How to use Node.js to simulate vehicle driving
With the continuous development of Internet of Things technology, intelligent transportation systems are also constantly improving. Among them, simulating vehicle driving is an important research direction. This article will introduce how to use Node.js to simulate vehicle driving and display the vehicle running status through a visual interface.
1. Introduction to Node.js
Node.js is a JavaScript running environment based on the Chrome V8 engine, which enables JavaScript to run on the server side. Node.js uses an event-driven, non-blocking I/O model, allowing it to efficiently handle large numbers of concurrent connections.
2. The need to simulate vehicle driving
In intelligent transportation systems, vehicle driving needs to be simulated to evaluate the impact of route planning, traffic flow and other factors on the transportation system. The specific requirements are as follows:
- Randomly generate the starting point and end point of the vehicle to simulate the vehicle operation process.
- Real-time display of vehicle position, speed, direction and other status information.
- You can control the speed and direction of the vehicle and manually intervene in the vehicle's driving process.
3. Use Node.js to implement vehicle driving simulation
- Install Node.js and related dependent libraries
Use Node.js to implement Vehicle driving simulation requires the installation of some related dependent libraries, such as the Express framework, Socket.IO library, etc. It can be installed through the npm package manager. The specific command is as follows:
npm install express --save npm install socket.io --save
- Randomly generate the starting and ending points of the vehicle
In Node.js, use Math.random() Functions can generate random numbers. We can use this function to generate the start and end points of the vehicle.
let startPoint = { x: Math.random() * 100, y: Math.random() * 100 }; let endPoint = { x: Math.random() * 100, y: Math.random() * 100 };
- Simulating the vehicle operation process
Use the setInterval() function to set a timer to execute a piece of code at a fixed time interval. We can simulate the movement of the vehicle in a timer.
let car = { position: startPoint, speed: 10, // 车速:每秒移动的距离 direction: { x: (endPoint.x - startPoint.x) / distance, y: (endPoint.y - startPoint.y) / distance } }; let timer = setInterval(() => { let distance = getDistance(car.position, endPoint); if (distance <= car.speed / 60) { // 到达终点 clearInterval(timer); } else { car.position.x += car.speed / 60 * car.direction.x; car.position.y += car.speed / 60 * car.direction.y; } io.emit('car update', car); // 发送车辆状态更新信息 }, 1000 / 60);
In the above code, the getDistance() function can calculate the distance between two points. At the same time, we also use the Socket.IO library to send vehicle status updates to all connected clients.
- Real-time display of vehicle status information
In the front-end page, use the Socket.IO library to receive vehicle status update information and store vehicle position, speed, direction and other information displayed in real time.
io.on('car update', (car) => { // 更新车辆图标的位置、旋转角度等信息 let icon = document.getElementById('car-icon'); icon.style.left = car.position.x + 'px'; icon.style.top = car.position.y + 'px'; icon.style.transform = 'rotate(' + getDirection(car.direction.x, car.direction.y) + 'deg)'; });
In the above code, the getDirection() function can calculate the rotation angle of the vehicle so that it always faces the driving direction.
- Manual intervention in the vehicle driving process
We can add control buttons to the front-end page to control the speed and direction of the vehicle. For example, you can set acceleration and deceleration buttons to increase or decrease the vehicle speed; you can also set left and right steering buttons to change the direction of the vehicle.
4. Summary
This article introduces how to use Node.js to simulate vehicle driving and display the vehicle running status through a visual interface. In practical applications, customized development can be carried out according to specific needs to achieve better simulation effects. At the same time, this method can also be applied to traffic optimization, driving training and other fields.
The above is the detailed content of How to use Node.js to simulate vehicle driving. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
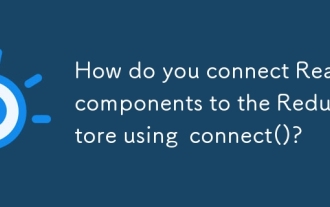
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
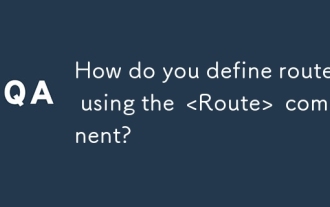
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
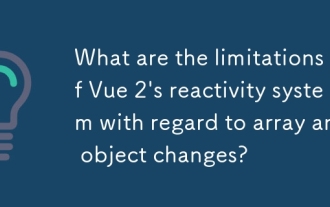
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
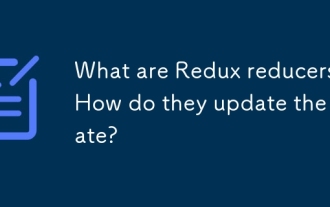
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
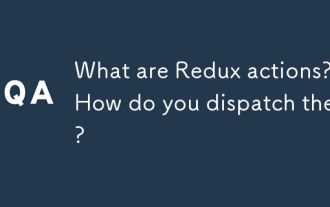
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
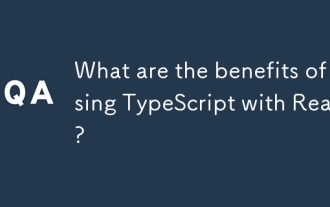
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
