What are the commonly used tool libraries in Java development?
Commonly used tool libraries in the Java development process
Apache Commons Class Library
Apache Commons is a very useful toolkit that provides common, ready-made code to solve various practical problems, without requiring us programmers to reinvent the wheel. For a detailed introduction to this class library, please visit the official website. The table below lists some of the tool kits. In our usual development process, we can choose the appropriate toolkit according to our own needs.
Components | Description | Latest Version | Released |
---|---|---|---|
BCEL | Byte Code Engineering Library - analyze, create, and manipulate Java class files | 6.3.1 | 2019/3/24 |
BeanUtils | Easy-to-use wrappers around the Java reflection and introspection APIs. | 1.9.3 | 2016/9/26 |
BSF | Bean Scripting Framework - interface to scripting languages, including JSR-223 | 3.1 | 2010/6/24 |
Chain | Chain of Responsibility pattern implemention. | 1.2 | 2008/6/2 |
CLI | Command Line arguments parser. | 1.4 | 2017/3/9 |
Codec | General encoding/decoding algorithms (for example phonetic, base64, URL). | 1.12 | 2019/2/16 |
Collections | Extends or augments the Java Collections Framework. | 4.3 | 2019/2/5 |
Compress | Defines an API for working with tar, zip and bzip2 files. | 1.18 | 2018/8/16 |
Configuration | Reading of configuration/preferences files in various formats. | 2.4 | 2018/10/29 |
Crypto | A cryptographic library optimized with AES-NI wrapping Openssl or JCE algorithm implementations. | 1.0.0 | 2016/7/22 |
CSV | Component for reading and writing comma separated value files. | 1.6 | 2018/9/25 |
Daemon | Alternative invocation mechanism for unix-daemon-like java code. | 1.0.15 | 2013/4/3 |
DBCP | Database connection pooling services. | 2.6.0 | 2019/2/19 |
DbUtils | JDBC helper library. | 1.7 | 2017/7/20 |
Digester | XML-to-Java-object mapping utility. | 3.2 | 2011/12/13 |
Library for sending e-mail from Java. | 1.5 | 2017/8/1 | |
Exec | API for dealing with external process execution and environment management in Java. | 1.3 | 2014/11/6 |
FileUpload | File upload capability for your servlets and web applications. | 1.4 | 2019/1/16 |
Functor | A functor is a function that can be manipulated as an object, or an object representing a single, generic function. | 1 | 2011-??-?? |
Geometry | Space and coordinates. | 1 | 2018-??-?? |
Imaging (previously called Sanselan) | A pure-Java image library. | 0.97-incubator | 2009/2/20 |
IO | Collection of I/O utilities. | 2.6 | 2017/10/15 |
JCI | Java Compiler Interface | 1.1 | 2013/10/14 |
JCS | Java Caching System | 2.2,1 | 2018/8/23 |
Jelly | XML based scripting and processing engine. | 1.0.1 | 2017/9/27 |
Jexl | Expression language which extends the Expression Language of the JSTL. | 3.1 | 2017/4/14 |
JXPath | Utilities for manipulating Java Beans using the XPath syntax. | 1.3 | 2008/8/14 |
Lang | Provides extra functionality for classes in java.lang. | 3.9 | 2019/4/15 |
Logging | Wrapper around a variety of logging API implementations. | 1.2 | 2014/7/11 |
Math | Lightweight, self-contained mathematics and statistics components. | 3.5 | 2015/4/17 |
Net | Collection of network utilities and protocol implementations. | 3.6 | 2017/2/15 |
Numbers | Number types (complex, quaternion, fraction) and utilities (arrays, combinatorics). | 1 | 2017-??-?? |
OGNL | An Object-Graph Navigation Language | 4 | 2013-??-?? |
Pool | Generic object pooling component. | 2.6.2 | 2019/4/11 |
Proxy | Library for creating dynamic proxies. | 1 | 2008/2/28 |
RDF | Common implementation of RDF 1.1 that could be implemented by systems on the JVM. | 0.3.0-incubating | 2016/11/15 |
RNG | Implementations of random numbers generators. | 1.2 | 2018/12/12 |
SCXML | An implementation of the State Chart XML specification aimed at creating and maintaining a Java SCXML engine.It is capable of executing a state machine defined using a SCXML document, and abstracts out the environment interfaces. | 0.9 | 2008/12/1 |
Statistics | Statistics. | 0.1 | ????-??-?? |
Text | Apache Commons Text is a library focused on algorithms working on strings. | 1.6 | 2018/10/16 |
Validator | Framework to define validators and validation rules in an xml file. | 1.6 | 2017/2/21 |
VFS | Virtual File System component for treating files, FTP, SMB, ZIP and such like as a single logical file system. | 2.3 | 2019/2/4 |
Weaver | Provides an easy way to enhance (weave) compiled bytecode. | 2 | 2018/9/7 |
In addition to the toolkits in the table above, the Apache Common project also includes the following toolkits, of which http-client is a commonly used one.
Cactus: Cactus is a simple test framework for unit testing server-side java code (Servlets, EJBs, Tag Libs, Filters, ...). The intent of Cactus is to lower the cost of writing tests for server-side code.
HiveMind: HiveMind is a services and configuration microkernel. HiveMind allows you to create your application using a service oriented architecture.
HttpClient 3.x: Framework for working with the client-side of the HTTP protocol.
Naming: The Naming subproject will contain common JNDI code along with various JNDI providers.
Guava class library
The Guava project contains several core libraries that are widely relied upon by Google's Java projects, such as: collections, caching, primitives support, concurrency libraries, common annotations, String processing, I/O, etc. The common function points in the Guava toolkit are listed below for easy reference and use when needed.
Basic utilities [Basic utilities]
Use and avoid null: Null is ambiguous, can cause confusing errors, and sometimes makes people uncomfortable. Many Guava utility classes use fail-fast to reject null values instead of blindly accepting them.
Preconditions: Make condition checking in methods easier.
Common Object methods: Simplify the implementation of Object methods, such as hashCode() and toString().
Sorting: Guava’s powerful “fluid style comparator”
Throwables: Simplifies the propagation and checking of exceptions and errors.
Collections[Collections]
Guava's extension to the JDK collection, which is the most mature and well-known part of Guava
Immutable Collections: Defensive programming and performance improvements with immutable collections.
New collection types: multisets, multimaps, tables, bidirectional maps, etc.
Powerful collection tool class: Provides collection tools not found in java.util.Collections.
Extend utility classes: Make it easier to implement and extend collection classes, such as creating Collection decorators or implementing iterators.
Caches[Caches]
Guava Cache: local cache implementation, supports multiple cache expiration strategies.
Functional style[Functional idioms]
Guava's functional support can significantly simplify your code, but use it with caution.
Concurrency[Concurrency]
Powerful yet simple abstractions make writing correct concurrent code easier
ListenableFuture: Future that triggers callback after completion
Service framework: Abstract services that can be turned on and off to help you maintain the state logic of the service
String processing [Strings]
Very useful string tools, including splitting, concatenating, filling and other operations
Primitive types[Primitives]
Extend native type (such as int, char) operations not provided by JDK, including unsigned forms of certain types
Interval[Ranges]
Interval API for comparable types, including continuous and discrete types
I/O
Simplify I/O, especially I/O stream and file operations, for Java5 and 6 versions
Hash[Hash]
Provides a more complex hash implementation than Object.hashCode() and provides an implementation of Bloom filter
Event Bus [EventBus]
Component communication in publish-subscribe mode, but components do not need to be explicitly registered with other components
Mathematical operations[Math]
Optimized, fully tested math tools
Reflection[Reflection]
Guava's Java reflection mechanism tool class
Common tool classes in Spring
FileCopyUtils;
WebUtil;
The above is the detailed content of What are the commonly used tool libraries in Java development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
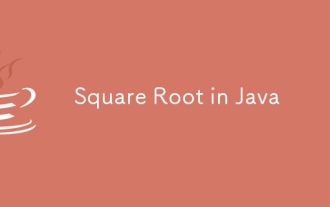
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
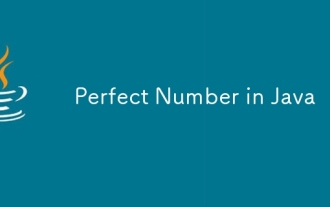
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
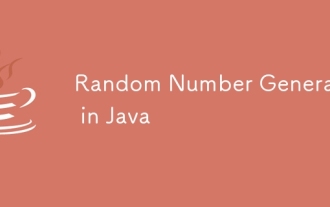
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
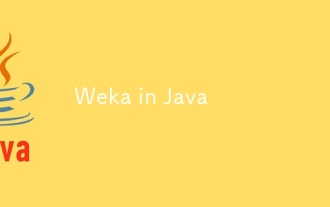
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
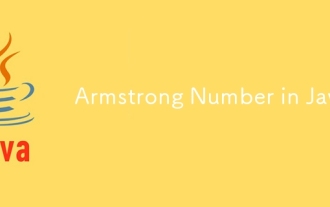
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
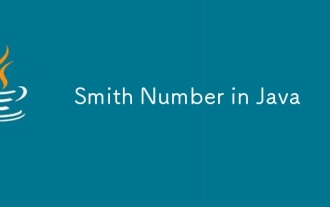
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
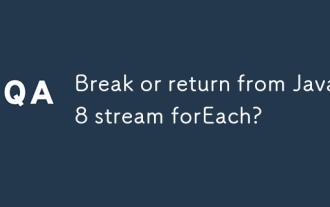
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
