: self.cursor.execute(sql) self.connection.commit() except pymysql.Error: print(pymysql.Error)
is stored in MySQL
Stores image data in binary format into MySQL
def insert_image(self, image): sql = "insert into picture(image) values(%s)" self.cursor.execute(sql, image) self.connection.commit()
Save the picture data obtained by MySQL query as picture
Write the picture in binary format
def get_image(self, path): sql = 'select * from picture' try: self.cursor.execute(sql) image = self.cursor.fetchone()[0] with open(path, "wb") as file: file.write(image) except pymysql.Error: print(pymysql.Error) except IOError: print(IOError)
Implementation code
import pymysql class Database(): ''' Description: database demo to store image in MySQL RDBMS Attributes: None ''' def __init__(self): self.connection = pymysql.connect(host='<host name>',user='<user name>',passwd='<password>',db='<database name>',charset='utf8') self.cursor = self.connection.cursor() ''' Description: create table to store images Args: None Return: None ''' def create_image_table(self): sql = 'create table if not exists picture ( \ image longblob);' try: self.cursor.execute(sql) self.connection.commit() except pymysql.Error: print(pymysql.Error) ''' Description: insert image into table Args: image: image to store Returns: None ''' def insert_image(self, image): sql = "insert into picture(image) values(%s)" self.cursor.execute(sql, image) self.connection.commit() ''' Description: get image from database Args: path: path to save image Returns: None ''' def get_image(self, path): sql = 'select * from picture' try: self.cursor.execute(sql) image = self.cursor.fetchone()[0] with open(path, "wb") as file: file.write(image) except pymysql.Error: print(pymysql.Error) except IOError: print(IOError) ''' Description: destruction method Args: None Returns: None ''' def __del__(self): self.connection.close() self.cursor.close() if __name__ == "__main__": database = Database() # read image from current directory with open("./test.jpg", "rb") as file: image = file.read() database.create_image_table() database.insert_image(image) database.get_image('./result.jpg')
Test result
The above is the detailed content of How to use Python program to store images to MySQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


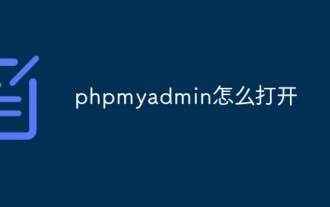
You can open phpMyAdmin through the following steps: 1. Log in to the website control panel; 2. Find and click the phpMyAdmin icon; 3. Enter MySQL credentials; 4. Click "Login".
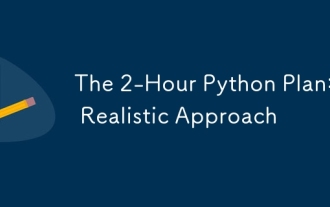
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
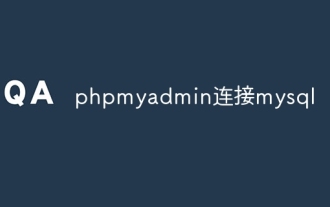
How to connect to MySQL using phpMyAdmin? The URL to access phpMyAdmin is usually http://localhost/phpmyadmin or http://[your server IP address]/phpmyadmin. Enter your MySQL username and password. Select the database you want to connect to. Click the "Connection" button to establish a connection.
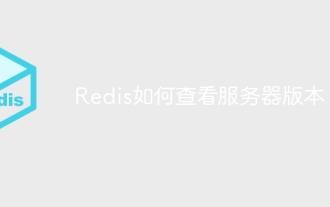
Question: How to view the Redis server version? Use the command line tool redis-cli --version to view the version of the connected server. Use the INFO server command to view the server's internal version and need to parse and return information. In a cluster environment, check the version consistency of each node and can be automatically checked using scripts. Use scripts to automate viewing versions, such as connecting with Python scripts and printing version information.
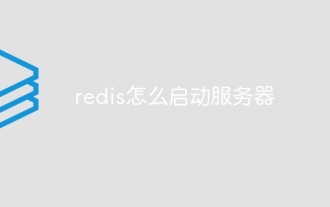
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
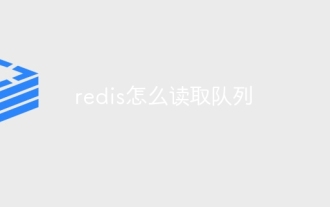
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
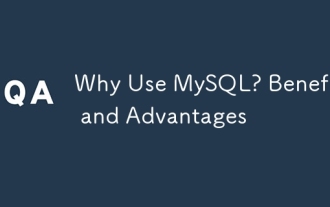
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
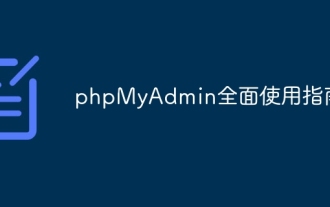
phpMyAdmin is not just a database management tool, it can give you a deep understanding of MySQL and improve programming skills. Core functions include CRUD and SQL query execution, and it is crucial to understand the principles of SQL statements. Advanced tips include exporting/importing data and permission management, requiring a deep security understanding. Potential issues include SQL injection, and the solution is parameterized queries and backups. Performance optimization involves SQL statement optimization and index usage. Best practices emphasize code specifications, security practices, and regular backups.
